Comparing Strings in Python
Daniel Hayes
Full-Stack Engineer · Leapcell
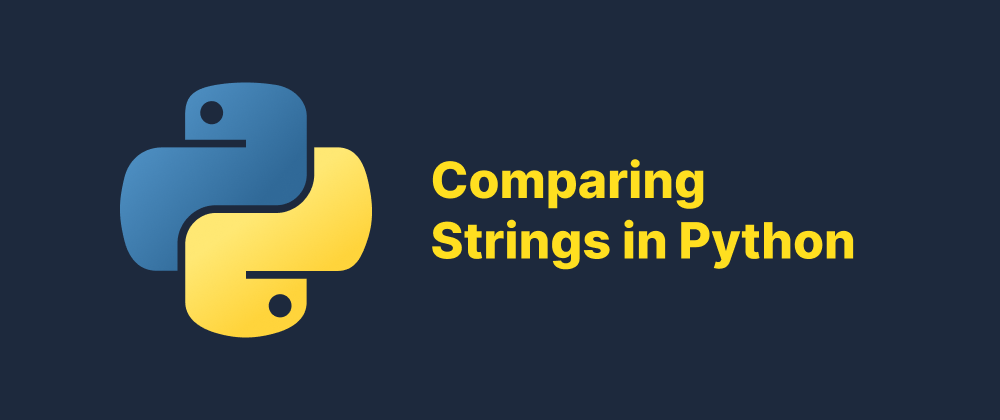
Key Takeaways
- Python string comparisons are case-sensitive by default.
- Use
==
for value equality, notis
. - For advanced matching, use the
re
module.
String comparison is a fundamental operation in Python, essential for tasks like sorting, searching, and validating user input. This article explores various methods for comparing strings in Python, covering equality checks, lexicographical comparisons, case sensitivity, and more.
Equality and Inequality Operators
The simplest way to compare two strings in Python is by using the equality (==
) and inequality (!=
) operators.
string1 = "apple" string2 = "banana" print(string1 == string2) # Output: False print(string1 != string2) # Output: True
These operators perform case-sensitive comparisons. For instance, "Apple"
and "apple"
are considered different strings.
Lexicographical Comparison
Python allows for lexicographical (dictionary order) comparisons using relational operators: <
, >
, <=
, and >=
.
print("apple" < "banana") # Output: True print("apple" > "banana") # Output: False
These comparisons are based on the Unicode code points of the characters. Notably, uppercase letters have lower Unicode values than lowercase letters, so "Apple"
is considered less than "apple"
.
Case Sensitivity in Comparisons
By default, string comparisons in Python are case-sensitive. To perform case-insensitive comparisons, you can convert both strings to the same case using .lower()
or .upper()
methods.
string1 = "Python" string2 = "python" print(string1.lower() == string2.lower()) # Output: True
This approach ensures that the comparison is not affected by the case of the letters.
Identity vs. Equality: is
vs. ==
It's important to distinguish between the is
operator and the ==
operator:
==
checks if the values of two objects are equal.is
checks if two references point to the same object in memory.
For string comparisons, always use ==
to compare values.
a = "hello" b = "hello" print(a == b) # Output: True print(a is b) # Output: True (but don't rely on this for value comparison)
While a is b
may return True
for small strings due to interning, it's not reliable for comparing string values.
Checking Substrings: in
and not in
To check if a substring exists within another string, use the in
and not in
operators.
sentence = "The quick brown fox" print("quick" in sentence) # Output: True print("slow" not in sentence) # Output: True
These operators are useful for simple substring checks.
Prefix and Suffix Checks: startswith()
and endswith()
Python provides methods to check if a string starts or ends with a specific substring.
filename = "example.txt" print(filename.startswith("ex")) # Output: True print(filename.endswith(".txt")) # Output: True
These methods return boolean values indicating whether the condition is met.
Finding Substrings: find()
and index()
To locate the position of a substring within a string, use the find()
or index()
methods.
text = "hello world" print(text.find("world")) # Output: 6 print(text.find("Python")) # Output: -1
The find()
method returns the lowest index of the substring if found, otherwise -1
. The index()
method behaves similarly but raises a ValueError
if the substring is not found.
Counting Substring Occurrences: count()
The count()
method returns the number of non-overlapping occurrences of a substring.
text = "banana" print(text.count("a")) # Output: 3
This method is useful for frequency analysis of substrings.
Regular Expressions for Advanced Matching
For complex pattern matching, Python's re
module allows the use of regular expressions.
import re pattern = r"\d{3}-\d{2}-\d{4}" text = "My number is 123-45-6789." match = re.search(pattern, text) if match: print("Pattern found:", match.group()) # Output: Pattern found: 123-45-6789
Regular expressions enable sophisticated string matching beyond simple comparisons.
Conclusion
Python offers a variety of tools for string comparison, ranging from basic equality checks to advanced pattern matching with regular expressions. Understanding these methods allows for effective string manipulation and analysis in Python programming.
FAQs
Yes, string comparisons in Python are case-sensitive unless normalized with .lower()
or .upper()
.
==
compares values; is
compares object identity in memory.
Use the in
operator, e.g., "foo" in "foobar"
returns True
.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ