Understanding Go's syscall Package
James Reed
Infrastructure Engineer · Leapcell
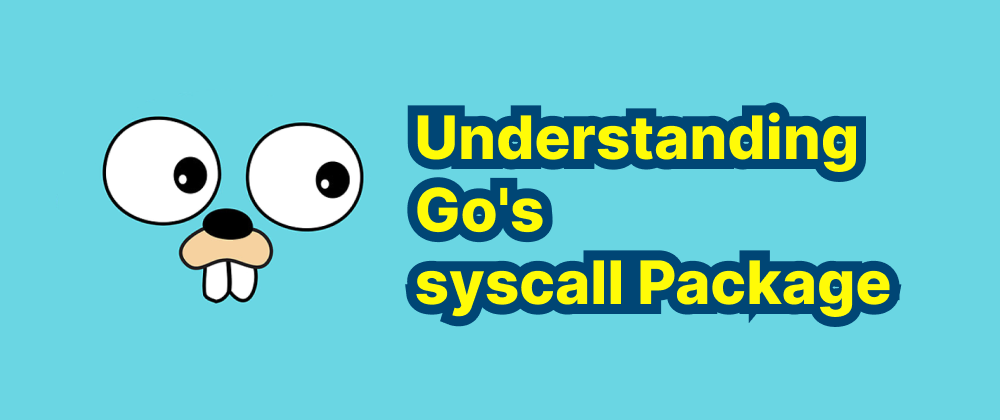
Key Takeaways
- The
syscall
package enables direct OS interaction but is now locked down in Go. - Developers should use
golang.org/x/sys
for better portability and maintenance. - System calls allow low-level operations like process management, file handling, and networking.
Introduction
In Go programming, the syscall
package provides an interface to low-level operating system primitives, enabling developers to perform system calls directly. This package serves as a bridge between Go applications and the underlying OS, facilitating operations such as process management, file handling, and network communication. However, it's important to note that the syscall
package has been locked down, and developers are encouraged to use the golang.org/x/sys
repository for new code.
Overview of the syscall Package
The syscall
package offers access to OS-level system calls, which are essential for tasks that require direct interaction with the operating system. These system calls allow programs to request services from the kernel, such as creating processes, reading and writing files, and managing memory.
Key Features
- Process Management: Functions like
syscall.ForkExec
andsyscall.Wait4
enable the creation and synchronization of processes. - File Operations: System calls such as
syscall.Open
,syscall.Read
, andsyscall.Write
allow direct file manipulation. - Networking: Low-level networking operations can be performed using functions like
syscall.Socket
,syscall.Bind
, andsyscall.Connect
.
Platform-Specific Implementations
The behavior and availability of certain system calls can vary between operating systems. For instance, the way system calls are invoked differs between Linux and Windows due to their distinct architectures and system interfaces.
Deprecation Notice and Alternative
As of Go 1.4, the syscall
package is locked down, and it's recommended to use the golang.org/x/sys
package for accessing system calls. This external package provides a more comprehensive and up-to-date set of system call wrappers, ensuring better portability and compatibility across different platforms.
Practical Example: Using syscall to Open a File
Below is an example demonstrating how to use the syscall
package to open a file and read its contents:
package main import ( "fmt" "syscall" "unsafe" ) func main() { // Open the file fd, err := syscall.Open("example.txt", syscall.O_RDONLY, 0) if err != nil { fmt.Println("Error opening file:", err) return } defer syscall.Close(fd) // Read from the file buf := make([]byte, 100) n, err := syscall.Read(fd, buf) if err != nil { fmt.Println("Error reading file:", err) return } // Convert the byte slice to a string and print fmt.Println("File contents:", *(*string)(unsafe.Pointer(&buf))) }
In this example:
syscall.Open
opens the file in read-only mode.syscall.Read
reads up to 100 bytes from the file into the buffer.- The contents of the buffer are then printed as a string.
Conclusion
The syscall
package in Go provides essential functionality for interacting directly with the operating system. However, due to its locked status, developers are advised to use the golang.org/x/sys
package for new projects to ensure better support and compatibility. Understanding how to perform system calls in Go is crucial for tasks that require low-level system interactions, but it's equally important to follow current best practices and utilize the recommended libraries.
FAQs
It has been locked down since Go 1.4; golang.org/x/sys
is recommended for better support.
They include file operations, process management, and low-level networking.
Yes, but it's not recommended due to portability and maintenance concerns.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ