Ternary Operator in Go: Why It’s Missing and Alternative Approaches
Daniel Hayes
Full-Stack Engineer · Leapcell
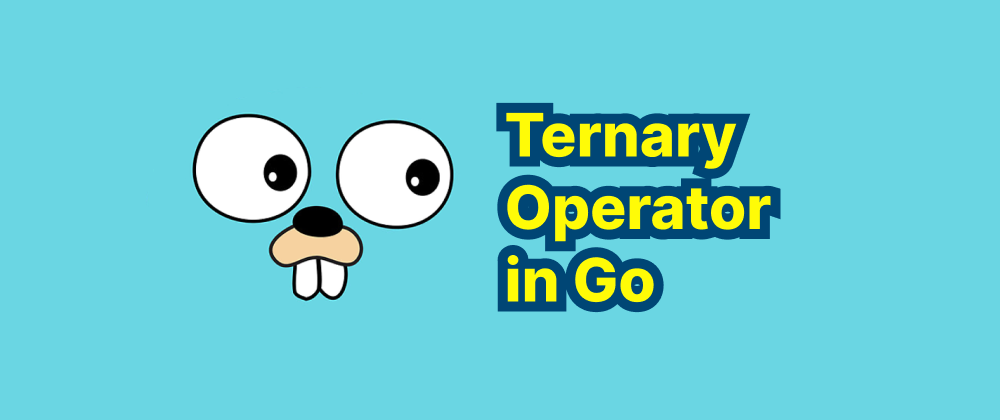
Key Takeaways
- Go does not support the ternary operator to maintain code clarity and simplicity.
if-else
statements are the idiomatic way to handle conditional assignments in Go.- Generics allow for helper functions that mimic ternary behavior concisely.
In many programming languages, the ternary operator (? :
) offers a concise way to perform conditional assignments. However, Go (Golang) intentionally omits this operator to promote code clarity and simplicity. This article explores idiomatic approaches in Go to achieve similar functionality.
The Ternary Operator in Other Languages
In languages like C or JavaScript, the ternary operator allows for compact conditional expressions:
int result = (condition) ? value_if_true : value_if_false;
This single line assigns value_if_true
to result
if condition
is true; otherwise, it assigns value_if_false
.
Go's Approach: If-Else Statements
Go emphasizes readability and explicitness. Instead of a ternary operator, Go utilizes straightforward if-else
statements:
package main import "fmt" func main() { condition := true var result string if condition { result = "It's true!" } else { result = "It's false!" } fmt.Println(result) }
Here, result
is assigned based on the evaluation of condition
. While more verbose than the ternary operator, this approach enhances code clarity.
Using Helper Functions
For scenarios requiring inline conditional evaluations, defining helper functions can be beneficial. With the introduction of generics in Go 1.18, such functions have become more versatile:
package main import "fmt" // Ternary function mimics the ternary operator func Ternary[T any](condition bool, trueVal, falseVal T) T { if condition { return trueVal } return falseVal } func main() { condition := false result := Ternary(condition, "Yes", "No") fmt.Println(result) // Outputs: No }
The Ternary
function evaluates condition
and returns either trueVal
or falseVal
accordingly. This method offers a concise alternative while maintaining readability.
Conclusion
While Go lacks a built-in ternary operator, its design philosophy encourages explicit and clear code through if-else
statements or helper functions. These practices ensure that Go code remains maintainable and understandable.
FAQs
Go prioritizes readability and explicitness over compact syntax.
Use an if-else
statement or a helper function with generics.
No significant concerns, but if-else
statements are the most direct approach.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ