Understanding `fallthrough` in Go's `switch` Statements
Grace Collins
Solutions Engineer · Leapcell
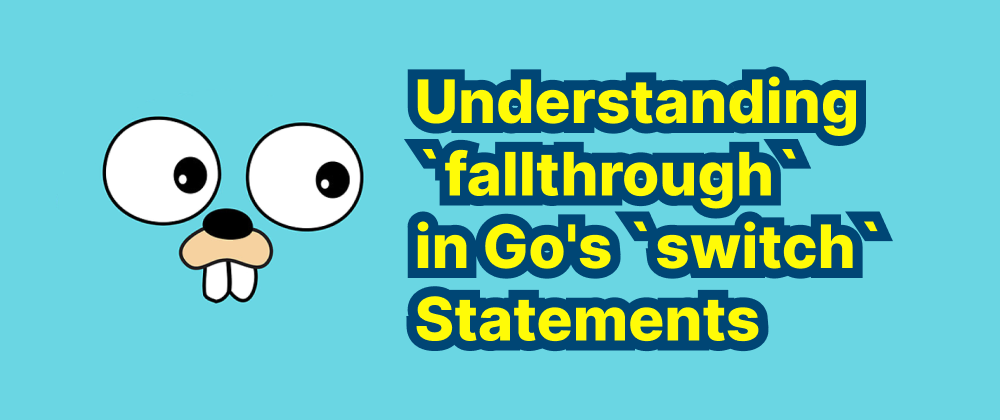
Key Takeaways
- Go's
switch
statements do not implicitly fall through; each case breaks automatically. - The
fallthrough
keyword allows execution to continue to the next case unconditionally. fallthrough
must be the last statement in a case block and cannot be used in the final case.
In Go, the switch
statement is a powerful control structure that allows for efficient branching based on the value of an expression. Unlike some other programming languages, Go's switch
statements automatically break after executing a matching case, preventing the fall-through behavior common in languages like C or JavaScript. However, there are scenarios where executing subsequent cases is desirable. This is where the fallthrough
keyword comes into play.
Default Behavior of switch
in Go
By default, when a case in a Go switch
statement matches, the code within that case executes, and the control flow exits the switch
block. There's no implicit fall-through to subsequent cases. Here's an example illustrating this behavior:
package main import ( "fmt" ) func main() { day := 3 switch day { case 1: fmt.Println("Monday") case 2: fmt.Println("Tuesday") case 3: fmt.Println("Wednesday") case 4: fmt.Println("Thursday") case 5: fmt.Println("Friday") default: fmt.Println("Weekend") } }
Output:
Wednesday
In this example, since day
is 3
, the output is "Wednesday". The program doesn't continue to execute the subsequent cases.
Introducing fallthrough
The fallthrough
keyword allows a case
to pass control to the next case, regardless of the evaluation of its expression. This can be useful in scenarios where multiple cases should execute sequentially. Here's how it works:
package main import ( "fmt" ) func main() { num := 4 switch num { case 1: fmt.Println("One") fallthrough case 2: fmt.Println("Two") fallthrough case 3: fmt.Println("Three") fallthrough case 4: fmt.Println("Four") fallthrough case 5: fmt.Println("Five") default: fmt.Println("Number out of range") } }
Output:
Four
Five
Number out of range
In this example, when num
is 4
, the program prints "Four" and, due to the fallthrough
statement, continues to execute the code in the next case, printing "Five" and then "Number out of range".
Important Considerations
-
Unconditional Transfer: The
fallthrough
keyword transfers control to the next case unconditionally. It doesn't evaluate the next case's expression. Therefore, use it cautiously to avoid unintended behavior. -
Placement Restrictions: The
fallthrough
statement must be the last statement in a case block. Attempting to place it elsewhere will result in a compile-time error. -
Final Case Limitation: You cannot use
fallthrough
in the last case of aswitch
statement, as there's no subsequent case to transfer control to.
Practical Example
Consider a scenario where you want to categorize a number based on its range:
package main import ( "fmt" ) func main() { num := 75 switch { case num < 50: fmt.Printf("%d is less than 50\n", num) case num < 100: fmt.Printf("%d is less than 100\n", num) fallthrough case num < 200: fmt.Printf("%d is less than 200\n", num) } }
Output:
75 is less than 100
75 is less than 200
Here, since num
is 75
, the first case (num < 50
) is false, so it's skipped. The second case (num < 100
) is true, so it prints "75 is less than 100" and then, due to the fallthrough
, proceeds to the next case, printing "75 is less than 200".
Conclusion
The fallthrough
keyword in Go provides a mechanism to explicitly allow control to pass to the subsequent case in a switch
statement. While this can be useful in certain scenarios, it's essential to use it judiciously to maintain code clarity and prevent unexpected behavior. Understanding its behavior and limitations ensures that your switch
statements function as intended.
FAQs
No, Go’s switch
automatically breaks after a matching case unless fallthrough
is explicitly used.
No, fallthrough
unconditionally transfers control to the next case.
It results in a compile-time error since there is no subsequent case to execute.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ