Go Prepared Statement: Guide and Examples
James Reed
Infrastructure Engineer · Leapcell
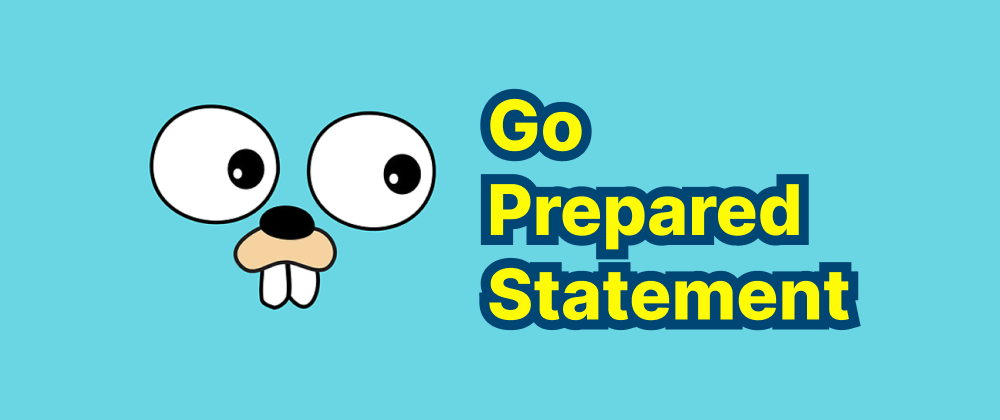
In Go, the database/sql
package provides robust support for prepared statements, which are beneficial for executing the same SQL statements multiple times efficiently and securely. Prepared statements help prevent SQL injection attacks and can improve performance by allowing the database to optimize the execution plan for repeated queries.
Key Takeaways
- Prepared statements improve security by preventing SQL injection.
- They enhance performance by optimizing query execution for repeated use.
- Proper resource management (closing statements, handling errors) is essential.
What is a Prepared Statement?
A prepared statement is a precompiled SQL statement that can be executed multiple times with different parameters. This approach separates the SQL logic from the data, enhancing security and performance.
Using Prepared Statements in Go
-
Import Necessary Packages
Begin by importing the required packages:
import ( "database/sql" "fmt" _ "github.com/go-sql-driver/mysql" // MySQL driver )
-
Establish a Database Connection
Create a connection to your database using
sql.Open
. Note thatsql.Open
does not establish a connection immediately but prepares the database handle for future use.db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/dbname") if err != nil { // Handle error panic(err) } defer db.Close()
-
Prepare the Statement
Use the
Prepare
method to create a prepared statement. This method returns ansql.Stmt
object, which can be executed multiple times with different parameters.stmt, err := db.Prepare("INSERT INTO users(name, age) VALUES(?, ?)") if err != nil { // Handle error panic(err) } defer stmt.Close()
In this example,
?
serves as a placeholder for the parameters that will be provided during execution. The exact placeholder syntax may vary depending on the database driver used. For instance, PostgreSQL uses$1
,$2
, etc., as placeholders. (go.dev) -
Execute the Prepared Statement
Execute the prepared statement with different sets of parameters using the
Exec
method._, err = stmt.Exec("Alice", 30) if err != nil { // Handle error panic(err) } _, err = stmt.Exec("Bob", 25) if err != nil { // Handle error panic(err) }
Each call to
Exec
executes the prepared statement with the provided parameters. -
Querying Data with Prepared Statements
Prepared statements can also be used for querying data. Here's an example of how to retrieve data using a prepared statement:
queryStmt, err := db.Prepare("SELECT id, name, age FROM users WHERE age > ?") if err != nil { // Handle error panic(err) } defer queryStmt.Close() rows, err := queryStmt.Query(20) if err != nil { // Handle error panic(err) } defer rows.Close() for rows.Next() { var id int var name string var age int err = rows.Scan(&id, &name, &age) if err != nil { // Handle error panic(err) } fmt.Printf("ID: %d, Name: %s, Age: %d\n", id, name, age) } if err = rows.Err(); err != nil { // Handle error panic(err) }
In this example, the prepared statement is used to select all users older than a specified age. The
Query
method executes the statement, and the results are iterated usingrows.Next()
. Each row's columns are read into variables using theScan
method.
FAQs
They prevent SQL injection and improve performance by reusing execution plans.
Yes, you can use them for INSERT
, UPDATE
, DELETE
, and SELECT
operations.
It may lead to resource leaks and unnecessary database load.
Best Practices
-
Close Statements: Always close your prepared statements using
stmt.Close()
to free up database resources. Usingdefer stmt.Close()
immediately after preparing the statement ensures that it will be closed when no longer needed. -
Error Handling: Implement robust error handling to catch and manage any issues that arise during database operations.
-
Connection Management: Remember that
sql.DB
manages a pool of connections. It's efficient to prepare statements once and reuse them, especially in applications that execute the same statements multiple times.
By following these steps and best practices, you can effectively utilize prepared statements in Go to write secure and efficient database applications.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ