How to Print the Type of a Variable in Go
Grace Collins
Solutions Engineer · Leapcell
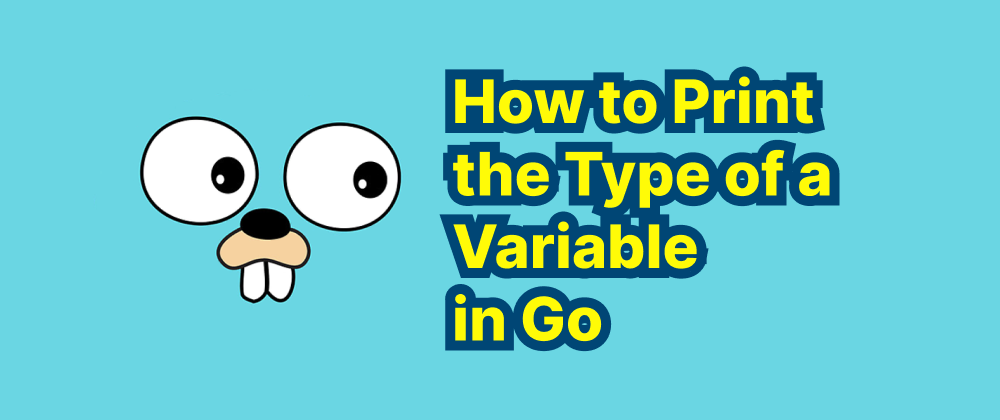
Key Takeaways
- Use
fmt.Printf("%T", variable)
for a simple way to print variable types. - The
reflect.TypeOf(variable)
function provides detailed type introspection. - Type switches allow dynamic handling of different variable types.
In Go, understanding the type of a variable during runtime can be essential for debugging and reflective operations. This article explores various methods to print or determine the type of a variable in Go.
Using fmt.Printf
with %T
Verb
The simplest way to print the type of a variable is by using the fmt.Printf
function with the %T
verb. This verb outputs the Go-syntax representation of the type of the value.
Example:
package main import "fmt" func main() { var ( booleanVar bool = true stringVar string = "Hello, Go!" intVar int = 42 floatVar float64 = 3.14 sliceVar []string = []string{"foo", "bar", "baz"} ) fmt.Printf("Type of booleanVar: %T\n", booleanVar) fmt.Printf("Type of stringVar: %T\n", stringVar) fmt.Printf("Type of intVar: %T\n", intVar) fmt.Printf("Type of floatVar: %T\n", floatVar) fmt.Printf("Type of sliceVar: %T\n", sliceVar) }
Output:
Type of booleanVar: bool
Type of stringVar: string
Type of intVar: int
Type of floatVar: float64
Type of sliceVar: []string
In this example, %T
retrieves and prints the type of each variable. This method is straightforward and does not require importing additional packages beyond fmt
.
Using the reflect
Package
For more advanced type introspection, Go provides the reflect
package, which allows inspection of variable types at runtime.
Example:
package main import ( "fmt" "reflect" ) func main() { var ( booleanVar bool = true stringVar string = "Hello, Go!" intVar int = 42 floatVar float64 = 3.14 sliceVar []string = []string{"foo", "bar", "baz"} ) fmt.Println("Type of booleanVar:", reflect.TypeOf(booleanVar)) fmt.Println("Type of stringVar:", reflect.TypeOf(stringVar)) fmt.Println("Type of intVar:", reflect.TypeOf(intVar)) fmt.Println("Type of floatVar:", reflect.TypeOf(floatVar)) fmt.Println("Type of sliceVar:", reflect.TypeOf(sliceVar)) }
Output:
Type of booleanVar: bool
Type of stringVar: string
Type of intVar: int
Type of floatVar: float64
Type of sliceVar: []string
Here, reflect.TypeOf(variable)
returns the reflect.Type
of the variable, which implements the String()
method to provide the name of the type.
Using Type Switches
Another approach to determine the type of a variable is by using a type switch. This method is particularly useful when you need to execute different code paths based on the variable's type.
Example:
package main import "fmt" func printType(v interface{}) { switch v.(type) { case int: fmt.Println("Type is int") case float64: fmt.Println("Type is float64") case string: fmt.Println("Type is string") case bool: fmt.Println("Type is bool") default: fmt.Println("Unknown type") } } func main() { printType(42) printType(3.14) printType("Hello, Go!") printType(true) }
Output:
Type is int
Type is float64
Type is string
Type is bool
In this example, the printType
function uses a type switch to determine the type of the interface{} parameter v
and prints a corresponding message.
Conclusion
Printing or determining the type of a variable in Go can be achieved through various methods:
- Using
fmt.Printf
with the%T
verb for straightforward type printing. - Utilizing the
reflect
package for more detailed type introspection. - Implementing type switches to handle different types dynamically.
Each method serves different use cases, and understanding them enhances your ability to write flexible and robust Go programs.
FAQs
Use fmt.Printf("%T", variable)
.
Use reflect.TypeOf(variable)
when detailed runtime type introspection is needed.
Use a type switch inside a function with interface{}
as a parameter.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ