Reading a File Line by Line in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
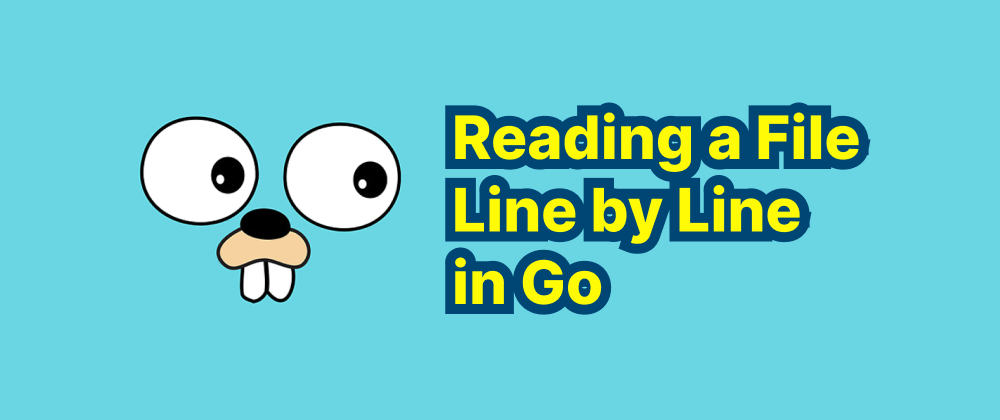
Key Takeaways
- Use
bufio.Scanner
for efficient line-by-line file reading. - Handle potential errors properly to avoid unexpected failures.
- Adjust buffer size for long lines using
Scanner.Buffer()
.
Reading files line by line is a common task in programming, especially when processing text files or logs. In Go, this can be efficiently achieved using the bufio
package, which provides buffered I/O operations. This article will guide you through the process of reading a file line by line using Go.
Using bufio.Scanner
The bufio.Scanner
is a convenient tool for reading data such as lines of text. It reads the input and splits it into tokens based on a specified delimiter, which defaults to newline characters, making it ideal for line-by-line reading.
Here's how you can use bufio.Scanner
to read a file line by line:
package main import ( "bufio" "fmt" "log" "os" ) func main() { // Open the file file, err := os.Open("path/to/your/file.txt") if err != nil { log.Fatalf("Failed to open file: %s", err) } defer file.Close() // Create a new Scanner for the file scanner := bufio.NewScanner(file) // Iterate over each line for scanner.Scan() { line := scanner.Text() // Process the line here fmt.Println(line) } // Check for errors during scanning if err := scanner.Err(); err != nil { log.Fatalf("Error reading file: %s", err) } }
In this example:
- We open the file using
os.Open
. It's important to handle any errors that may occur during this operation. - A
bufio.Scanner
is created for the file. The scanner defaults to splitting the input into lines. - We iterate over each line using
scanner.Scan()
. Thescanner.Text()
method returns the current line as a string. - After the loop, we check if any errors occurred during the scanning process using
scanner.Err()
.
Handling Long Lines
The bufio.Scanner
has a default buffer size, which may not be sufficient for extremely long lines. If you anticipate lines longer than 64KB, you can adjust the buffer size using the Scanner.Buffer
method:
scanner := bufio.NewScanner(file) buf := make([]byte, 0, 1024*1024) // 1 MB buffer scanner.Buffer(buf, 1024*1024)
This sets the buffer to 1MB, accommodating longer lines as needed.
Conclusion
Reading a file line by line in Go is straightforward with the bufio.Scanner
. It provides a simple and efficient way to process each line, handling common tasks such as reading logs or processing text files. Always ensure to handle potential errors gracefully and adjust the buffer size when working with files that may contain unusually long lines.
FAQs
It defaults to newline characters (\n
).
Use Scanner.Buffer()
to increase the buffer size.
Use scanner.Err()
to check and handle errors properly.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ