Merging Maps in Go: A Comprehensive Guide
Grace Collins
Solutions Engineer · Leapcell
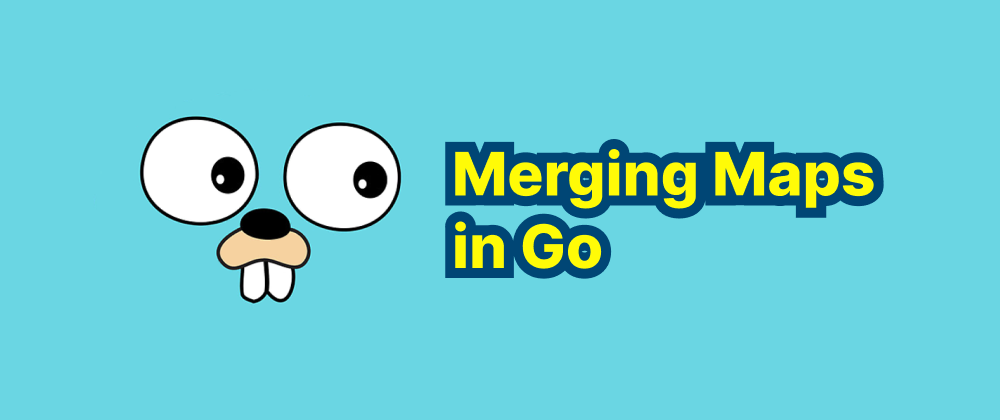
Key Takeaways
- Go 1.21 introduces
maps.Copy
, simplifying map merging. - Manual iteration is required for Go versions before 1.21.
- The
golang.org/x/exp/maps
package provides an alternative for Go 1.18-1.20.
In Go, maps are essential data structures that store key-value pairs, allowing for efficient data retrieval. Merging two maps is a common task that can be accomplished in various ways, depending on the Go version and specific requirements. This article explores different methods to merge maps in Go, from manual iteration to utilizing new language features introduced in recent versions.
Manual Iteration Method
Before Go 1.21, there wasn't a built-in function to merge maps directly. The idiomatic approach involved manually iterating over the source map and adding each key-value pair to the destination map. Here's how it can be done:
package main import "fmt" func main() { src := map[string]int{ "one": 1, "two": 2, } dst := map[string]int{ "two": 42, "three": 3, } for k, v := range src { dst[k] = v } fmt.Println("src:", src) fmt.Println("dst:", dst) }
Output:
src: map[one:1 two:2]
dst: map[one:1 three:3 two:2]
In this example, the key "two" exists in both maps. The value from the src
map overwrites the value in the dst
map, resulting in the merged map.
Using the maps.Copy
Function (Go 1.21 and Later)
With the release of Go 1.21, a new standard library function maps.Copy
was introduced, simplifying the process of copying key-value pairs from one map to another. This function is part of the maps
package and provides a concise way to merge maps:
package main import ( "fmt" "maps" ) func main() { src := map[string]int{ "one": 1, "two": 2, } dst := map[string]int{ "two": 42, "three": 3, } maps.Copy(dst, src) fmt.Println("src:", src) fmt.Println("dst:", dst) }
Output:
src: map[one:1 two:2]
dst: map[one:1 three:3 two:2]
The maps.Copy
function copies all key-value pairs from the src
map to the dst
map, overwriting existing keys with the values from src
. This method is straightforward and leverages the latest language features for improved code readability and maintainability.
Using the golang.org/x/exp/maps
Package (Go 1.18 to 1.20)
For Go versions 1.18 to 1.20, the experimental package golang.org/x/exp/maps
provides a Copy
function that offers similar functionality to the maps.Copy
introduced in Go 1.21. Here's how to use it:
package main import ( "fmt" "golang.org/x/exp/maps" ) func main() { src := map[string]int{ "one": 1, "two": 2, } dst := map[string]int{ "two": 42, "three": 3, } maps.Copy(dst, src) fmt.Println("src:", src) fmt.Println("dst:", dst) }
Output:
src: map[one:1 two:2]
dst: map[one:1 three:3 two:2]
It's important to note that the golang.org/x/exp
package is experimental, and its APIs may change in future releases. Therefore, caution is advised when using this package in production code.
Conclusion
Merging maps in Go can be achieved through various methods, each suited to different scenarios and Go versions:
- Manual Iteration: Suitable for all Go versions, providing explicit control over the merging process.
maps.Copy
Function: Available from Go 1.21 onwards, offering a concise and efficient way to merge maps.golang.org/x/exp/maps
Package: Applicable for Go 1.18 to 1.20, but as an experimental package, it should be used with caution.
When choosing a method, consider the Go version in use and the specific requirements of your application to select the most appropriate approach.
FAQs
The value from the source map overwrites the destination map’s value.
No, Go requires maps to have the same key and value types for merging.
No, maps.Copy
is only available in Go 1.21 and later.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ