How to Use `random.choice()` in Python
Grace Collins
Solutions Engineer · Leapcell
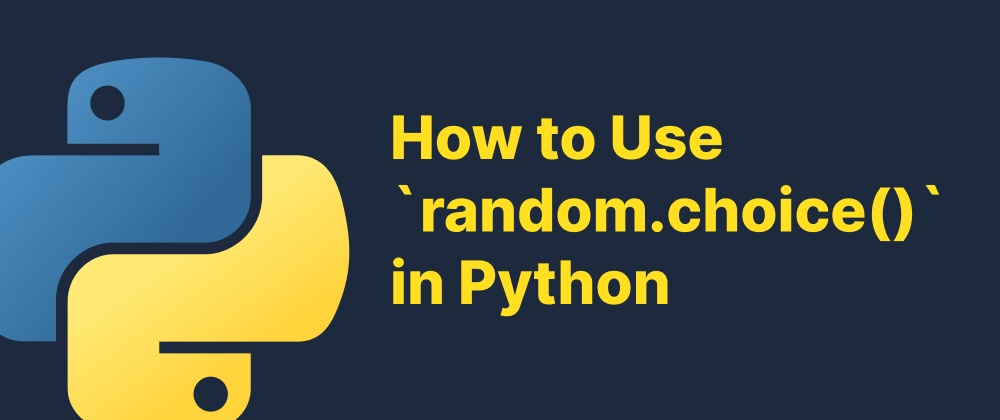
Key Takeaways
random.choice()
selects a random item from a non-empty sequence.- Always check that the sequence is not empty to avoid errors.
- Use
random.seed()
to make random choices reproducible.
When working with data in Python, you may occasionally need to select a random item from a list or sequence. The random
module in Python provides a convenient method called choice()
for exactly this purpose. This article will guide you through how to use random.choice()
effectively, along with practical examples and some important considerations.
What Is random.choice()
?
random.choice()
is a function in Python’s built-in random
module. It allows you to randomly select a single item from a non-empty sequence, such as a list, tuple, or string.
Syntax
import random random.choice(sequence)
sequence
: A non-empty sequence (like a list, tuple, or string).- Returns: A single randomly selected item from the sequence.
Basic Examples
Here are some common use cases:
Example 1: Choosing from a List
import random colors = ['red', 'green', 'blue', 'yellow'] chosen_color = random.choice(colors) print(chosen_color)
This code will print one color randomly selected from the list.
Example 2: Choosing from a String
import random word = "python" letter = random.choice(word) print(letter)
In this case, a random letter from the string "python"
will be selected.
Handling Edge Cases
It’s important to remember that random.choice()
will raise an IndexError
if the sequence is empty:
import random empty_list = [] random.choice(empty_list) # Raises IndexError
To avoid this, always check if the sequence is not empty before calling choice()
:
if my_list: item = random.choice(my_list) else: print("List is empty")
How to Make It Reproducible
If you want reproducible results for testing or debugging, set a random seed using random.seed()
:
import random random.seed(42) print(random.choice(['apple', 'banana', 'cherry']))
Using the same seed will ensure the same sequence of results every time the script runs.
Conclusion
The random.choice()
function is a powerful and easy-to-use tool in Python for selecting random elements from a sequence. Just be sure the sequence is not empty, and use random.seed()
when reproducibility is important. Whether you're building games, running simulations, or just experimenting with Python, random.choice()
is a tool worth mastering.
FAQs
You can use lists, tuples, strings, or any non-empty sequence.
random.choice()
raises an IndexError
if used on an empty sequence.
Use random.seed()
with a fixed value before calling random.choice()
.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ