Exploring Golang's Validation Libraries
Grace Collins
Solutions Engineer · Leapcell
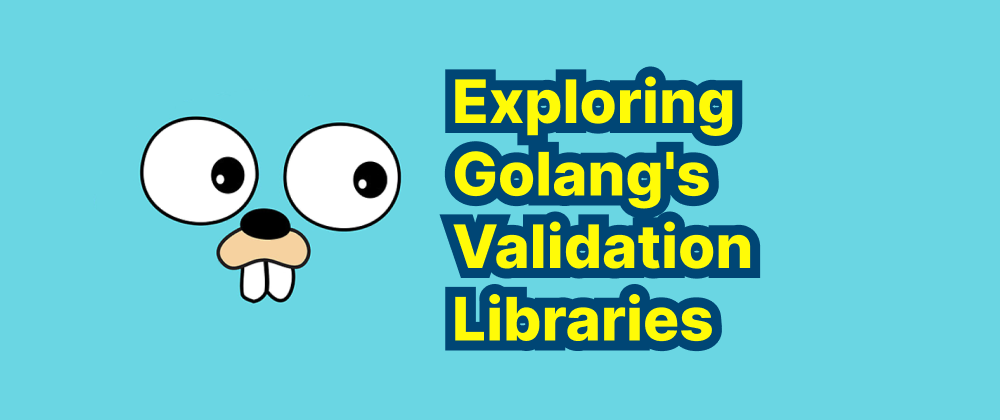
Key Takeaways
- The
validator
library uses struct tags for concise and efficient validation. - The
ozzo-validation
library offers a flexible, code-based validation approach. - Choosing between the two depends on project complexity and validation needs.
Data validation is a crucial aspect of software development, ensuring that the input data meets the required standards before processing. In the Go programming language, several libraries facilitate this process, with validator
and ozzo-validation
being among the most prominent. This article delves into these libraries, highlighting their features, usage, and differences.
The validator
Library
The validator
library, developed by the Go Playground community, is a widely adopted solution for struct field validation in Go. It employs struct tags to define validation rules, making the code both concise and readable.
Installation
To incorporate validator
into your project, use the following command:
go get github.com/go-playground/validator/v10
Basic Usage
Define your struct with appropriate validation tags:
package main import ( "fmt" "github.com/go-playground/validator/v10" ) type User struct { Name string `validate:"required,min=3,max=32"` Email string `validate:"required,email"` Age int `validate:"gte=18,lte=100"` } func main() { validate := validator.New() user := &User{ Name: "Alice", Email: "alice@example.com", Age: 30, } err := validate.Struct(user) if err != nil { fmt.Println("Validation errors:", err) } else { fmt.Println("Validation passed!") } }
In this example, the User
struct has three fields with validation rules specified using struct tags:
Name
: Must be present (required
), with a length between 3 and 32 characters.Email
: Must be present and follow a valid email format.Age
: Must be between 18 and 100, inclusive.
Common Validation Tags
The validator
library offers a comprehensive set of validation tags:
required
: Ensures the field is not empty.len
: Specifies the exact length for strings, slices, arrays, or maps.min
andmax
: Define minimum and maximum values for numbers or lengths for strings, slices, arrays, or maps.eq
andne
: Assert that the field is equal or not equal to a given value.lt
,lte
,gt
,gte
: Represent less than, less than or equal to, greater than, and greater than or equal to comparisons, respectively.oneof
: Ensures the field matches one of the specified values.
For a comprehensive list of validation tags and their usage, refer to the official documentation.
Custom Validation Functions
Beyond the built-in tags, validator
allows the creation of custom validation functions. For instance, to validate that a string starts with "Go":
func startsWithGo(fl validator.FieldLevel) bool { return strings.HasPrefix(fl.Field().String(), "Go") } func main() { validate := validator.New() validate.RegisterValidation("startsWithGo", startsWithGo) type Language struct { Name string `validate:"startsWithGo"` } lang := &Language{Name: "Golang"} err := validate.Struct(lang) if err != nil { fmt.Println("Validation errors:", err) } else { fmt.Println("Validation passed!") } }
In this snippet, the custom validation function startsWithGo
checks if the Name
field starts with "Go".
The ozzo-validation
Library
Another robust validation library in the Go ecosystem is ozzo-validation
. Unlike validator
, which relies on struct tags, ozzo-validation
uses code to define validation rules, offering greater flexibility and control.
Installation
To add ozzo-validation
to your project, execute:
go get github.com/go-ozzo/ozzo-validation/v4
Basic Usage
Here's how you can define and validate a struct using ozzo-validation
:
package main import ( "fmt" "github.com/go-ozzo/ozzo-validation/v4" "github.com/go-ozzo/ozzo-validation/v4/is" ) type User struct { Name string Email string Age int } func (u User) Validate() error { return validation.ValidateStruct(&u, validation.Field(&u.Name, validation.Required, validation.Length(3, 32)), validation.Field(&u.Email, validation.Required, is.Email), validation.Field(&u.Age, validation.Min(18), validation.Max(100)), ) } func main() { user := User{ Name: "Alice", Email: "alice@example.com", Age: 30, } err := user.Validate() if err != nil { fmt.Println("Validation errors:", err) } else { fmt.Println("Validation passed!") } }
In this approach, the User
struct has a Validate
method that specifies the validation rules using the validation
package's functions.
Key Features
- Fluent API: Validation rules are defined using a fluent interface, enhancing readability.
- Conditional Validation: Supports conditional validation rules based on other field values.
- Custom Validators: Allows the creation of custom validation rules tailored to specific requirements.
Choosing Between validator
and ozzo-validation
Both libraries are powerful tools for data validation in Go, but they have distinct approaches:
validator
: Utilizes struct tags for validation rules, leading to concise code. It's widely used and integrates seamlessly with frameworks like Gin. However, complex validation logic might become cumbersome with struct tags alone.ozzo-validation
: Employs code to define validation rules, offering greater flexibility and clarity, especially for intricate validation scenarios. This approach benefits from compile-time checks and reduces the likelihood of silent errors due to misconfigured tags.
The choice between the two depends on the project's needs:
- For straightforward validations with minimal logic,
validator
provides a clean and efficient solution. - For complex validations requiring conditional logic or custom rules,
ozzo-validation
might be more appropriate.
Conclusion
Data validation is essential in ensuring the integrity and reliability of software applications. Go's ecosystem offers robust libraries like validator
and ozzo-validation
to facilitate this process. Understanding their features and differences enables developers to select the most suitable tool for their specific use cases, leading to more maintainable and error-free codebases.
FAQs
validator
uses struct tags, while ozzo-validation
defines rules in code.
Yes, it allows custom validation functions to extend its capabilities.
ozzo-validation
is better suited for complex and conditional validation rules.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ