A Guide to Golang Cache Libraries
Grace Collins
Solutions Engineer · Leapcell
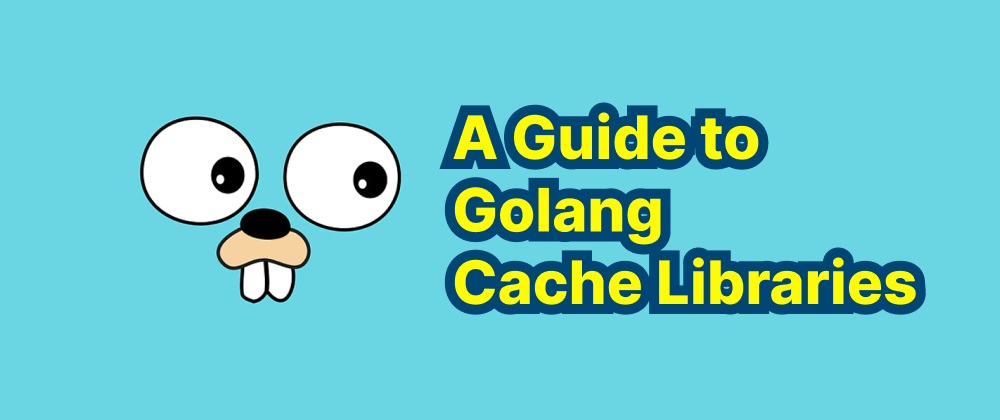
Key Takeaways
- Different Golang cache libraries serve different needs – Some focus on simplicity (
go-cache
), while others prioritize high performance (BigCache
,FreeCache
,Ristretto
). - Performance and scalability vary – High-throughput applications benefit from
BigCache
orRistretto
, while smaller projects may prefergo-cache
. - Expiration support differs –
go-cache
andFreeCache
have built-in expiration, butBigCache
requires manual handling.
Caching is an essential technique in software development that helps improve application performance and reduce redundant computations. In Golang, several caching libraries can be used depending on the application's requirements. This article explores some of the most popular Golang cache libraries and their use cases.
go-cache
Overview
go-cache
is an in-memory key-value store similar to Memcached but designed for Golang applications. It is simple, thread-safe, and supports item expiration.
Installation
go get github.com/patrickmn/go-cache
Usage Example
package main import ( "fmt" "time" "github.com/patrickmn/go-cache" ) func main() { c := cache.New(5*time.Minute, 10*time.Minute) // Set a cache item with default expiration c.Set("foo", "bar", cache.DefaultExpiration) // Retrieve the item foo, found := c.Get("foo") if found { fmt.Println("Found value:", foo) } else { fmt.Println("Item not found") } }
Pros and Cons
✅ Pros:
- Easy to use and lightweight.
- Supports automatic expiration and cleanup.
- Thread-safe.
❌ Cons:
- Limited to a single instance (not distributed).
- Might not scale well for high-concurrency applications.
BigCache
Overview
BigCache
is a high-performance in-memory cache designed to handle large amounts of data efficiently. It minimizes garbage collection overhead by using custom memory management.
Installation
go get github.com/allegro/bigcache
Usage Example
package main import ( "fmt" "log" "time" "github.com/allegro/bigcache" ) func main() { cache, err := bigcache.NewBigCache(bigcache.DefaultConfig(10 * time.Minute)) if err != nil { log.Fatal(err) } cache.Set("foo", []byte("bar")) entry, err := cache.Get("foo") if err != nil { fmt.Println("Item not found") } else { fmt.Println("Found value:", string(entry)) } }
Pros and Cons
✅ Pros:
- Optimized for high-throughput applications.
- Does not use
map[string]interface{}
internally, reducing garbage collection pressure. - Scales well with large datasets.
❌ Cons:
- Does not support built-in expiration for individual items.
- More complex than
go-cache
.
FreeCache
Overview
FreeCache
is another high-performance Golang cache library that provides efficient memory allocation and supports item expiration.
Installation
go get github.com/coocood/freecache
Usage Example
package main import ( "fmt" "github.com/coocood/freecache" ) func main() { cacheSize := 100 * 1024 * 1024 // 100MB cache := freecache.NewCache(cacheSize) cache.Set([]byte("foo"), []byte("bar"), 60) entry, err := cache.Get([]byte("foo")) if err != nil { fmt.Println("Item not found") } else { fmt.Println("Found value:", string(entry)) } }
Pros and Cons
✅ Pros:
- Supports item expiration.
- Optimized to reduce garbage collection pressure.
❌ Cons:
- Uses byte slices as keys, which might be less convenient than string keys.
Ristretto
Overview
Ristretto
is a high-performance Golang caching library developed by Dgraph. It uses advanced cache admission policies and efficient memory management.
Installation
go get github.com/dgraph-io/ristretto
Usage Example
package main import ( "fmt" "time" "github.com/dgraph-io/ristretto" ) func main() { cache, err := ristretto.NewCache(&ristretto.Config{ NumCounters: 1e7, // Number of keys to track. MaxCost: 1 << 30, // Maximum memory usage. BufferItems: 64, }) if err != nil { panic(err) } cache.Set("foo", "bar", 1) // Allow cache write to complete time.Sleep(10 * time.Millisecond) value, found := cache.Get("foo") if found { fmt.Println("Found value:", value) } else { fmt.Println("Item not found") } }
Pros and Cons
✅ Pros:
- Uses TinyLFU-based admission policy for better cache hit rates.
- Efficient and scales well with large datasets.
❌ Cons:
- More complex configuration than simpler caches like
go-cache
.
Choosing the Right Cache Library
When selecting a Golang caching library, consider the following factors:
- Performance: For high-throughput applications,
BigCache
,FreeCache
, orRistretto
are better choices. - Expiration Management: If you need item expiration,
go-cache
andFreeCache
provide built-in support. - Concurrency:
BigCache
andRistretto
are optimized for concurrent access. - Ease of Use:
go-cache
is the simplest and best suited for small-scale applications.
Feature | go-cache | BigCache | FreeCache | Ristretto |
---|---|---|---|---|
Expiration Support | ✅ | ❌ | ✅ | ✅ |
High Concurrency | ❌ | ✅ | ✅ | ✅ |
Garbage Collection Optimized | ❌ | ✅ | ✅ | ✅ |
Admission Policy | ❌ | ❌ | ❌ | ✅ |
Use Case | Simple apps | Large-scale caching | Expiring cache | High-performance |
Conclusion
Golang provides a variety of caching libraries, each tailored to different use cases. Whether you need a simple in-memory store like go-cache
, a high-performance solution like BigCache
or FreeCache
, or an advanced caching system like Ristretto
, there is a library that suits your needs. Carefully consider your application's requirements before choosing a caching solution.
FAQs
BigCache
and Ristretto
are optimized for high-concurrency workloads.
No, go-cache
is an in-memory cache and does not support distributed caching.
Ristretto
uses TinyLFU admission policies, improving cache hit rates and memory efficiency.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ