Is Golang Object-Oriented?
Grace Collins
Solutions Engineer · Leapcell
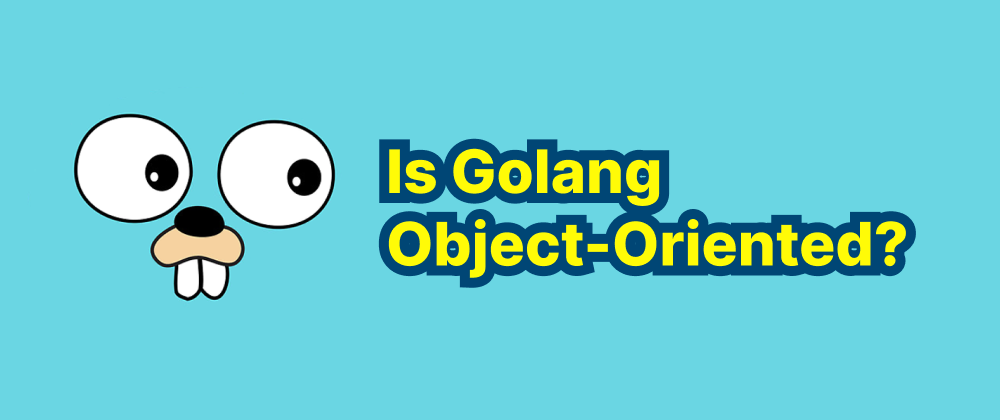
Key Takeaways
- Go does not have traditional class-based inheritance but supports object-oriented design through composition and interfaces.
- Structs and methods enable encapsulation, while interfaces promote polymorphism without explicit implementation.
- Go prioritizes composition over inheritance, reducing complexity in software design.
Is Golang Object-Oriented?
The question of whether Go, often referred to as Golang, qualifies as an object-oriented programming (OOP) language has been a topic of discussion among developers. To address this, it's essential to understand both the principles of OOP and the features that Go offers.
Understanding Object-Oriented Programming
Object-oriented programming is a paradigm centered around the concept of "objects"—entities that encapsulate data and behavior. The core principles of OOP include:
- Encapsulation: Bundling data and methods that operate on that data within a single unit, typically a class.
- Inheritance: Allowing a class to inherit characteristics (data and behavior) from another class.
- Polymorphism: Enabling entities to take on multiple forms, allowing for methods to operate differently based on the object they are acting upon.
- Abstraction: Hiding complex implementation details and exposing only the necessary components.
Go's Approach to Object-Oriented Concepts
Go does not have traditional classes or inheritance as seen in languages like Java or C++. However, it embodies several OOP principles through its unique constructs:
-
Structs and Methods: In Go, structs serve as the foundation for creating complex types. Methods can be associated with structs, allowing for behavior to be defined on these types. This combination provides encapsulation.
type User struct { Name string Email string } func (u User) Notify() { fmt.Printf("Sending email to %s\n", u.Email) }
-
Interfaces: Go's interfaces provide a way to define behavior. Any type that implements the methods specified in an interface satisfies that interface, promoting polymorphism. Notably, Go's interfaces are satisfied implicitly, meaning there's no need for explicit declarations.
type Notifier interface { Notify() } func SendNotification(n Notifier) { n.Notify() }
Here, any type with a
Notify
method satisfies theNotifier
interface, allowing for flexible and decoupled code. -
Composition over Inheritance: Instead of classical inheritance, Go promotes composition. By embedding structs within other structs, Go allows for the reuse of code and shared behavior without the complexities of inheritance hierarchies.
type Admin struct { User // Embedding User struct Level string }
In this example,
Admin
inherits the fields and methods ofUser
through embedding, facilitating code reuse and modular design.
Perspectives from the Go Community
The Go community acknowledges that while Go supports object-oriented programming styles, it intentionally diverges from traditional OOP paradigms:
-
The Go FAQ states:
"Yes and no. Although Go has types and methods and allows an object-oriented style of programming, there is no type hierarchy."
-
Discussions among developers highlight that Go's design favors composition over inheritance, aligning with modern software engineering practices that aim to reduce the pitfalls associated with deep inheritance chains.
Conclusion
While Go does not fit the mold of traditional class-based object-oriented languages, it provides robust features—such as structs, methods, and interfaces—that enable developers to employ object-oriented design principles effectively. By emphasizing composition and interface-based polymorphism, Go offers a flexible and efficient approach to modeling complex systems, embodying the essence of object-oriented programming in a manner suited to contemporary development needs.
FAQs
No, Go uses composition instead of classical inheritance to share behavior.
Go uses interfaces, which any type can implement implicitly.
Yes, but it follows a different paradigm, focusing on composition and interfaces rather than class hierarchies.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ