Testing Regular Expressions in Go: A Guide to Pattern Matching and Text Processing
Grace Collins
Solutions Engineer · Leapcell
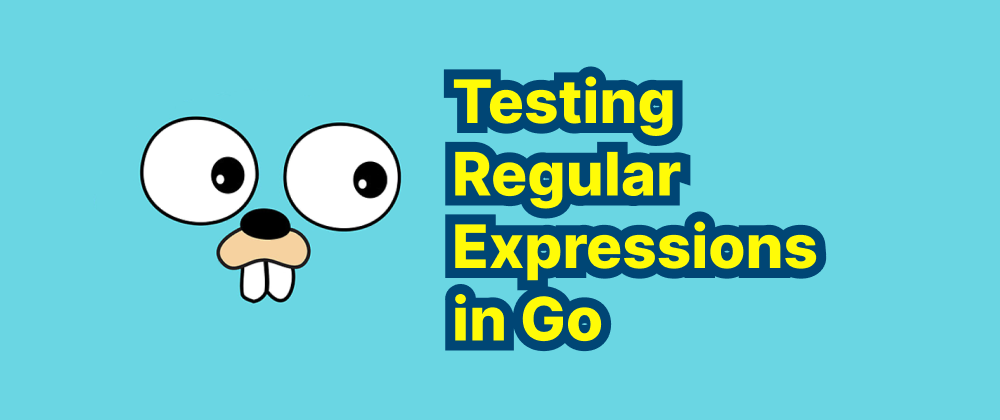
Key Takeaways
- Go's
regexp
package provides powerful tools for regex-based text processing. - Functions like
MatchString
,FindString
, andReplaceAllString
enable efficient pattern matching. - Understanding regex syntax helps in extracting and manipulating text effectively.
Regular expressions (regex) are powerful tools for pattern matching and text processing. In Go, the regexp
package provides robust support for working with regular expressions, enabling developers to perform tasks such as searching, matching, and replacing text efficiently.
Importing the regexp
Package
To utilize regular expressions in your Go programs, you need to import the regexp
package:
import "regexp"
Compiling Regular Expressions
Before using a regular expression, you must compile it. Go offers two functions for this purpose:
-
regexp.Compile
: Compiles the regular expression and returns aRegexp
object along with an error if the pattern is invalid. -
regexp.MustCompile
: Similar toCompile
, but panics if the pattern is invalid. It's useful when you're confident that the pattern is correct.
Example using regexp.MustCompile
:
re := regexp.MustCompile(`^Golang`)
Testing for Matches
To check if a string matches a regular expression, you can use the MatchString
method:
package main import ( "fmt" "regexp" ) func main() { re := regexp.MustCompile(`^Golang`) str := "Golang regular expressions example" match := re.MatchString(str) fmt.Println("Match:", match) }
Output:
Match: true
In this example, the regular expression ^Golang
checks if the string starts with "Golang". The MatchString
method returns true
because the string matches the pattern.
Finding Substrings
The FindString
method returns the first occurrence of the pattern in the input string:
package main import ( "fmt" "regexp" ) func main() { re := regexp.MustCompile(`p([a-z]+)e`) str := "Golang regular expressions example" match := re.FindString(str) fmt.Println("Found:", match) }
Output:
Found: pre
Here, the regular expression p([a-z]+)e
matches substrings that start with 'p' and end with 'e', with one or more lowercase letters in between. The FindString
method returns the first matching substring.
Finding Submatch Groups
If you want to extract specific parts of the matching string, you can use the FindStringSubmatch
method, which returns a slice containing the entire match and the submatches defined by parentheses in the pattern:
package main import ( "fmt" "regexp" ) func main() { re := regexp.MustCompile(`p([a-z]+)e`) str := "Golang regular expressions example" match := re.FindStringSubmatch(str) if match != nil { fmt.Println("Full match:", match[0]) fmt.Println("Submatch:", match[1]) } }
Output:
Full match: pre
Submatch: r
In this case, match[0]
contains the entire matched substring, while match[1]
contains the substring matched by the first capturing group ([a-z]+)
.
Replacing Substrings
The ReplaceAllString
method allows you to replace all occurrences of the pattern in the input string with a specified replacement:
package main import ( "fmt" "regexp" ) func main() { re := regexp.MustCompile(`examp([a-z]+)e`) str := "Golang regular expressions example" result := re.ReplaceAllString(str, "tutorial") fmt.Println("Result:", result) }
Output:
Result: Golang regular expressions tutorial
Here, the pattern examp([a-z]+)e
matches the word "example", and ReplaceAllString
replaces it with "tutorial".
Conclusion
Go's regexp
package provides a comprehensive set of tools for working with regular expressions, enabling efficient text processing and pattern matching. By understanding and utilizing functions like Compile
, MatchString
, FindString
, FindStringSubmatch
, and ReplaceAllString
, you can effectively implement regex-based solutions in your Go applications.
FAQs
Use regexp.MatchString(pattern, string)
or re.MatchString(string)
after compiling the regex.
regexp.Compile
returns an error if the pattern is invalid, while regexp.MustCompile
panics on errors.
Use FindStringSubmatch
, which returns both the full match and submatches in an array.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ