Understanding the `return` Statement in Python
James Reed
Infrastructure Engineer · Leapcell
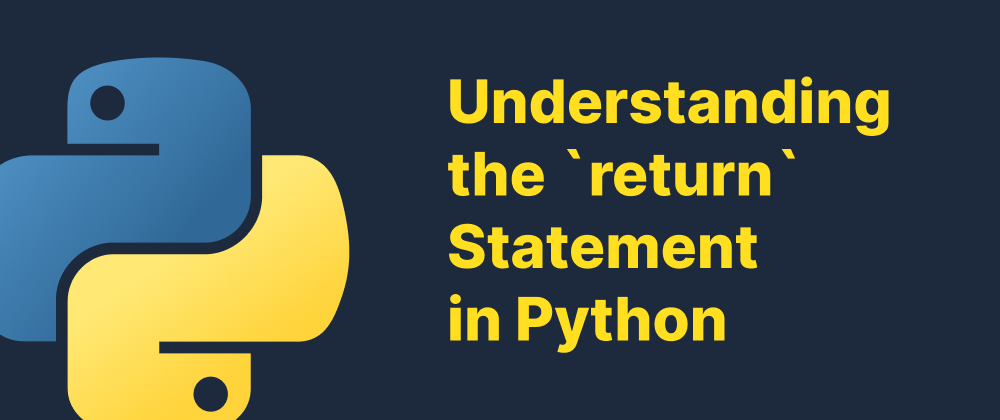
Key Takeaways
- The
return
statement ends a function and optionally sends back a value. - Functions can return multiple values as a tuple.
- Omitting
return
results in the function returningNone
by default.
In Python, the return
statement is used to exit a function and send a result back to the caller. It is one of the most fundamental aspects of function design, allowing values to be passed back after a computation or process.
What Does return
Do?
When a function reaches a return
statement, it immediately ends execution and returns the specified value. If no value is specified, the function returns None
by default.
def greet(): return "Hello, World!" message = greet() print(message) # Output: Hello, World!
In the above example, the function greet()
returns the string "Hello, World!"
, which is then assigned to the variable message
.
Returning Multiple Values
Python allows a function to return multiple values as a tuple. This is a powerful feature that can make your code more expressive and concise.
def get_coordinates(): x = 10 y = 20 return x, y coords = get_coordinates() print(coords) # Output: (10, 20) print(coords[0]) # Output: 10 print(coords[1]) # Output: 20
Conditional Returns
You can use return
statements conditionally, depending on logic within the function.
def check_even(number): if number % 2 == 0: return True return False
This function returns True
only if the number is even.
Returning None
If no return
statement is used, or if a function hits the end of its block without encountering return
, it returns None
by default.
def do_nothing(): pass result = do_nothing() print(result) # Output: None
Using return
to Exit Early
Sometimes, return
is used to exit a function early before reaching the end, especially when handling errors or invalid input.
def divide(a, b): if b == 0: return "Division by zero is not allowed." return a / b
Best Practices
- Use
return
to keep functions modular and predictable. - Avoid using multiple
return
statements in complex functions unless necessary—it can make debugging harder. - Document what your function returns to make your code more understandable.
Conclusion
The return
statement is essential in Python for sending values back from functions. Understanding how and when to use return
will help you write cleaner, more efficient code. Whether you're returning a simple value, multiple results, or exiting early due to an error condition, return
plays a key role in structuring your programs.
FAQs
It returns None
automatically.
Yes, Python functions can return multiple values as a tuple.
Yes, but use them carefully to avoid making the code harder to read.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ