How to Split Strings in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
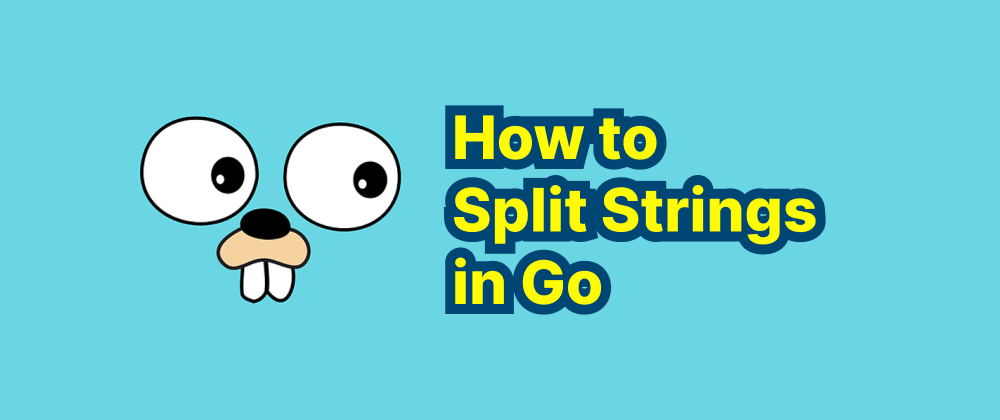
Key Takeaways
- Go provides multiple functions for splitting strings, including
strings.Fields
,strings.Split
, andstrings.FieldsFunc
. - Regular expressions can be used for complex string-splitting scenarios.
- Choosing the right method depends on the delimiter type and complexity of the split condition.
String manipulation is a fundamental aspect of programming, and splitting strings into substrings based on specific delimiters is a common task. In Go, the strings
package provides a variety of functions to handle such operations efficiently. This article explores different methods to split strings in Go, including splitting by spaces, specific characters or strings, multiple characters, and using regular expressions.
Splitting by Spaces
To split a string by spaces, Go offers the strings.Fields
function. This function divides the input string into substrings based on white space characters and returns a slice of these substrings.
package main import ( "fmt" "strings" ) func main() { str := " foo bar baz " fields := strings.Fields(str) fmt.Printf("Fields are: %q\n", fields) }
Output:
Fields are: ["foo" "bar" "baz"]
In this example, strings.Fields
ignores leading and trailing spaces and splits the string at each sequence of white space characters.
Splitting by Specific Characters or Strings
For splitting a string by a specific character or substring, the strings.Split
function is utilized. This function takes the input string and a separator, splitting the string at each occurrence of the separator.
package main import ( "fmt" "strings" ) func main() { str1 := "a,b,c" split1 := strings.Split(str1, ",") fmt.Printf("%q\n", split1) str2 := "a man a plan a canal panama" split2 := strings.Split(str2, "a ") fmt.Printf("%q\n", split2) }
Output:
["a" "b" "c"]
["" "man " "plan " "canal panama"]
In the first case, the string is split at each comma. In the second case, the string is split at each occurrence of the substring "a ".
Splitting by Multiple Characters
When the delimiter consists of multiple characters, strings.FieldsFunc
can be employed. This function splits the string based on a user-defined function that determines whether a character is a separator.
package main import ( "fmt" "strings" "unicode" ) func main() { str := " foo1;bar2,baz3..." f := func(c rune) bool { return !unicode.IsLetter(c) && !unicode.IsNumber(c) } fields := strings.FieldsFunc(str, f) fmt.Printf("Fields are: %q\n", fields) }
Output:
Fields are: ["foo1" "bar2" "baz3"]
In this example, strings.FieldsFunc
uses the function f
to determine separators. The function f
considers any character that is not a letter or number as a separator, effectively splitting the string at punctuation marks and spaces.
Splitting by Multiple Strings
As of Go 1.20, the standard library does not provide a function to split a string by multiple substrings directly. However, this functionality can be achieved by chaining calls to strings.Split
or by using regular expressions.
package main import ( "fmt" "regexp" ) func main() { str := "Hello, This is my, first code in, Golang!" re := regexp.MustCompile(",\\s*") split := re.Split(str, -1) fmt.Println(split) }
Output:
[Hello This is my first code in Golang!]
In this example, a regular expression is used to split the string at each comma followed by any number of spaces.
Splitting Using Regular Expressions
For more complex splitting scenarios, regular expressions provide a powerful tool. The regexp
package's Split
method allows splitting a string based on patterns.
package main import ( "fmt" "regexp" ) func main() { str := "Hello :), This is my, first code in, Golang!" re := regexp.MustCompile(`:\),\s*`) split := re.Split(str, -1) fmt.Println(split) }
Output:
[Hello This is my first code in Golang!]
Here, the regular expression :\),\s*
matches the smiley face :)
followed by any number of spaces, splitting the string accordingly.
Conclusion
Go provides robust tools for splitting strings through its strings
and regexp
packages. Depending on the specific requirements—such as the nature of the delimiter and the complexity of the split condition—developers can choose the appropriate function to efficiently divide strings into substrings. Understanding these functions and their applications is essential for effective string manipulation in Go.
FAQs
strings.Fields
splits based on whitespace and ignores empty fields, while strings.Split
splits at every occurrence of a specified delimiter.
Yes, you can use strings.FieldsFunc
for character-based splitting or regexp.Split
for splitting by multiple substrings.
No, but you can use regexp.Split
with a regular expression pattern to achieve this.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ