Understanding Python's `dict.get()` Method
Daniel Hayes
Full-Stack Engineer · Leapcell
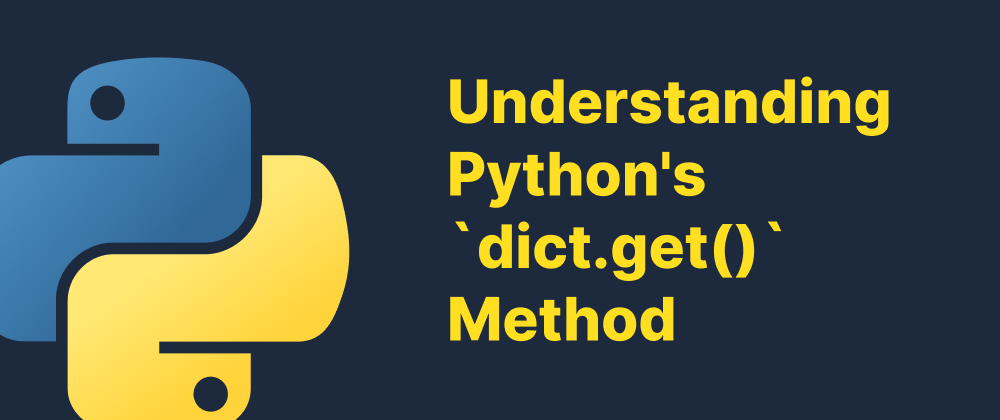
Key Takeaways
dict.get()
preventsKeyError
when accessing missing keys.- You can provide a default return value using
get()
. get()
enhances code readability and robustness.
In Python, dictionaries are versatile data structures that store key-value pairs, allowing for efficient data retrieval. Accessing values in a dictionary typically involves using square brackets ([]
) with the key:
my_dict = {'name': 'Alice', 'age': 30} print(my_dict['name']) # Output: Alice
However, if you attempt to access a key that doesn't exist using this method, Python raises a KeyError
:
print(my_dict['gender']) # Raises KeyError
To handle such situations gracefully, Python provides the get()
method.
What is the get()
Method?
The get()
method allows you to retrieve the value associated with a given key. If the key exists in the dictionary, get()
returns its corresponding value. If the key is absent, it returns a default value, which is None
unless specified otherwise. The syntax is:
dictionary.get(key, default_value)
Here:
key
: The key to search for in the dictionary.default_value
(optional): The value to return if the key is not found. If not provided, defaults toNone
.
Advantages of Using get()
- Avoiding KeyError: Using
get()
prevents the program from raising aKeyError
when a key is missing. - Providing Default Values: It allows specifying a default return value when the key is not present, making the code more robust and readable.
Examples
Basic Usage:
person = {'name': 'Alice', 'age': 30} # Key exists print(person.get('name')) # Output: Alice # Key doesn't exist print(person.get('gender')) # Output: None
Using a Default Value:
person = {'name': 'Alice', 'age': 30} # Key doesn't exist, provide a default value print(person.get('gender', 'Female')) # Output: Female
Difference Between get()
and Direct Access:
person = {'name': 'Alice', 'age': 30} # Using get() print(person.get('gender')) # Output: None # Using direct access print(person['gender']) # Raises KeyError
Practical Use Case
Consider a scenario where you need to count the occurrences of letters in a word. Using get()
, you can simplify the code:
word = 'hello' letter_count = {} for letter in word: letter_count[letter] = letter_count.get(letter, 0) + 1 print(letter_count) # Output: {'h': 1, 'e': 1, 'l': 2, 'o': 1}
In this example, letter_count.get(letter, 0)
retrieves the current count of the letter, defaulting to 0
if the letter isn't already in the dictionary. This approach avoids the need for conditional checks or exception handling when updating the count.
Conclusion
The get()
method is a powerful tool for dictionary operations in Python, offering a safe and concise way to access values. By providing a default value for missing keys, it enhances code readability and robustness, making it a preferred choice in many scenarios.
FAQs
dict.get()
returns None
or a default value if the key is missing, while square brackets raise a KeyError
.
Yes, get()
avoids the need for if
statements by returning a fallback value.
Slightly, but the performance difference is negligible for most use cases and often outweighed by code clarity.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ