Understanding Type Conversion in Go
James Reed
Infrastructure Engineer · Leapcell
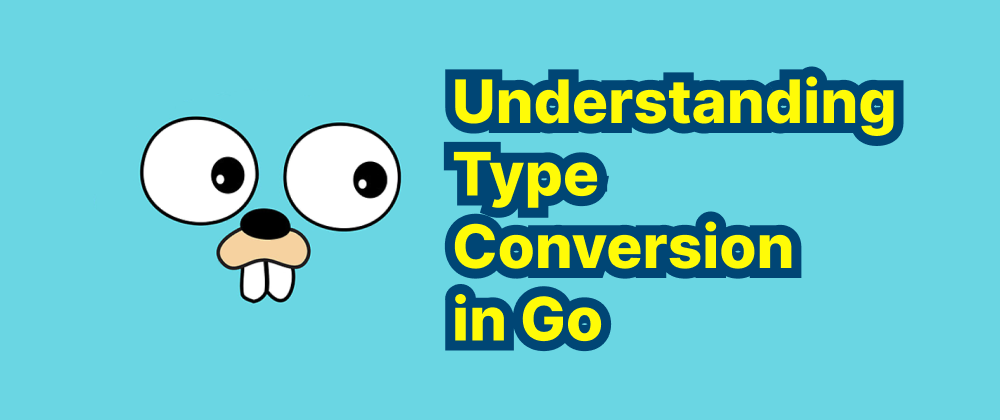
Key Takeaways
- Go requires explicit type conversion to maintain type safety.
- Strings and numeric types require specialized conversion functions.
- Type assertions are used for interface conversions.
In Go programming, type conversion (often referred to as "casting" in other languages) is essential for converting a value from one data type to another. Go emphasizes type safety, so explicit type conversions are necessary when dealing with different data types.
Basic Type Conversion
To convert between basic types, such as integers and floats, you can use the following syntax:
package main import ( "fmt" ) func main() { var i int = 42 var f float64 = float64(i) var u uint = uint(f) fmt.Println(i, f, u) }
In this example, an integer i
is converted to a float64 f
, and then to an unsigned integer u
. Each conversion requires an explicit type conversion to ensure type safety.
String Conversion
Converting between strings and other types requires specific functions:
-
To convert a numeric type to a string, use
fmt.Sprintf
orstrconv.Itoa
(for integers):package main import ( "fmt" "strconv" ) func main() { var i int = 42 var s1 string = strconv.Itoa(i) var s2 string = fmt.Sprintf("%d", i) fmt.Println(s1, s2) }
-
To convert a string to a numeric type, use functions like
strconv.Atoi
(for integers) orstrconv.ParseFloat
:package main import ( "fmt" "strconv" ) func main() { var s string = "42" i, err := strconv.Atoi(s) if err != nil { fmt.Println(err) } else { fmt.Println(i) } }
Interface Conversion
In Go, the empty interface interface{}
can hold values of any type. To retrieve the original value, you need to perform a type assertion:
package main import ( "fmt" ) func main() { var i interface{} = 42 if v, ok := i.(int); ok { fmt.Println(v) } else { fmt.Println("Type assertion failed") } }
Here, the type assertion i.(int)
checks if i
holds an int
. If it does, v
will be assigned the value, and ok
will be true. Otherwise, ok
will be false, and the assertion fails gracefully.
Pointer Conversion
Go allows pointer conversions between types that have the same underlying type:
package main import ( "fmt" ) type MyInt int func main() { var i int = 42 var p *int = &i var mp *MyInt = (*MyInt)(p) fmt.Println(*p, *mp) }
In this case, MyInt
is a defined type with int
as its underlying type. A pointer to int
can be converted to a pointer to MyInt
and vice versa.
Conclusion
Type conversion in Go requires explicit syntax to maintain type safety and clarity. Understanding how to perform these conversions is crucial for effective Go programming.
FAQs
Go enforces type safety to prevent unexpected behavior and errors.
Use strconv.Atoi
for simple cases or strconv.ParseInt
for more control.
If not handled properly, it causes a runtime panic; using the ok
pattern prevents this.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ