How to Read Rust Source Code Effectively: A Practical Approach
Olivia Novak
Dev Intern · Leapcell
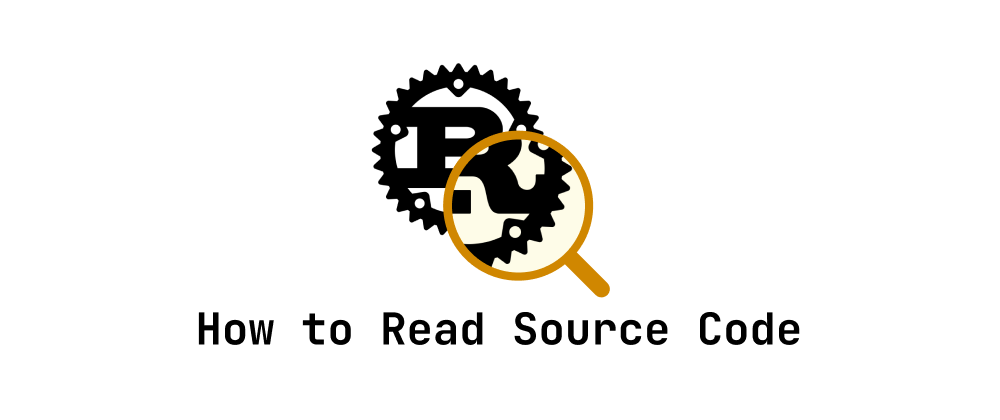
1. Start with Fundamental Modules
These modules are used in almost every Rust program, and understanding them is the foundation of learning the Rust standard library.
-
std::option::Option and std::result::Result
Option
is used to represent cases where a value may be absent, whileResult
is used for error handling. Understanding the implementation of these enums is crucial because they are core mechanisms in Rust for avoiding null references and capturing errors.- Learning Path: Review the documentation for these two modules, then analyze their specific implementations in the source code.
-
std::vec::Vec
Vec
is Rust’s dynamic array that automatically manages memory. By studyingVec
, you can understand how Rust implements safe memory management.- Learning Path: Read the
Vec
documentation and examine how its underlying implementation handles memory allocation and deallocation.
-
std::string::String
String
is a mutable UTF-8 encoded string. Gaining an in-depth understanding of its implementation helps you grasp efficient string operations.- Learning Path: Check the
String
documentation and study its relationship withVec<u8>
.
2. Understand Core System Interface Modules
The system interface modules in the Rust standard library interact with the operating system and low-level hardware.
-
std::fs and std::io
- The
fs
module provides filesystem operations, while theio
module encapsulates input/output operations. They are core modules for system programming. - Learning Path: Read the documentation for
fs
andio
, analyze how they encapsulate system calls, and study how they useResult
for error handling.
- The
-
std::thread and std::sync
- Rust’s concurrency model is based on threads and synchronization primitives (such as
Mutex
andArc
). Studying these modules helps you understand how Rust achieves thread safety and data sharing. - Learning Path: Read the
thread
andsync
documentation, and study the implementation of thread creation and data sharing.
- Rust’s concurrency model is based on threads and synchronization primitives (such as
3. Explore Memory Management and Smart Pointers
Memory management is one of Rust’s key features, and understanding smart pointers and memory management modules is crucial.
-
std::rc::Rc and std::sync::Arc
Rc
andArc
are reference-counting smart pointers used in single-threaded and multi-threaded environments, respectively. Studying their implementation helps you understand how Rust manages shared memory.- Learning Path: Read the
Rc
andArc
documentation, and analyze the underlying implementation of reference counting.
-
std::cell::RefCell and std::cell::Cell
RefCell
andCell
are smart pointers used for runtime borrow checking.- Learning Path: Review the documentation for
RefCell
andCell
and study their internal borrow checking mechanisms.
4. Further Study on Concurrency and Asynchronous Programming
- std::future and async/await
Future
is the core of Rust’s asynchronous programming. Understanding howasync/await
works helps you master Rust’s async programming model.- Learning Path: Read the
Future
documentation and analyze the source code to understand howasync/await
is transformed.
5. Master Error Handling and Logging Systems
-
std::error::Error and std::result::Result
- Error handling is an essential part of Rust. Understanding how the
Error
trait andResult
enum work together helps you grasp Rust’s error-handling mechanisms. - Learning Path: Review the
Error
andResult
documentation.
- Error handling is an essential part of Rust. Understanding how the
-
std::log and env_logger
- Rust’s logging system allows developers to configure log output flexibly.
- Learning Path: Read the
log
andenv_logger
documentation.
6. Safely Using unsafe
The unsafe
keyword enables Rust to perform low-level system programming, but it must be used cautiously to avoid undefined behavior.
- Learning Path: Understand the use cases and limitations of
unsafe
, and study its implementation in the standard library, such as the memory management ofVec<T>
.
7. Get Familiar with the Macro System
Rust’s macro system allows code generation at compile time. It can be learned from two aspects: declarative macros (macro_rules!
) and procedural macros.
-
Declarative Macros (
macro_rules!
)- Understand the syntax and use cases of declarative macros and analyze commonly used macros in the standard library, such as
vec!
andprintln!
.
- Understand the syntax and use cases of declarative macros and analyze commonly used macros in the standard library, such as
-
Procedural Macros
- Procedural macros are more powerful and allow manipulation of the abstract syntax tree (AST). Studying well-known procedural macro libraries (such as
serde
) helps deepen your understanding.
- Procedural macros are more powerful and allow manipulation of the abstract syntax tree (AST). Studying well-known procedural macro libraries (such as
We are Leapcell, your top choice for hosting Rust projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ