Efficient String Concatenation in Go
James Reed
Infrastructure Engineer · Leapcell
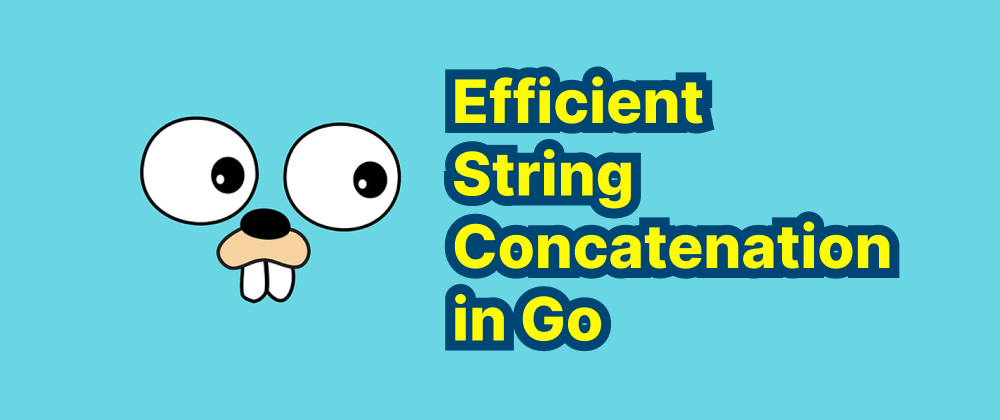
Key Takeaways
- The
+
operator is simple but inefficient for large-scale concatenation. strings.Builder
andbytes.Buffer
offer the best performance for frequent concatenation.strings.Join
is optimal for merging multiple strings in a slice.
In Go, string concatenation can be achieved through various methods, each with its own performance characteristics. Understanding these methods is essential for writing efficient code.
1. Using the +
Operator
The simplest way to concatenate strings in Go is by using the +
operator. This method is straightforward but may not be the most efficient for large or numerous concatenations, as strings in Go are immutable, leading to the creation of new strings with each concatenation.
package main import "fmt" func main() { s1 := "Hello" s2 := "World" result := s1 + " " + s2 fmt.Println(result) }
Output:
Hello World
2. Using fmt.Sprintf
The fmt.Sprintf
function allows for formatted string concatenation. While versatile, it is generally slower than other methods due to its formatting capabilities.
package main import "fmt" func main() { s1 := "Hello" s2 := "World" result := fmt.Sprintf("%s %s", s1, s2) fmt.Println(result) }
Output:
Hello World
3. Using strings.Join
The strings.Join
function concatenates a slice of strings into a single string. This method is efficient and particularly useful when dealing with multiple strings.
package main import ( "fmt" "strings" ) func main() { parts := []string{"Hello", "World"} result := strings.Join(parts, " ") fmt.Println(result) }
Output:
Hello World
4. Using bytes.Buffer
The bytes.Buffer
type provides a buffer for constructing strings efficiently, especially when concatenating a large number of strings. It minimizes memory allocations by writing to an internal buffer.
package main import ( "bytes" "fmt" ) func main() { var buffer bytes.Buffer buffer.WriteString("Hello") buffer.WriteString(" ") buffer.WriteString("World") result := buffer.String() fmt.Println(result) }
Output:
Hello World
5. Using strings.Builder
Introduced in Go 1.10, strings.Builder
is designed for efficient string concatenation. It is generally more performant than bytes.Buffer
for string operations, as it avoids some of the overhead associated with byte slices.
package main import ( "fmt" "strings" ) func main() { var builder strings.Builder builder.WriteString("Hello") builder.WriteString(" ") builder.WriteString("World") result := builder.String() fmt.Println(result) }
Output:
Hello World
Performance Considerations
The performance of each method varies based on the context:
-
+
Operator: Suitable for concatenating a small number of strings. However, it can lead to performance issues when used extensively in loops due to repeated memory allocations. -
fmt.Sprintf
: Offers flexibility with formatting but is generally slower and incurs more memory allocations. -
strings.Join
: Efficient for concatenating multiple strings, especially when they are stored in a slice. -
bytes.Buffer
: Provides efficient string concatenation with fewer memory allocations, suitable for scenarios involving numerous concatenations. -
strings.Builder
: Recommended for high-performance string concatenation, offering better efficiency and lower memory usage compared to other methods.
In conclusion, for optimal performance in Go, it is advisable to use strings.Builder
or bytes.Buffer
when dealing with numerous or large string concatenations. For a small number of concatenations, the +
operator or strings.Join
can be convenient and sufficiently efficient.
FAQs
Go strings are immutable, causing excessive memory allocation when using +
repeatedly.
Use strings.Builder
for string operations as it is optimized for strings and avoids unnecessary overhead.
It is flexible but generally slower due to additional formatting overhead.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ