Understanding Global Variables in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
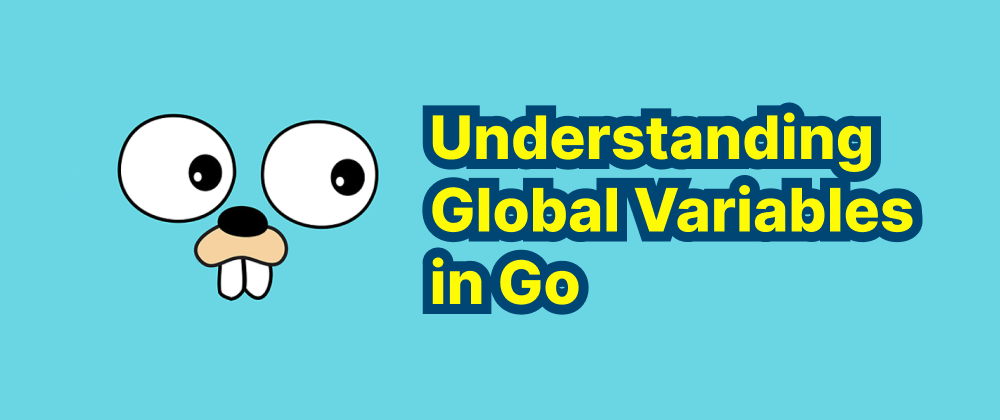
Key Takeaways
- Global variables in Go are accessible within the same package but should be used cautiously.
- Overuse of global variables can lead to uncontrolled access, tight coupling, and testing difficulties.
- Best practices like dependency injection and struct encapsulation help minimize reliance on global variables.
In Go, variables can be declared with different scopes, determining their accessibility throughout the program. One such scope is the global scope. This article delves into the concept of global variables in Go, their declaration, usage, and the considerations to keep in mind when utilizing them.
What Are Global Variables?
Global variables are those declared outside of any function, typically at the top of a Go source file. These variables are accessible from any function within the same package, maintaining their value throughout the program's execution. For example:
package main import "fmt" var globalVar = "I am global" func main() { fmt.Println(globalVar) anotherFunction() } func anotherFunction() { fmt.Println(globalVar) }
In this example, globalVar
is accessible both in main
and anotherFunction
, illustrating its global scope.
Declaring Global Variables
To declare a global variable in Go, use the var
keyword outside of any function:
package main var globalVar string = "Accessible throughout the package"
It's important to note that Go does not allow the shorthand :=
syntax for declaring global variables; this syntax is reserved for local variable declarations within functions.
Access Control
In Go, the visibility of global variables across packages is determined by the casing of the variable name:
-
Exported (Public) Variables: If a global variable's name starts with an uppercase letter, it is exported and can be accessed from other packages.
package main var ExportedVar = "I can be accessed from other packages"
-
Unexported (Package-Private) Variables: If a global variable's name starts with a lowercase letter, it is unexported and accessible only within the same package.
package main var unexportedVar = "I am accessible only within this package"
Potential Issues with Global Variables
While global variables can be convenient, they come with several drawbacks:
-
Uncontrolled Access: Any function within the package can modify global variables, making it challenging to track changes and debug issues.
-
Tight Coupling: Overreliance on global variables can lead to tightly coupled code, reducing modularity and flexibility.
-
Testing Difficulties: Global variables can hinder testing, as their state might persist across tests, leading to flaky or unreliable test results.
Best Practices to Avoid Global Variables
To mitigate the issues associated with global variables, consider the following practices:
-
Dependency Injection: Pass variables or dependencies as parameters to functions or methods, promoting explicitness and reducing hidden dependencies.
package main import "fmt" func printMessage(message string) { fmt.Println(message) } func main() { msg := "Hello, World!" printMessage(msg) }
-
Use of Structs: Encapsulate related variables within structs and define methods on these structs. This approach enhances data organization and encapsulation.
package main import "fmt" type Greeter struct { message string } func (g Greeter) greet() { fmt.Println(g.message) } func main() { greeter := Greeter{message: "Hello, Go!"} greeter.greet() }
-
Package-Level Variables: Limit the use of package-level variables and ensure they are unexported unless necessary. This practice confines their scope and reduces unintended interactions.
Conclusion
Global variables in Go offer a way to define package-wide accessible data. However, their use should be judicious, considering the potential pitfalls such as uncontrolled access and testing challenges. By adopting best practices like dependency injection and encapsulation within structs, developers can write more maintainable and robust Go applications.
FAQs
Only if they start with an uppercase letter, making them exported.
They can cause tight coupling, uncontrolled modifications, and testing difficulties.
Use dependency injection or encapsulate data within structs.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ