Deep Dive into Cryptographic Algorithms with Python
James Reed
Infrastructure Engineer ยท Leapcell
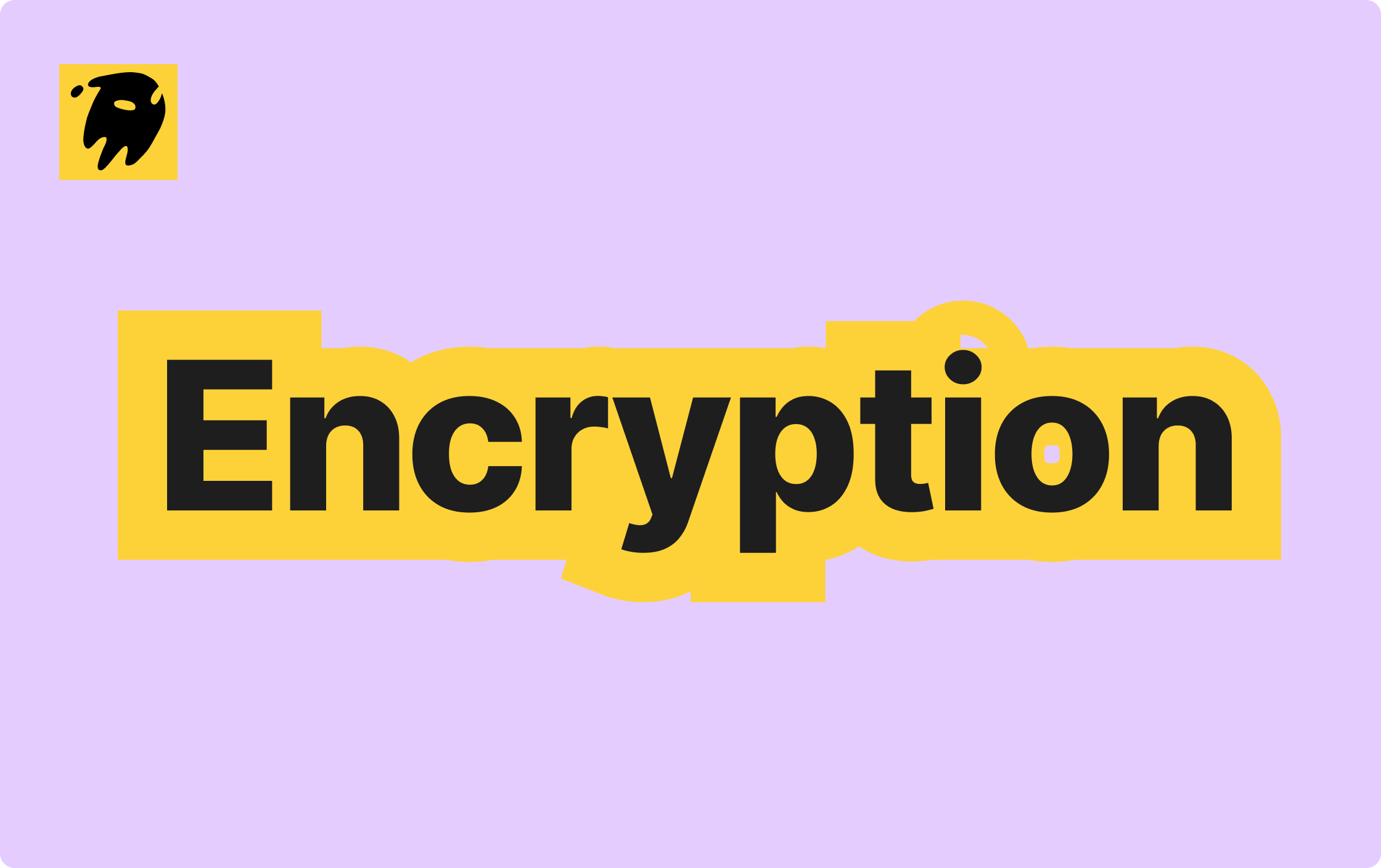
Analysis of Commonly Used Encryption Methods in Internet Development
In the current era of vigorous development of Internet technology, data is like the "blood" of the digital world. Its security is directly related to user rights and interests, corporate reputation, and even the stability of the entire network ecosystem. From users' account passwords to financial transaction data, each piece of information may become the target of hackers. Therefore, as a "shield" to protect data security, encryption technology plays a crucial and pivotal role in Internet development. This article will deeply analyze several commonly used encryption methods, including symmetric encryption, asymmetric encryption, hash functions, and salting technology. Through the elaboration of principles, Python code examples, and analysis of implementation scenarios, it aims to help developers comprehensively master these key technologies.
I. Symmetric Encryption
As the name implies, symmetric encryption is an encryption method in which the same key is used for both the encryption and decryption processes. Its core advantage lies in the extremely fast speed of encryption and decryption, which enables it to efficiently process a large amount of data. This makes it widely applied in scenarios of data transmission and storage. Common symmetric encryption algorithms include AES (Advanced Encryption Standard), DES (Data Encryption Standard), etc. Among them, AES, with its higher security and performance, has become the most widely used symmetric encryption algorithm currently.
Algorithm Principle
AES adopts a block encryption mode. It divides the plaintext into multiple data blocks according to a fixed length (usually 128 bits), and then uses the key to encrypt each data block to generate ciphertext. The key length can be selected as 128 bits, 192 bits, or 256 bits. The longer the key length, the more difficult it is to crack, and the higher the security. For example, when the key length is 128 bits, the theoretical number of possible key combinations is as high as 2 to the power of 128, making brute-force cracking almost impossible.
Python Implementation
In Python, the pycryptodome
library can be used to implement AES encryption and decryption. The following is the specific code:
from Crypto.Cipher import AES from Crypto.Util.Padding import pad, unpad import os # AES encryption function def aes_encrypt(plain_text, key): # Create an AES encryption object using the CBC (Cipher Block Chaining) mode, which can enhance the security of encryption cipher = AES.new(key, AES.MODE_CBC) # Initialization Vector (IV), used to increase the randomness of encryption iv = cipher.iv # Pad the plaintext to make its length an integer multiple of AES.block_size padded_plain_text = pad(plain_text.encode(), AES.block_size) # Encrypt the padded plaintext cipher_text = cipher.encrypt(padded_plain_text) # Return the initialization vector and the encrypted ciphertext, because the initialization vector is required for decryption return iv + cipher_text # AES decryption function def aes_decrypt(cipher_text, key): # Extract the initialization vector iv = cipher_text[:AES.block_size] # Create an AES decryption object using the extracted initialization vector and the key cipher = AES.new(key, AES.MODE_CBC, iv) # Decrypt the ciphertext and remove the padding part plain_text = unpad(cipher.decrypt(cipher_text[AES.block_size:]), AES.block_size) # Convert the decrypted byte data into a string and return it return plain_text.decode() # Generate a 128-bit key (16 bytes) key = os.urandom(16) plain_text = "Hello, World!" cipher_text = aes_encrypt(plain_text, key) decrypted_text = aes_decrypt(cipher_text, key) print("Before AES encryption:", plain_text) print("After AES encryption:", cipher_text.hex()) print("After AES decryption:", decrypted_text)
Implementation Scenarios
During the data transmission process between the client and the server, symmetric encryption is often used to protect users' sensitive information. For example, the account passwords entered by users during login, the bank card numbers during online payments, etc., can all be encrypted and transmitted through symmetric encryption algorithms to ensure that the information is not stolen during network transmission. In addition, sensitive data stored in the database, such as users' ID numbers, medical records, etc., can also be encrypted and stored using symmetric encryption to further enhance data security.
II. Asymmetric Encryption
Asymmetric encryption is different from symmetric encryption. It uses a pair of keys: a public key and a private key. The public key can be made public and is used for encrypting data; while the private key should be properly kept by the user and is used for decrypting data. This encryption method solves the problem of key distribution in symmetric encryption. Common asymmetric encryption algorithms include RSA, ECC (Elliptic Curve Cryptography Algorithm), etc., among which RSA is the most widely used.
Algorithm Principle
The RSA algorithm is based on the difficulty of factoring large numbers. In simple terms, it multiplies two large prime numbers to obtain a composite number, and then uses the composite number and one of the prime numbers as the public key, and the other prime number as the private key. When encrypting, the public key is used to encrypt the plaintext; when decrypting, the corresponding private key is used to decrypt the ciphertext. Since the process of factoring a large composite number into two prime numbers is extremely difficult, this ensures the security of the RSA algorithm.
Python Implementation
The pycryptodome
library can be used to implement RSA encryption and decryption. The code is as follows:
from Crypto.PublicKey import RSA from Crypto.Cipher import PKCS1_OAEP import os # Function to generate RSA key pair def generate_rsa_keys(): # Generate a 2048-bit RSA key pair. The longer the key length, the higher the security key = RSA.generate(2048) # Export the private key private_key = key.export_key() # Export the public key public_key = key.publickey().export_key() # Save the private key to a file with open("private.pem", "wb") as f: f.write(private_key) # Save the public key to a file with open("public.pem", "wb") as f: f.write(public_key) # RSA encryption function def rsa_encrypt(plain_text, public_key_path): # Read the public key file with open(public_key_path, "rb") as f: public_key = RSA.import_key(f.read()) # Create a PKCS1_OAEP encryption object using the public key cipher = PKCS1_OAEP.new(public_key) # Encrypt the plaintext cipher_text = cipher.encrypt(plain_text.encode()) return cipher_text # RSA decryption function def rsa_decrypt(cipher_text, private_key_path): # Read the private key file with open(private_key_path, "rb") as f: private_key = RSA.import_key(f.read()) # Create a PKCS1_OAEP decryption object using the private key cipher = PKCS1_OAEP.new(private_key) # Decrypt the ciphertext plain_text = cipher.decrypt(cipher_text) return plain_text.decode() # Generate RSA key pair when running for the first time # generate_rsa_keys() public_key_path = "public.pem" private_key_path = "private.pem" plain_text = "Hello, RSA!" cipher_text = rsa_encrypt(plain_text, public_key_path) decrypted_text = rsa_decrypt(cipher_text, private_key_path) print("Before RSA encryption:", plain_text) print("After RSA encryption:", cipher_text.hex()) print("After RSA decryption:", decrypted_text)
Implementation Scenarios
Asymmetric encryption is often used in scenarios of key exchange and digital signatures. In key exchange, the client and the server can safely exchange the keys for symmetric encryption through an asymmetric encryption algorithm, and then use the symmetric encryption algorithm to encrypt and transmit a large amount of data, achieving a balance between security and efficiency. In terms of digital signatures, for example, when releasing software, developers use their own private keys to sign the software, and users use the developers' public keys to verify the signatures, so as to ensure that the software has not been tampered with during the transmission process.
III. Hash Functions
A hash function can convert input data of any length into a fixed-length hash value. It has the property of one-way irreversibility, that is, it is impossible to restore the original data from the hash value. At the same time, it is very difficult for different input data to obtain the same hash value (collision resistance). Common hash functions include MD5 (Message Digest Algorithm 5), SHA - 1 (Secure Hash Algorithm 1), SHA - 256, etc. Although MD5 was once widely used, due to the threat to its collision resistance, it is gradually being replaced by SHA - 256 and others in scenarios with high security requirements.
MD5 Algorithm Principle
MD5 divides the input data into blocks of 512 bits. Through a series of complex hash operations, it finally generates a 128-bit hash value. However, with the improvement of computing power, methods have been found to generate the same MD5 hash value for two different inputs, which greatly reduces the security of MD5.
Python Implementation
The built-in hashlib
library in Python can easily implement MD5 hash calculation. The sample code is as follows:
import hashlib # MD5 hash calculation function def md5_hash(plain_text): # Create an MD5 hash object md5 = hashlib.md5() # Update the hash object with the plaintext data for which the hash value needs to be calculated md5.update(plain_text.encode()) # Return the hash value in hexadecimal form return md5.hexdigest() plain_text = "Hello, MD5!" md5_hash_value = md5_hash(plain_text) print("MD5 hash value:", md5_hash_value)
Implementation Scenarios
Hash functions are often used for data integrity verification. During the file transfer process, the sender calculates the hash value of the file and sends it to the receiver. After receiving the file, the receiver recalculates the hash value. By comparing the two hash values, it can be determined whether the file has been tampered with during the transfer process. In addition, in the password storage scenario, the traditional practice is to convert the user's password into a hash value through a hash function and store it in the database to avoid the leakage of plaintext passwords. However, this method has certain risks and needs to be combined with salting technology to further enhance security.
IV. Salting Technology
In the field of password storage, if only a hash function is used to process passwords, attackers may use tools such as rainbow tables to crack the hash values and obtain users' passwords. To solve this problem, salting technology came into being. Salting technology is to add a randomly generated salt value (Salt) to the password, mix the salt value with the password and then perform hash processing, and finally store the salt value and the hash value together.
Technical Principle
The salt value is a randomly generated string, and the salt value generated each time is different. Even if two users use the same password, due to different salt values, the generated hash values will also be different. This greatly increases the difficulty for attackers to crack passwords through rainbow tables and effectively improves the security of password storage.
Python Implementation
The code for implementing salted hashing and password verification using the hashlib
library is as follows:
import hashlib import os # Salted hashing function def salted_hash(password, salt=None): if salt is None: # Generate a 16-byte salt value (32-bit hexadecimal string) salt = os.urandom(16).hex() # Concatenate the password and the salt value combined = password + salt # Use the SHA - 256 hash algorithm to calculate the hash value of the concatenated string hashed = hashlib.sha256(combined.encode()).hexdigest() return salt, hashed # Password verification function def verify_password(password, salt, hashed_password): # Concatenate the input password and the salt value obtained from the database combined = password + salt # Calculate the hash value of the concatenated string new_hash = hashlib.sha256(combined.encode()).hexdigest() # Compare whether the calculated hash value is the same as the hash value stored in the database return new_hash == hashed_password # Process the password during registration password = "user_password" salt, hashed_password = salted_hash(password) print("Salt value:", salt) print("Salted hash value:", hashed_password) # Verify the password during login input_password = "user_password" is_valid = verify_password(input_password, salt, hashed_password) print("Password verification result:", is_valid)
Implementation Scenarios
Salting technology is mainly applied to the user password storage scenario. When a user registers, the system generates a random salt value, mixes it with the password entered by the user and then performs hash processing, and stores the salt value and the hash value in the database. When the user logs in, the system retrieves the salt value from the database, mixes it with the password entered by the user and then performs hash processing again. By comparing the newly generated hash value with the hash value in the database, it verifies the correctness of the password and effectively resists brute-force cracking and rainbow table attacks.
V. Selection and Application of Encryption Methods
In actual Internet development, choosing the appropriate encryption method requires a comprehensive consideration of the application scenario and security requirements:
- Data Transmission Scenario: For the transmission of a large amount of data, symmetric encryption algorithms (such as AES) should be preferentially used for data encryption, and at the same time, asymmetric encryption algorithms (such as RSA) should be used to complete the key exchange to achieve a balance between security and efficiency.
- Password Storage Scenario: Adopt salting technology combined with a secure hash function (such as SHA - 256) to process passwords, and avoid using hash functions with lower security such as MD5 to prevent password leakage.
- Data Integrity Verification Scenario: Use hash functions such as SHA - 256 to hash the data and generate hash values for verifying whether the data has been tampered with.
- Digital Signature Scenario: Use asymmetric encryption algorithms (such as RSA) for digital signatures to ensure the integrity and authenticity of the data.
Encryption technology in Internet development is a key defense line for ensuring data security. Developers should reasonably select encryption algorithms according to different scenarios, and continuously optimize the encryption scheme by combining technologies such as salting. At the same time, they should pay attention to the latest research achievements in encryption technology to deal with the increasingly complex network security threats and safeguard the security and stability of the digital world.
Leapcell: The Best of Serverless Web Hosting
Finally, I would like to recommend a platform that is most suitable for deploying Web services, Leapcell
๐ Build with Your Favorite Language
Develop effortlessly in JavaScript, Python, Go, or Rust.
๐ Deploy Unlimited Projects for Free
Only pay for what you useโno requests, no charges.
โก Pay-as-You-Go, No Hidden Costs
No idle fees, just seamless scalability.
๐ Explore Our Documentation
๐น Follow us on Twitter: @LeapcellHQ