Redis Data Types Explained: When and How to Use Them
Grace Collins
Solutions Engineer · Leapcell
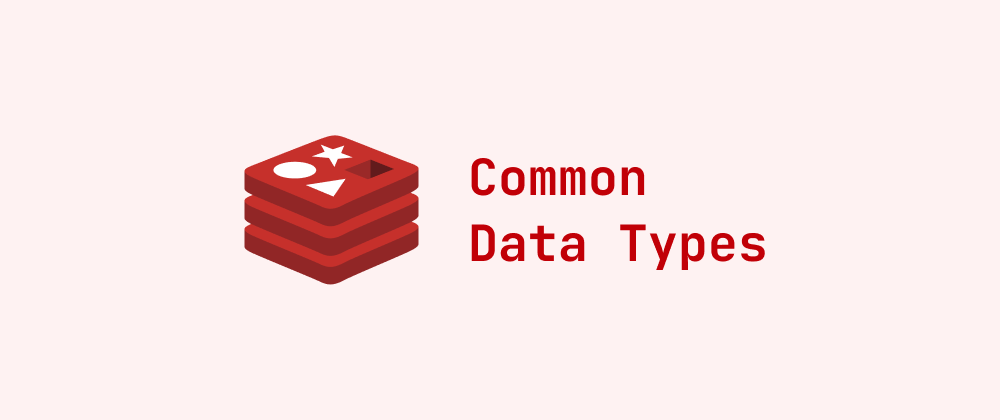
Common Redis Data Types
Strings
Strings in Redis are a fundamental data type used for storing and retrieving string values.
- Use Cases: Suitable for storing text or numbers, such as usernames, email addresses, or page counters. Strings can also store binary data, making them ideal for saving images or serialized objects.
- Advantages: Simple operations with atomic actions like incrementing (INCR).
// Save a string let _: () = conn.set("username", "alice").await.unwrap(); // Retrieve a string let username: String = conn.get("username").await.unwrap();
Lists
Redis lists are collections of strings sorted by insertion order.
- Use Cases: Ideal for implementing message queues, activity logs, or lists of recently accessed items. Lists support adding or removing elements from either end and can be used as stacks or queues.
- Advantages: Fast insertion and deletion operations. Suitable for FIFO (First-In-First-Out) queues or LIFO (Last-In-First-Out) stacks.
// Add elements to the head of a list let _: () = conn.lpush("events", "login").await.unwrap(); let _: () = conn.lpush("events", "logout").await.unwrap(); // Retrieve list elements let events: Vec<String> = conn.lrange("events", 0, -1).await.unwrap();
Sets
Sets are unordered collections of unique strings.
- Use Cases: Ideal for storing unique elements without a specific order, such as tags, visited IP addresses, or friend lists in a social network.
- Advantages: Fast operations for adding, deleting, and checking the existence of elements. Supports set operations like union, intersection, and difference.
// Add elements to a set let _: () = conn.sadd("tags", "redis").await.unwrap(); let _: () = conn.sadd("tags", "database").await.unwrap(); // Retrieve all set members let tags: Vec<String> = conn.smembers("tags").await.unwrap();
Sorted Sets
Sorted sets are similar to sets but associate each member with a score.
- Use Cases: Suitable for storing data that needs to be sorted by score, such as leaderboards, priority queues, or data with weighted sorting.
- Advantages: Besides basic set operations, it supports retrieving elements by score or lexicographical order and fetching elements within specific ranges.
// Add elements to a sorted set let _: () = conn.zadd("leaderboard", "alice", 100).await.unwrap(); let _: () = conn.zadd("leaderboard", "bob", 200).await.unwrap(); // Retrieve sorted set elements let leaderboard: Vec<(String, f64)> = conn.zrange_withscores("leaderboard", 0, -1).await.unwrap();
Hashes
Hashes are collections of key-value pairs, similar to dictionaries or objects in programming languages.
- Use Cases: Ideal for storing objects or multiple related data points, such as user attributes (name, age, email, etc.).
- Advantages: Efficient for reading or writing multiple fields at once, making it suitable for representing objects or aggregating data points.
// Add key-value pairs to a hash let _: () = conn.hset("user:100", "email", "alice@example.com").await.unwrap(); // Retrieve all key-value pairs from the hash let user_info: HashMap<String, String> = conn.hgetall("user:100").await.unwrap();
Bitmaps
Bitmaps are arrays of bits where each bit can be independently set or queried.
- Use Cases: Ideal for scenarios that require marking existence, such as user sign-ins or toggling feature statuses.
- Advantages: Highly space-efficient for handling large volumes of boolean values.
// Set a bit in the bitmap let _: () = conn.setbit("features", 0, true).await.unwrap(); // Enable feature 0 // Retrieve the value of a bit let feature_enabled: bool = conn.getbit("features", 0).await.unwrap();
HyperLogLogs
HyperLogLog is a probabilistic data structure used for efficiently estimating the cardinality (the number of unique elements).
- Use Cases: Ideal for estimating unique counts in large datasets, such as website unique visitors.
- Advantages: Extremely memory-efficient compared to traditional counting methods, especially when handling large datasets.
// Add elements to a HyperLogLog let _: () = conn.pfadd("pageviews", "user1").await.unwrap(); let _: () = conn.pfadd("pageviews", "user2").await.unwrap(); // Retrieve the approximate cardinality let unique_pageviews: i64 = conn.pfcount("pageviews").await.unwrap();
Choosing Data Types
Selecting the appropriate Redis data type for backend operations is crucial for achieving optimal storage and retrieval efficiency. Understanding your data structure and use case is key. Below are common backend scenarios with recommended Redis data types:
User Sessions
- Recommended Type: Hash
- Reason: Hashes can store multiple properties of a user session object (e.g., user ID, token, last access time) and allow individual updates or retrievals.
let _: () = conn.hset("session:userid", "token", "abc123").await.unwrap(); let _: () = conn.hset("session:userid", "last_access", "2023-01-01").await.unwrap();
Real-time Messaging or Event Queuing
- Recommended Type: List
- Reason: Lists provide FIFO queue characteristics, suitable for real-time messaging or task queues.
let _: () = conn.rpush("events_queue", "event1").await.unwrap(); let event: String = conn.lpop("events_queue").await.unwrap();
Access Counters or Rate Limiting
- Recommended Type: String
- Reason: Strings support atomic increment operations, ideal for counters.
let _: () = conn.incr("page_view_count", 1).await.unwrap(); let count: i64 = conn.get("page_view_count").await.unwrap();
Leaderboards or Score Sorting
- Recommended Type: Sorted Set
- Reason: Sorted sets automatically sort data based on scores, perfect for leaderboards or any sorted data.
let _: () = conn.zadd("leaderboard", "user123", 2500).await.unwrap(); let leaderboard: Vec<(String, f64)> = conn.zrange_withscores("leaderboard", 0, -1).await.unwrap();
Unique Value Collections like Tags or Categories
- Recommended Type: Set
- Reason: Sets provide unique value storage, suitable for deduplication scenarios.
let _: () = conn.sadd("tags", "redis").await.unwrap(); let tags: Vec<String> = conn.smembers("tags").await.unwrap();
Multi-attribute Object Storage
- Recommended Type: Hash
- Reason: Hashes can store multiple fields of an object and allow independent access or updates.
let _: () = conn.hset("user:100", "name", "Alice").await.unwrap(); let user: HashMap<String, String> = conn.hgetall("user:100").await.unwrap();
Feature Flags or Toggles
- Recommended Type: Bitmap
- Reason: Bitmaps are ideal for storing boolean states like feature toggles.
let _: () = conn.setbit("features", 1, true).await.unwrap(); let feature_on: bool = conn.getbit("features", 1).await.unwrap();
Conclusion
Choosing the right type depends on your specific needs. If your data structure is simple (like a single key-value pair), a string may suffice. For more complex structures, like user profiles or session information, hashes may be better. If your application involves leaderboards or sorted data, use sorted sets accordingly.
We are Leapcell, your top choice for hosting backend projects, with a built-in serverless Redis.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ