Understanding `__init__.py` in Python Packages
Daniel Hayes
Full-Stack Engineer · Leapcell
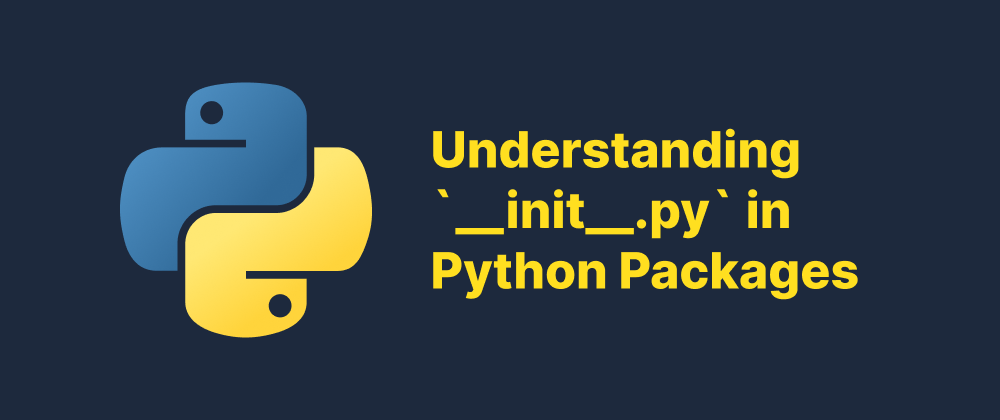
Key Takeaways
__init__.py
makes a directory a Python package and enables package initialization.- It simplifies and controls module imports within a package.
- Including it ensures compatibility across tools and Python versions.
When working with Python projects, especially those involving multiple modules and directories, you may encounter a file named __init__.py
. Although it may seem like just another file, it plays a key role in how Python handles packages and module imports. In this article, we’ll explore what __init__.py
does, why it exists, and how you can use it effectively.
What Is __init__.py
?
__init__.py
is a special Python file that is used to mark a directory as a Python package. Prior to Python 3.3, its presence was required for Python to recognize a directory as a package during imports. While modern Python versions support implicit namespace packages (i.e., packages without __init__.py
), the file is still widely used and recommended for several reasons.
Key Purposes of __init__.py
-
Package Initialization
Code inside__init__.py
is executed when the package is imported. This makes it a convenient place to put any package-level setup code.# mypackage/__init__.py print("Initializing mypackage")
When someone runs
import mypackage
, the message above will be printed. -
Controlling What Gets Imported
You can define the__all__
variable in__init__.py
to control which submodules are accessible when usingfrom mypackage import *
.__all__ = ['module1', 'module2']
-
Simplifying Imports
By importing submodules or symbols in__init__.py
, you can simplify how users of your package access its functionality.from .module1 import ClassA from .module2 import function_b
This allows users to write:
from mypackage import ClassA, function_b
instead of:
from mypackage.module1 import ClassA from mypackage.module2 import function_b
-
Maintaining Backward Compatibility
Many tools and libraries still expect__init__.py
to be present. Including it ensures broader compatibility and avoids subtle issues with tools that parse or package your code.
Example Structure
Here's an example of a simple Python package:
mypackage/
│
├── __init__.py
├── module1.py
└── module2.py
With this structure, you can access module1
and module2
as submodules of mypackage
.
Namespace Packages (Python 3.3+)
As of Python 3.3, you can create namespace packages without needing an __init__.py
file. This is useful for distributing a single logical package across multiple directories or distributions. However, these should be used with care, especially if you rely on initialization code or dynamic imports.
When Should You Include __init__.py
?
- Always, if you're writing code for wide compatibility or using tools like setuptools.
- When you want to include package-level initialization logic.
- When you want to simplify your package’s import interface.
- When you're working with complex or nested package structures.
Conclusion
Even though __init__.py
is no longer strictly required in all cases, it remains a crucial part of writing clean, organized, and user-friendly Python packages. Understanding how and when to use it helps you structure your code more effectively and makes your packages easier to use and maintain.
FAQs
No, but it’s recommended for compatibility and clarity.
Python executes the code inside __init__.py
.
Yes, by defining the __all__
list in __init__.py
.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ