Simplifying HTTP Requests in Go with Resty
Daniel Hayes
Full-Stack Engineer · Leapcell
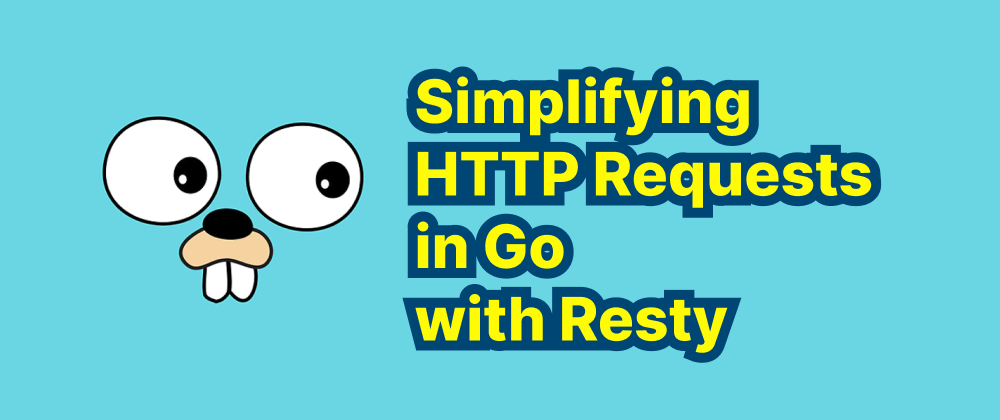
Key Takeaways
- Resty provides a concise and chainable API for making HTTP requests in Go.
- It supports automatic JSON unmarshalling, making data handling easier.
- Advanced features like retries, authentication, and middleware improve efficiency.
In the Go programming ecosystem, handling HTTP requests is a common task. While Go's standard library provides the net/http
package for this purpose, developers often seek more feature-rich and user-friendly alternatives. Enter Resty, a robust HTTP client library for Go that simplifies and enhances the process of making HTTP requests.
Overview of Resty
Resty is an HTTP client library for Go that offers a rich set of features and a simple API. It supports almost all HTTP methods (GET, POST, PUT, DELETE, OPTIONS, HEAD, PATCH, etc.) and provides an easy-to-use interface.
Key Features
- Chainable Methods: Resty allows chainable method calls, enabling concise and readable code.
- Automatic Unmarshalling: It can automatically unmarshal JSON or XML responses into Go structs.
- Flexible Request Configuration: Resty provides methods to set query parameters, headers, cookies, and more.
- Built-in Retries: It supports automatic retries with customizable policies.
- Request and Response Middleware: Developers can add middleware functions to process requests and responses.
Getting Started with Resty
To begin using Resty, first install it using Go Modules:
$ go get -u github.com/go-resty/resty/v2
Here's a simple example of making a GET request to fetch a webpage:
package main import ( "fmt" "log" "github.com/go-resty/resty/v2" ) func main() { client := resty.New() resp, err := client.R().Get("https://example.com") if err != nil { log.Fatal(err) } fmt.Println("Status Code:", resp.StatusCode()) fmt.Println("Response Body:", resp.String()) }
In this example:
- A new Resty client is created.
- A GET request is made to "https://example.com".
- The status code and response body are printed.
Automatic Unmarshalling
Resty simplifies handling structured data by automatically unmarshalling JSON responses into Go structs. Consider the following example where we fetch a list of libraries from an API:
type Library struct { Name string Latest string } type Libraries struct { Results []*Library } func main() { client := resty.New() libraries := &Libraries{} _, err := client.R(). SetResult(libraries). Get("https://api.cdnjs.com/libraries") if err != nil { log.Fatal(err) } fmt.Printf("Number of libraries: %d\n", len(libraries.Results)) if len(libraries.Results) > 0 { fmt.Printf("First library: Name: %s, Latest Version: %s\n", libraries.Results[0].Name, libraries.Results[0].Latest) } }
Here, Resty automatically unmarshals the JSON response into the Libraries
struct, making it easy to work with the data.
Advanced Usage: Fetching GitHub Repositories
Let's explore a more advanced use case where we fetch repositories from a GitHub organization using Resty. GitHub's API requires authentication via a personal access token.
First, generate a personal access token from your GitHub account settings. Then, use the following code:
type Repository struct { Name string `json:"name"` StargazersCount int `json:"stargazers_count"` ForksCount int `json:"forks_count"` } func main() { client := resty.New() var repos []Repository _, err := client.R(). SetAuthToken("your_github_token"). SetHeader("Accept", "application/vnd.github.v3+json"). SetQueryParams(map[string]string{ "per_page": "3", "page": "1", "sort": "created", "direction": "asc", }). SetPathParams(map[string]string{ "org": "golang", }). SetResult(&repos). Get("https://api.github.com/orgs/{org}/repos") if err != nil { log.Fatal(err) } for i, repo := range repos { fmt.Printf("Repo %d: Name: %s, Stars: %d, Forks: %d\n", i+1, repo.Name, repo.StargazersCount, repo.ForksCount) } }
In this example:
- We define a
Repository
struct to hold relevant data. - A Resty client is created.
- We set the authentication token, headers, query parameters, and path parameters.
- The response is automatically unmarshalled into the
repos
slice. - We iterate over the repositories and print their details.
Conclusion
Resty is a powerful and flexible HTTP client library for Go that enhances productivity by providing a rich set of features and a user-friendly API. Its capabilities, such as chainable methods, automatic unmarshalling, and flexible request configurations, make it a valuable tool for developers working with HTTP requests in Go.
FAQs
Resty offers a more user-friendly API, automatic unmarshalling, and built-in features like retries.
Yes, it supports token-based authentication and custom headers for API security.
Yes, its efficiency, middleware support, and retry mechanisms make it ideal for production use.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ