Mastering Flask: A Deep Dive
Daniel Hayes
Full-Stack Engineer · Leapcell
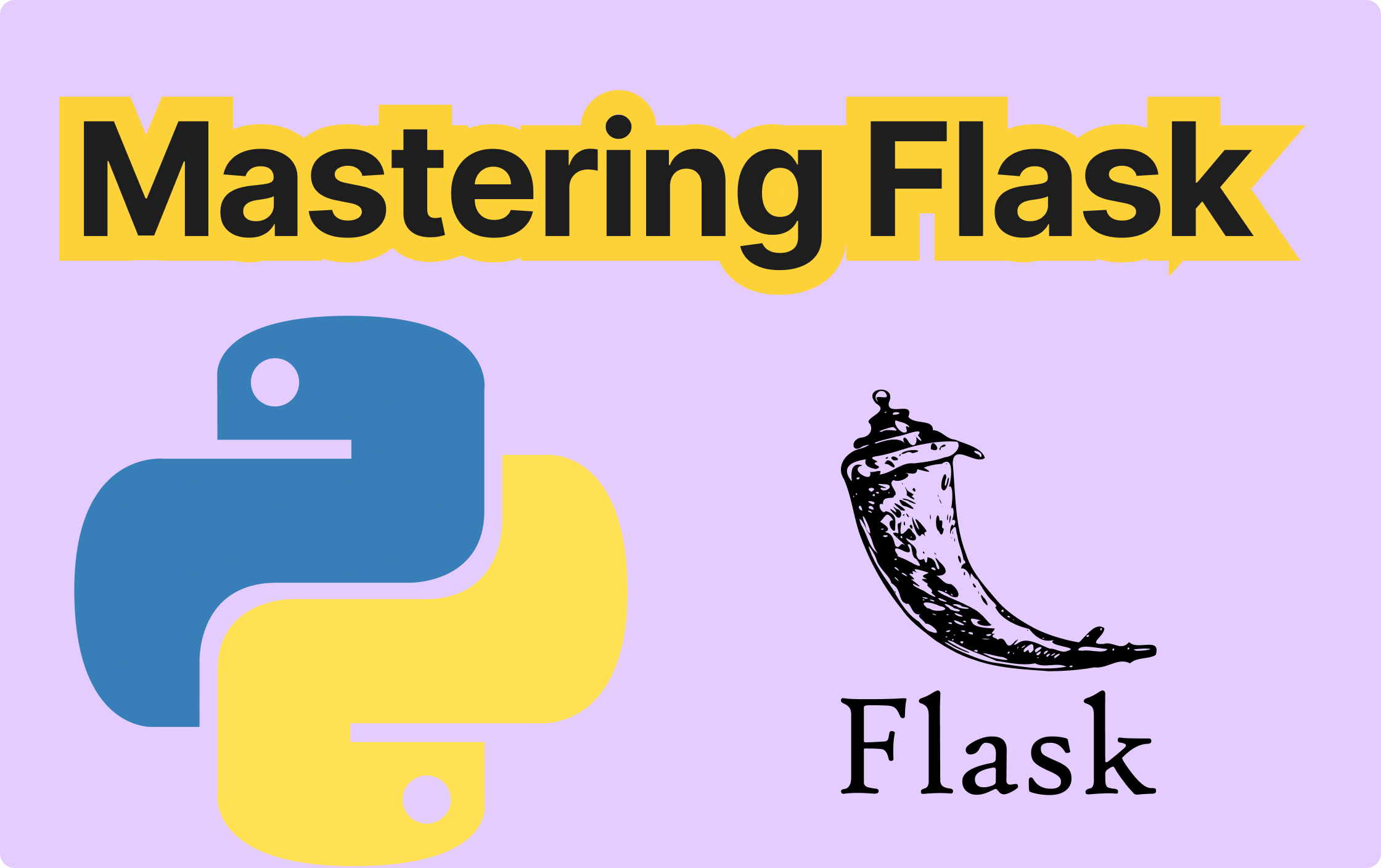
1. Background Introduction
Flask is a lightweight web framework. It is a Python web application used to build web services and APIs. Its design philosophy is "minimal dependencies", which means Flask only depends on two components: the Werkzeug WSGI toolkit and the Jinja 2 template engine.
Flask is an open - source Python web application framework that provides a simple way to create web services and APIs. Its design philosophy of "minimal dependencies" results in it relying only on the Werkzeug WSGI toolkit and the Jinja 2 template engine.
The core concepts of Flask include application, routing, request, response, context, configuration, etc. These concepts will be introduced in detail later.
2. Core Concepts and Their Relationships
In this section, we will introduce the core concepts of Flask in detail and explain the relationships between them.
2.1 Flask Application
The Flask application is a class that inherits from the Flask class. It contains the application's configuration, routing, and context. An application can be created by instantiating the Flask class.
from flask import Flask app = Flask(__name__)
2.2 Flask Routing
Routing is a core component of a Flask application. It defines the application's URLs and request methods, as well as the view functions associated with these URLs and request methods. Routes can be defined using the @app.route
decorator.
@app.route('/') def index(): return 'Hello, World!'
2.3 Flask Request
A request is an HTTP request sent by a client to the server. Flask provides a request
object to handle requests. The request
object contains various information about the request, such as the request method, URL, headers, query parameters, form data, etc.
from flask import request method = request.method url = request.url headers = request.headers # The original code here was wrong, it should be request.args query_params = request.args form_data = request.form
2.4 Flask Response
A response is an HTTP response sent by the server to the client. Flask provides a response
object to build responses. The response
object contains various information about the response, such as the status code, headers, content, etc.
from flask import Response response = Response(response=b'Hello, World!', status = 200, mimetype='text/plain')
2.5 Flask Context
The context is a scope within a Flask application. When handling a request, Flask creates a context to store information about the request and response. The context can be accessed through the current_app
and g
objects.
from flask import current_app app_name = current_app.name
2.6 Flask Configuration
Configuration refers to the settings of a Flask application. They can be accessed through the config
attribute. Configuration can be set through environment variables, configuration files, or code.
from flask import Flask app = Flask(__name__) app.config['DEBUG'] = True
3. Core Algorithm Principles, Specific Operation Steps, and Mathematical Model Formulas
In this section, we will introduce the core algorithm principles, specific operation steps, and mathematical model formulas of Flask in detail.
3.1 Flask Request Processing Flow
The Flask request processing flow includes the following steps:
- The client sends an HTTP request to the server.
- The server receives the HTTP request and creates a Werkzeug
Request
object. - The server parses the Werkzeug
Request
object and creates a FlaskRequest
object. - The server looks up the route associated with the request URL and calls the corresponding view function.
- The view function processes the request and creates a Flask
Response
object. - The server sends the response back to the client.
3.2 Flask Response Building
The Flask response building includes the following steps:
- Create a Flask
Response
object and set the response content, status code, and MIME type. - Set the response headers, such as
Content - Type
,Content - Length
, etc. - If the response content is HTML, set the response
Content - Type
totext/html
and render the response content as HTML using therender_template
function. - If the response content is JSON, set the response
Content - Type
toapplication/json
and convert the response content to JSON using thejsonify
function. - Send the response back to the client.
3.3 Flask Template Rendering
The Flask template rendering includes the following steps:
- Load the template file and parse the variables, tags, and filters in the template.
- Use the return value of the view function as the context of the template and render it as HTML.
- Send the rendered HTML back to the client.
4. Specific Code Examples and Detailed Explanations
In this section, we will explain the usage of Flask in detail through a specific code example.
4.1 Creating a Flask Application
First, we need to create a Flask application. We can achieve this with the following code:
from flask import Flask app = Flask(__name__)
4.2 Defining Routes
Next, we need to define a route. We can achieve this with the following code:
@app.route('/') def index(): return 'Hello, World!'
In the above code, we use the @app.route
decorator to define a route with the URL /
and associate it with a view function named index
. When the client accesses the /
URL, the server will call the index
function and send its return value back to the client.
4.3 Running the Flask Application
Finally, we need to run the Flask application. We can achieve this with the following code:
if __name__ == '__main__': app.run()
In the above code, we use the if __name__ == '__main__':
condition to determine whether the current script is being run directly. If so, the Flask application is run.
5. Future Development Trends and Challenges
In this section, we will discuss the future development trends and challenges of Flask.
5.1 Future Development Trends of Flask
The future development trends of Flask include the following aspects:
- Better Performance Optimization: Performance optimization of Flask will be an important direction for its future development. This includes aspects such as better request handling, response building, and template rendering.
- More Powerful Scalability: Scalability of Flask will be an important direction for its future development. This includes more third - party extensions, plugins, and middleware.
- Better Documentation and Tutorials: Documentation and tutorials of Flask will be an important direction for its future development. This includes more detailed documentation, more tutorials, and better sample code.
5.2 Challenges of Flask
The challenges of Flask include the following aspects:
- Performance Bottlenecks: Performance bottlenecks in Flask, including those in request handling, response building, and template rendering, will be one of its challenges.
- Scalability Limitations: Scalability limitations of Flask, including those related to third - party extensions, plugins, and middleware, will be one of its challenges.
- Learning Curve: The learning curve of Flask, including its core concepts, algorithm principles, and usage methods, will be one of its challenges.
6. Appendix: Frequently Asked Questions and Answers
In this section, we will answer some frequently asked questions about Flask.
6.1 How Does Flask Handle Static Files?
Flask handles static files through the url_for
function. We can achieve this with the following code:
from flask import url_for url_for('static', filename='style.css')
In the above code, we use the url_for
function to generate the URL of a static file. The url_for
function accepts a dictionary as a parameter, where the key is the name of the routing rule and the value is the parameter value.
6.2 How Does Flask Handle Form Data?
Flask handles form data through the request.form
object. We can achieve this with the following code:
from flask import request name = request.form['name']
In the above code, we use the request.form
object to obtain form data. The request.form
object is a dictionary where the keys are the form field names and the values are the form field values.
6.3 How Does Flask Handle File Uploads?
Flask handles file uploads through the request.files
object. We can achieve this with the following code:
from flask import request file = request.files['file']
In the above code, we use the request.files
object to obtain the file upload object. The request.files
object is a dictionary where the keys are the file field names and the values are the file upload objects.
6.4 How Does Flask Handle Sessions?
Flask handles sessions through the session
object. We can achieve this with the following code:
from flask import session session['key'] = 'value'
In the above code, we use the session
object to store session data. The session
object is a dictionary where the keys are session keys and the values are session values.
6.5 How Does Flask Handle Errors?
Flask handles errors through the @app.errorhandler
decorator. We can achieve this with the following code:
from flask import Flask app = Flask(__name__) @app.errorhandler(404) def not_found_error(e): return 'Not Found', 404
In the above code, we use the @app.errorhandler
decorator to define an error - handling function. The @app.errorhandler
decorator accepts a parameter, which is the error type. When an error of the specified type occurs, the server will call the error - handling function and pass the error object as a parameter.
7. Summary
In this article, we have introduced the background, core concepts, core algorithm principles, specific code examples, and future development trends of Flask in detail. We have also answered some frequently asked questions about Flask. We hope this article is helpful to you.
Leapcell: The Best Serverless Platform for Python app Hosting
Finally, I would like to recommend Leapcell, the best platform for deploying Python services.
1. Multi - Language Support
- Develop with JavaScript, Python, Go, or Rust.
2. Deploy unlimited projects for free
- Pay only for usage — no requests, no charges.
3. Unbeatable Cost Efficiency
- Pay - as - you - go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
4. Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real - time metrics and logging for actionable insights.
5. Effortless Scalability and High Performance
- Auto - scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the documentation!
Leapcell Twitter: https://x.com/LeapcellHQ