Exploring TUI Libraries in Go
James Reed
Infrastructure Engineer · Leapcell
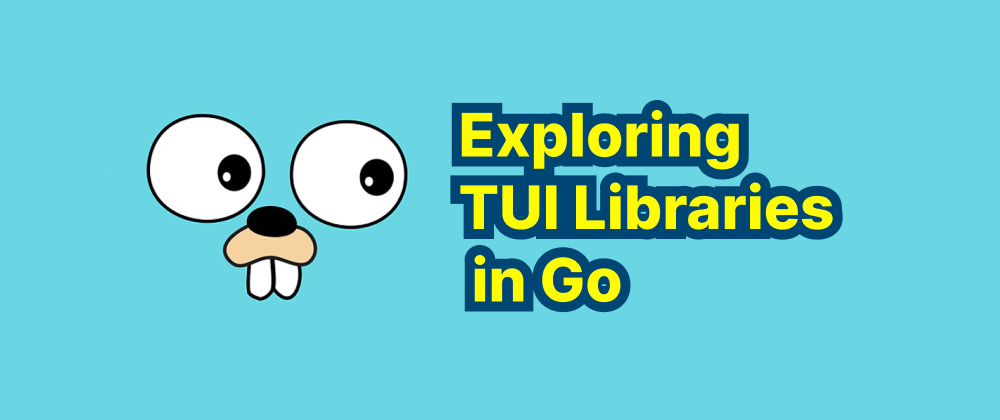
Key Takeaways
tview
offers a comprehensive set of components for building rich, cross-platform TUIs in Go.Bubble Tea
utilizes a functional programming approach, ideal for scalable and maintainable TUIs.- Additional libraries like
termbox-go
andpromptui
provide minimalist and specialized solutions for TUI development.
Text User Interfaces (TUIs) have seen a resurgence in popularity, offering a blend of nostalgia and efficiency for command-line applications. Golang, with its robust standard library and active community, provides several libraries to facilitate the creation of TUIs. This article delves into some of the prominent TUI libraries available for Go developers.
tview
tview
is a comprehensive library designed for building rich TUIs in Go. It offers a variety of components such as tables, forms, lists, and more, enabling developers to craft intricate terminal applications. Key features include:
-
Cross-Platform Compatibility:
tview
operates seamlessly across various Unix/Linux terminals and Windows command prompts. -
Rich Component Set: Provides numerous interactive components like tables, forms, and lists.
-
Event Handling: Simplifies layout management and event processing, including keyboard and mouse interactions.
-
Customizable Styling: Supports color and style customizations for personalized interfaces.
-
Developer-Friendly API: Designed with simplicity in mind, allowing for quick onboarding and reduced complexity in terminal UI development.
Example: Creating a simple box with a title using tview
:
package main import ( "github.com/rivo/tview" ) func main() { box := tview.NewBox().SetBorder(true).SetTitle("Hello, world!") if err := tview.NewApplication().SetRoot(box, true).Run(); err != nil { panic(err) } }
In this snippet, a box labeled "Hello, world!" is rendered in the terminal using tview
.
Bubble Tea
Bubble Tea
is another powerful TUI framework inspired by The Elm Architecture. It's well-suited for both simple and complex terminal applications, offering a functional approach to UI development. Notable aspects include:
-
Functional Design: Emphasizes a functional programming paradigm, promoting clear and maintainable code structures.
-
Component Library: Accompanied by
Bubbles
, a collection of common UI components like text inputs, viewports, and spinners. -
Styling Tools: Integrates with
Lip Gloss
, a library for styling terminal applications with borders, spacing, alignment, and color customization.
Example: Implementing a simple counter using Bubble Tea
:
package main import ( "fmt" "os" tea "github.com/charmbracelet/bubbletea" ) type model int func (m model) Init() tea.Cmd { return nil } func (m model) Update(msg tea.Msg) (tea.Model, tea.Cmd) { switch msg := msg.(type) { case tea.KeyMsg: switch msg.String() { case "ctrl+c", "q": return m, tea.Quit case "+": return m + 1, nil case "-": return m - 1, nil } } return m, nil } func (m model) View() string { return fmt.Sprintf("Count: %d\nPress + to increment, - to decrement, q to quit.\n", m) } func main() { p := tea.NewProgram(model(0)) if err := p.Start(); err != nil { fmt.Fprintf(os.Stderr, "Error running program: %v\n", err) os.Exit(1) } }
This example demonstrates a simple counter that increments or decrements based on user input.
Additional Libraries
Beyond tview
and Bubble Tea
, the Go ecosystem offers other libraries for TUI development:
-
termbox-go: A minimalistic library providing basic terminal control functionalities, suitable for developers seeking fine-grained control over terminal rendering.
-
go-tui: Offers a higher-level abstraction over
termbox-go
, simplifying the creation of text-based user interfaces. -
promptui: Specializes in interactive prompts and command-line inputs, ideal for CLI applications requiring user interaction.
Conclusion
Golang's versatility extends into the realm of text-based user interfaces, with libraries like tview
and Bubble Tea
providing robust tools for developers. Whether you're building simple command-line tools or complex terminal applications, these libraries offer the flexibility and functionality needed to create effective TUIs.
FAQs
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ