Simplifying Testing in Go with Testify
James Reed
Infrastructure Engineer · Leapcell
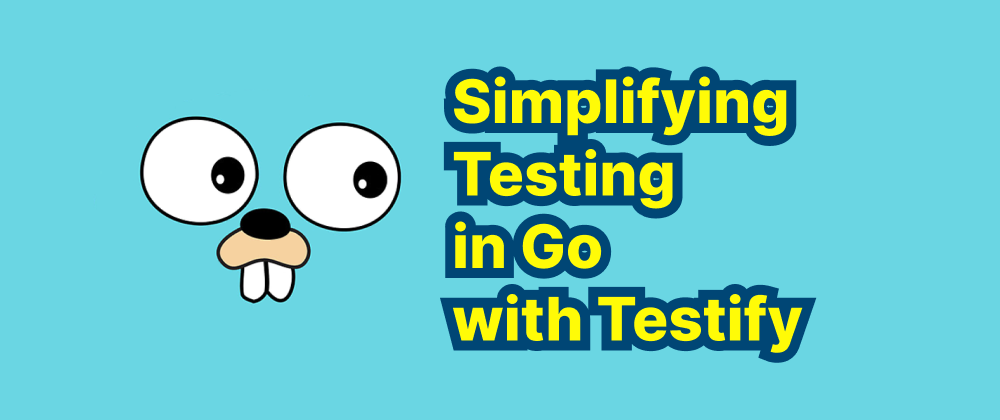
Key Takeaways
- Testify enhances Go testing with powerful assertions, mocking, and test suites.
- Assertions improve test readability and simplify validation.
- Mocking and test suites streamline unit testing for complex applications.
Testing is a crucial aspect of software development, ensuring code reliability and maintainability. In Go, while the standard testing
package provides basic functionalities, the Testify toolkit offers a richer set of features to enhance the testing experience.
What is Testify?
Testify is a comprehensive testing toolkit for Go that provides:
- Assertions: Simplified methods to assert conditions in tests.
- Mocking: Tools to create mock objects and control their behavior.
- Test Suites: Structures to group and manage tests with setup and teardown functionalities.
Installing Testify
To integrate Testify into your Go project, use the following command:
go get github.com/stretchr/testify
This command fetches the Testify package and makes it available for use in your tests.
Using Assertions with Testify
Assertions are fundamental in testing to validate that code behaves as expected. Testify's assert
package offers a variety of assertion functions that make tests more readable and concise.
Example:
package main import ( "testing" "github.com/stretchr/testify/assert" ) func TestAddition(t *testing.T) { result := Add(2, 3) assert.Equal(t, 5, result, "they should be equal") }
In this example, assert.Equal
checks if the Add
function returns the expected result. If not, the test fails, and the provided message is displayed.
Mocking with Testify
Mocking is essential when testing components that depend on external systems or complex interactions. Testify's mock
package facilitates the creation of mock objects to simulate these dependencies.
Example:
package main import ( "testing" "github.com/stretchr/testify/mock" ) // Define a mock object type MockService struct { mock.Mock } func (m *MockService) PerformAction() error { args := m.Called() return args.Error(0) } // Test function using the mock func TestAction(t *testing.T) { mockService := new(MockService) mockService.On("PerformAction").Return(nil) err := mockService.PerformAction() assert.NoError(t, err) mockService.AssertExpectations(t) }
In this scenario, MockService
simulates the behavior of an actual service, allowing isolated testing of the PerformAction
method.
Organizing Tests with Test Suites
Testify's suite
package enables the organization of related tests into test suites, providing setup and teardown functionalities for better test management.
Example:
package main import ( "testing" "github.com/stretchr/testify/suite" ) type ExampleTestSuite struct { suite.Suite Value int } func (suite *ExampleTestSuite) SetupTest() { suite.Value = 5 } func (suite *ExampleTestSuite) TestValue() { suite.Equal(5, suite.Value) } func TestExampleTestSuite(t *testing.T) { suite.Run(t, new(ExampleTestSuite)) }
Here, ExampleTestSuite
groups related tests, with SetupTest
initializing common values before each test.
Benefits of Using Testify
- Enhanced Readability: Testify's expressive assertion methods make tests easier to read and understand.
- Comprehensive Assertions: A wide range of assertion functions caters to various testing scenarios.
- Effective Mocking: Simplifies the creation and management of mock objects, essential for unit testing.
- Structured Test Suites: Facilitates organized testing with setup and teardown capabilities.
Conclusion
Testify significantly enhances Go's testing capabilities by providing a robust set of tools for assertions, mocking, and test suite management. By integrating Testify into your Go projects, you can write more maintainable and readable tests, leading to higher code quality and reliability.
FAQs
Testify provides more expressive assertions, better mocking support, and structured test suites.
Testify’s mock
package allows creating mock objects and setting expectations for unit tests.
Test suites organize tests, enable shared setup/teardown, and improve test maintainability.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ