Understanding `make` and Map Initialization (like `make map`) in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
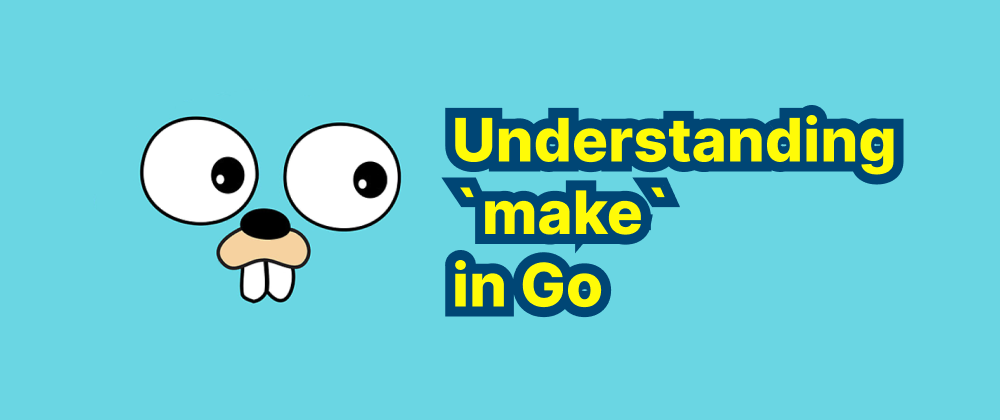
Key Takeaways
- The
make
function initializes and allocates memory for maps, making them immediately usable. new
returns a pointer to a nil map, requiring additional initialization before use.- Map literals provide a concise way to declare and initialize maps with predefined key-value pairs.
In Go, maps are essential data structures that store unordered key-value pairs, allowing for efficient data retrieval using keys. Proper initialization of maps is crucial to avoid runtime errors and ensure optimal performance. This article delves into the role of the make
function in map initialization and compares it with other methods of creating maps in Go.
The Role of make
in Map Initialization
The make
function in Go is a built-in function specifically designed to initialize and allocate memory for slices, maps, and channels. When it comes to maps, make
not only allocates the necessary memory but also ensures that the map is ready for use. Here's how you can use make
to create a map:
dict := make(map[string]int)
In this example, dict
is a map with keys of type string
and values of type int
. The make
function initializes the map, allowing you to add key-value pairs immediately:
dict["Alice"] = 30
Attempting to add elements to a map that hasn't been properly initialized will result in a runtime panic. Therefore, using make
is the standard and recommended approach to ensure that the map is ready for operations.
Comparing make
with Other Map Creation Methods
While make
is the conventional method for initializing maps, Go provides other ways to declare maps, each with its nuances.
Using Map Literals
Map literals allow for the declaration and initialization of maps in a concise manner. This approach is particularly useful when you know the initial key-value pairs at the time of declaration:
dict := map[string]int{ "Alice": 30, "Bob": 25, }
This syntax not only declares the map but also initializes it with the provided key-value pairs. The map is ready for use immediately after this declaration.
Using the new
Function
The new
function in Go allocates memory and returns a pointer to the zero value of the specified type. When used with maps, it returns a pointer to a nil map, which isn't immediately ready for use:
dictPtr := new(map[string]int)
Here, dictPtr
is a pointer to a nil map. To use this map, you must first initialize it using make
:
*dictPtr = make(map[string]int) (*dictPtr)["Alice"] = 30
Alternatively, you can assign it to a fully initialized map:
initializedMap := make(map[string]int) *dictPtr = initializedMap
It's important to note that using new
alone doesn't allocate the necessary underlying data structures for the map, leading to a nil map that cannot store key-value pairs until properly initialized.
Key Differences Between make
and new
-
Initialization:
make
initializes and allocates memory for the map, making it ready for use immediately. In contrast,new
allocates memory for a map pointer but doesn't initialize the underlying map, requiring an additional step to make the map usable. -
Return Type:
make
returns an initialized map, whereasnew
returns a pointer to a nil map.
Conclusion
Understanding the differences between make
, new
, and map literals is essential for effective map initialization in Go. The make
function is tailored for initializing maps, slices, and channels, ensuring that they are ready for immediate use. While new
can allocate memory for a map pointer, it doesn't initialize the map itself, necessitating further steps before the map can be utilized. Map literals offer a concise way to declare and initialize maps with known key-value pairs. By choosing the appropriate initialization method, you can write more robust and error-free Go programs.
FAQs
make
initializes the map for immediate use, while new
only allocates memory for a pointer, leaving the map uninitialized.
Use a map literal when you know the initial key-value pairs at declaration for concise and readable code.
Attempting to assign key-value pairs to a nil map results in a runtime panic, requiring proper initialization with make
or a map literal.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ