Understanding How to Close Channels in Golang
James Reed
Infrastructure Engineer · Leapcell
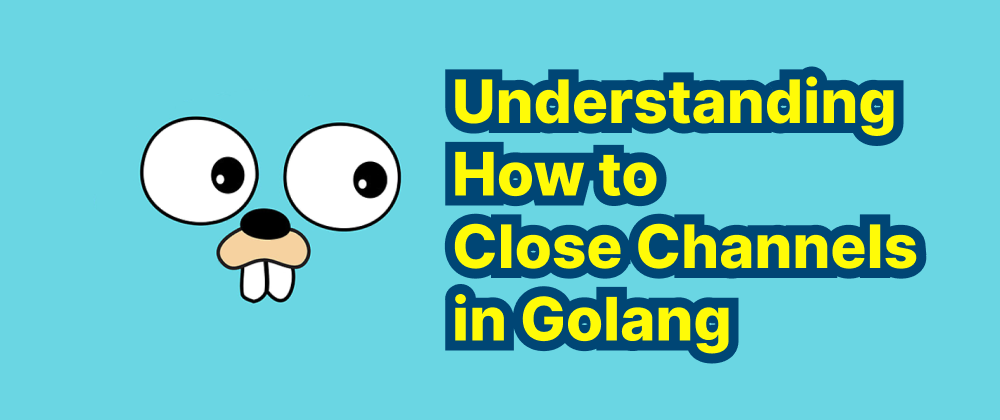
Key Takeaways
- Only the sender should close a channel to signal completion of data transmission.
- Closing a channel prevents further sends but still allows receiving remaining values.
- Improper closing (e.g., multiple closures or sending to a closed channel) causes runtime panics.
Golang's concurrency model is built around goroutines and channels, which allow safe communication between concurrent processes. However, properly closing channels is crucial to avoid resource leaks and unexpected behavior. This article explores how and when to close a channel in Go, along with best practices.
Why Close a Channel?
Closing a channel in Golang signals to receivers that no more values will be sent on the channel. This is particularly useful in scenarios where multiple goroutines are consuming data and need to know when to stop processing.
However, it is important to note that closing a channel is only necessary in specific cases. If a channel is only used within a function and all goroutines that use it terminate together, there is no need to explicitly close it.
How to Close a Channel
In Go, you can close a channel using the built-in close()
function. However, only the sender should close the channel—receivers should never attempt to close it.
Here is a simple example:
package main import "fmt" func main() { ch := make(chan int) go func() { for i := 0; i < 5; i++ { ch <- i } close(ch) // Closing the channel after sending data }() for val := range ch { fmt.Println(val) } }
Checking If a Channel Is Closed
When receiving from a channel, Go provides a way to check if a channel has been closed. This can be done using the second value returned by the receive operation:
value, ok := <-ch if !ok { fmt.Println("Channel is closed") }
Here, ok
will be false
if the channel is closed and drained of all values.
Common Mistakes When Closing Channels
- Closing a channel multiple times: Once a channel is closed, any attempt to close it again will result in a panic.
- Sending on a closed channel: Writing to a closed channel causes a panic, so ensure the sender does not send values after closing.
- Closing a channel from the receiver side: It is the sender's responsibility to close the channel. Receivers should only read and not manage the channel’s lifecycle.
When Not to Close a Channel
- When a channel is used within a function and all goroutines complete together.
- If the channel is still needed by other parts of the program.
- When using an unbuffered channel for a request-response model.
Conclusion
Closing channels in Golang is an important practice to indicate completion of data transmission. While it helps prevent goroutines from waiting indefinitely, it should be done carefully to avoid runtime errors. By following best practices, you can ensure safe and efficient channel usage in your concurrent Go applications.
FAQs
It results in a panic, as Go does not allow closing an already closed channel.
No, only the sender should close a channel to avoid unexpected behavior.
By using the second return value from a receive operation: value, ok := <-ch
.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ