Iterating Over Maps in Go: Methods, Order, and Best Practices
Grace Collins
Solutions Engineer · Leapcell
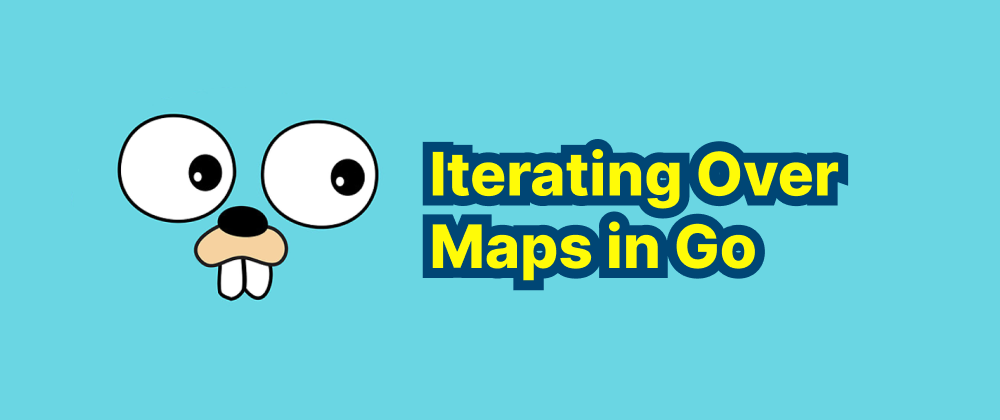
Key Takeaways
- Go's
for
-range
loop is the primary method for iterating over maps. - Map iteration order is not guaranteed and may vary between executions.
- Sorting keys is necessary for iterating over maps in a specific order.
In Go, maps are key-value data structures that provide efficient lookups, additions, and deletions. Iterating over a map is a common task, especially when you need to process or analyze all entries. This article explores how to iterate over maps in Go, highlighting key considerations and best practices.
Iterating Over a Map Using for
-range
Go provides a concise syntax for iterating over maps using the for
-range
loop. Here's how it works:
package main import "fmt" func main() { sampleMap := map[string]int{ "apple": 2, "banana": 5, "cherry": 7, } for key, value := range sampleMap { fmt.Printf("Key: %s, Value: %d\n", key, value) } }
In this example, sampleMap
is a map with string keys and integer values. The for
-range
loop retrieves each key-value pair, allowing you to process them as needed.
Order of Iteration
It's important to note that Go's map iteration order is not specified and can vary between executions. This means that each run of the program may produce a different order of key-value pairs. Therefore, if a specific order is required, you'll need to implement additional logic.
Iterating Over Keys in a Specific Order
To iterate over map keys in a specific order, such as ascending or descending, follow these steps:
- Extract the keys from the map and store them in a slice.
- Sort the slice using the
sort
package. - Iterate over the sorted keys to access the map values in the desired order.
Here's an example demonstrating this approach:
package main import ( "fmt" "sort" ) func main() { sampleMap := map[string]int{ "apple": 2, "banana": 5, "cherry": 7, } // Extract keys from the map keys := make([]string, 0, len(sampleMap)) for key := range sampleMap { keys = append(keys, key) } // Sort keys in ascending order sort.Strings(keys) // Iterate over sorted keys for _, key := range keys { fmt.Printf("Key: %s, Value: %d\n", key, sampleMap[key]) } }
In this code, we first extract the keys from sampleMap
and store them in the keys
slice. We then sort the keys in ascending order using sort.Strings
. Finally, we iterate over the sorted keys to access and process the corresponding values from the map.
Conclusion
Iterating over maps in Go is straightforward with the for
-range
loop. However, due to the unspecified iteration order, additional steps are necessary when a specific order is required. By extracting, sorting, and iterating over the keys, you can control the order in which you process map entries, ensuring your program behaves as intended.
FAQs
Go's map implementation optimizes performance, making iteration order unpredictable.
Extract the keys, sort them using sort.Strings
, and iterate over the sorted slice.
Modifying a map during iteration may cause runtime errors or unexpected behavior.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ