Using PostgreSQL in Go: A Comprehensive Guide
Daniel Hayes
Full-Stack Engineer · Leapcell
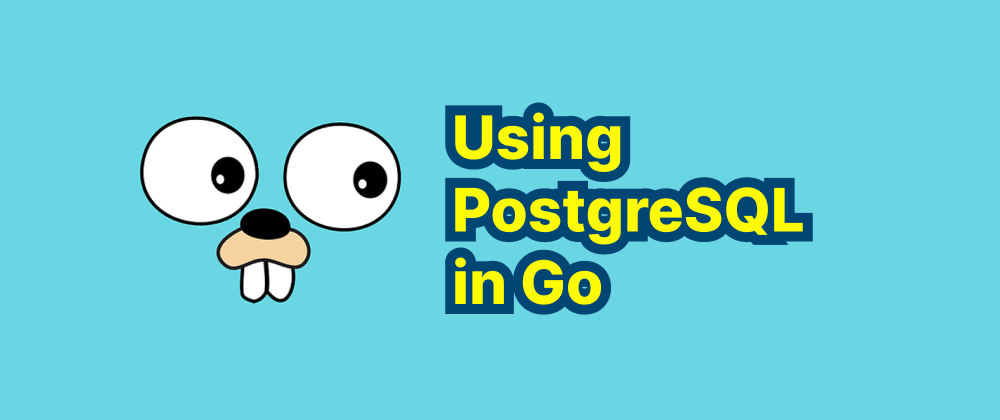
Key Takeaways
- Go can efficiently connect and interact with PostgreSQL using
database/sql
andlib/pq
. - Basic CRUD operations (Create, Read, Update, Delete) can be performed seamlessly in Go.
- Proper error handling is essential for maintaining a stable database connection.
PostgreSQL is a powerful, open-source object-relational database system known for its robustness and standards compliance. When combined with Go (Golang), a statically typed, compiled programming language designed for simplicity and efficiency, developers can build high-performance applications with efficient data management capabilities. This article provides a step-by-step guide on integrating PostgreSQL with Go, covering setup, connection, and basic CRUD (Create, Read, Update, Delete) operations.
Prerequisites
Before diving into code, ensure you have the following installed:
-
Go Environment: Download and install Go from the official website.
-
PostgreSQL: Install PostgreSQL suitable for your operating system from the official PostgreSQL website.
-
Database Driver: Go's
database/sql
package requires a driver to communicate with PostgreSQL. Thegithub.com/lib/pq
package is a popular choice. Install it using:go get github.com/lib/pq
Setting Up PostgreSQL
-
Start PostgreSQL Service: Ensure the PostgreSQL service is running.
-
Create a Database: Access the PostgreSQL prompt and create a new database:
CREATE DATABASE mydatabase;
-
Create a Table: Within your database, create a
users
table:CREATE TABLE users ( id SERIAL PRIMARY KEY, username VARCHAR(100) NOT NULL, email VARCHAR(100) NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
Connecting to PostgreSQL in Go
To interact with PostgreSQL, establish a connection using Go's database/sql
package and the pq
driver.
-
Import Packages: Include necessary packages in your Go file:
import ( "database/sql" "fmt" _ "github.com/lib/pq" )
-
Define Connection Parameters: Specify your PostgreSQL credentials:
const ( host = "localhost" port = 5432 user = "your_username" password = "your_password" dbname = "mydatabase" )
-
Establish Connection: Use the
sql.Open
function to connect:func main() { psqlInfo := fmt.Sprintf("host=%s port=%d user=%s password=%s dbname=%s sslmode=disable", host, port, user, password, dbname) db, err := sql.Open("postgres", psqlInfo) if err != nil { panic(err) } defer db.Close() // Verify connection err = db.Ping() if err != nil { panic(err) } fmt.Println("Successfully connected!") }
CRUD Operations
Create
Insert a new record into the users
table:
sqlStatement := ` INSERT INTO users (username, email) VALUES ($1, $2) RETURNING id` id := 0 err := db.QueryRow(sqlStatement, "johndoe", "[email protected]").Scan(&id) if err != nil { panic(err) } fmt.Printf("New record ID is: %d\n", id)
Read
Retrieve records from the users
table:
rows, err := db.Query("SELECT id, username, email, created_at FROM users") if err != nil { panic(err) } defer rows.Close() for rows.Next() { var id int var username, email string var createdAt time.Time err = rows.Scan(&id, &username, &email, &createdAt) if err != nil { panic(err) } fmt.Printf("%d: %s (%s) - %s\n", id, username, email, createdAt) }
Update
Modify an existing record:
sqlStatement := ` UPDATE users SET email = $2 WHERE id = $1;` res, err := db.Exec(sqlStatement, id, "[email protected]") if err != nil { panic(err) } count, err := res.RowsAffected() if err != nil { panic(err) } fmt.Printf("%d records updated\n", count)
Delete
Remove a record from the table:
sqlStatement := ` DELETE FROM users WHERE id = $1;` res, err := db.Exec(sqlStatement, id) if err != nil { panic(err) } count, err := res.RowsAffected() if err != nil { panic(err) } fmt.Printf("%d records deleted\n", count)
Error Handling
Robust error handling is crucial. Implement a helper function:
func checkErr(err error) { if err != nil { log.Fatal(err) } }
Use checkErr(err)
after operations to handle any errors gracefully.
Conclusion
Integrating PostgreSQL with Go enables developers to build efficient and reliable applications. By following this guide, you can set up a PostgreSQL database, connect to it using Go, and perform basic CRUD operations. For more advanced functionalities, consider exploring ORMs like GORM or SQL builders that can simplify complex queries and enhance productivity.
FAQs
Use go get github.com/lib/pq
to install the PostgreSQL driver.
Use db.Ping()
to verify the connection after calling sql.Open()
.
Implement a helper function like checkErr(err)
and use log.Fatal(err)
for debugging.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ