Understanding Constructors in Go
Grace Collins
Solutions Engineer · Leapcell
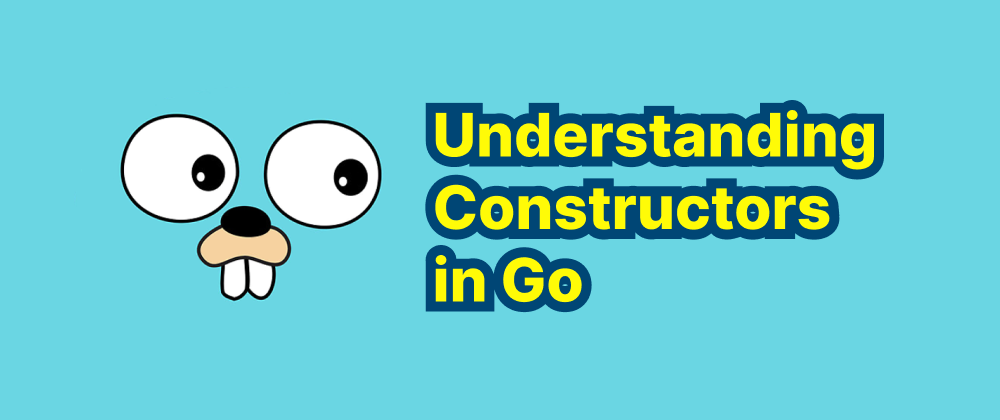
Key Takeaways
- Go uses factory functions instead of traditional constructors.
- Factory functions allow for initialization, validation, and setting defaults.
- Factory functions enhance code encapsulation and flexibility.
In Go, the concept of a constructor, as found in other programming languages, does not exist in the same form. However, Go developers commonly use factory functions to achieve similar functionality. This article explores how to implement and utilize these constructor-like patterns in Go.
What is a Constructor?
A constructor is a special function or method in object-oriented languages that initializes a newly created object. It often sets initial values for the object's attributes and performs any setup or validation required before the object is used.
Constructors in Go: Factory Functions
Go does not have classes or traditional constructors. Instead, it uses structs to define data structures and functions to operate on them. To initialize a struct with specific values or to enforce certain invariants, Go developers often use factory functions. These are regular functions that return an instance of the struct.
Example: Basic Factory Function
Consider a simple struct representing a User
:
type User struct { Name string Email string }
A factory function to create a new User
might look like this:
func NewUser(name, email string) *User { return &User{ Name: name, Email: email, } }
Here, NewUser
initializes a User
struct with the provided name and email, returning a pointer to the new User
.
Example: Factory Function with Validation
Factory functions can also include validation logic to ensure that the created struct is in a valid state:
func NewUser(name, email string) (*User, error) { if name == "" { return nil, errors.New("name cannot be empty") } if !isValidEmail(email) { return nil, errors.New("invalid email address") } return &User{ Name: name, Email: email, }, nil }
In this example, NewUser
checks that the name is not empty and that the email is valid before creating the User
. If any validation fails, it returns an error.
Benefits of Using Factory Functions
- Encapsulation: Factory functions can hide the details of struct initialization, allowing changes to the struct's internal representation without affecting code that uses it.
- Validation: They provide a centralized place to enforce invariants and validate input before creating an instance.
- Default Values: Factory functions can set default values for fields that are not provided by the caller.
Conclusion
While Go does not have traditional constructors, factory functions serve a similar purpose by providing controlled and validated creation of structs. This pattern leverages Go's strengths and idioms, offering a flexible and effective way to initialize complex data structures.
FAQs
Go emphasizes simplicity and uses factory functions for object initialization.
Include validation logic within the factory function to ensure data integrity.
They provide encapsulation, input validation, and the ability to set default values.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ