How to Generate Random Integers in Python
Daniel Hayes
Full-Stack Engineer · Leapcell
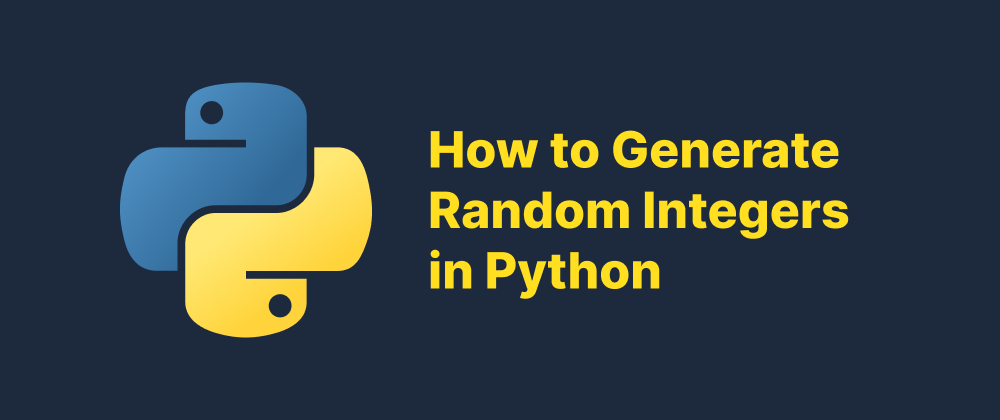
Key Takeaways
- Use
random.randint()
for general-purpose integer generation. - Use
secrets.randbelow()
for cryptographically secure random numbers. - Use
numpy.random.randint()
for fast, large-scale random integer arrays.
Generating random integers is a common task in programming, useful in a wide range of applications such as simulations, games, cryptography, and testing. Python provides several built-in methods to generate random integers efficiently and safely. In this article, we’ll explore the most commonly used approaches.
Using the random
Module
Python's standard library includes the random
module, which offers a suite of functions for generating pseudo-random numbers.
1. random.randint(a, b)
This function returns a random integer N such that a <= N <= b
.
import random num = random.randint(1, 10) print(num) # Output could be any integer from 1 to 10 (inclusive)
Note: Both endpoints are inclusive.
2. random.randrange(start, stop[, step])
randrange()
is similar to range()
, but it returns a randomly selected element from the range.
num = random.randrange(0, 100, 5) print(num) # Output could be 0, 5, 10, ..., up to less than 100
Using the secrets
Module (for Security)
If you need cryptographically secure random integers (e.g., for passwords, tokens, or authentication), use the secrets
module, which is also in the Python standard library (since Python 3.6).
import secrets num = secrets.randbelow(10) # Returns an int in the range [0, 10) print(num)
This is more secure than random.randint()
, but slightly slower.
Using numpy
for Large-Scale Generation
If you're working with data science or simulations and need to generate many random integers efficiently, numpy
provides fast vectorized tools:
import numpy as np arr = np.random.randint(0, 100, size=10) print(arr) # Outputs a NumPy array of 10 random integers between 0 and 99
numpy
is not part of the standard library and must be installed separately (pip install numpy
).
Conclusion
To summarize:
Method | Use Case | Inclusive Range | Secure? |
---|---|---|---|
random.randint(a, b) | General use | Yes | No |
random.randrange(a, b) | Random element from range | No (excludes b ) | No |
secrets.randbelow(n) | Secure random numbers | No (excludes n ) | Yes |
numpy.random.randint() | Efficient array generation | Yes | No |
Choose the method that best fits your needs, whether it’s for everyday scripting, secure applications, or large-scale numerical work.
FAQs
randint(a, b)
includes both a
and b
, while randrange(a, b)
excludes b
.
Use it when security is a concern, like for generating passwords or tokens.
Yes, NumPy must be installed separately using pip install numpy
.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ