Handling Command Line Arguments in Golang
Olivia Novak
Dev Intern · Leapcell
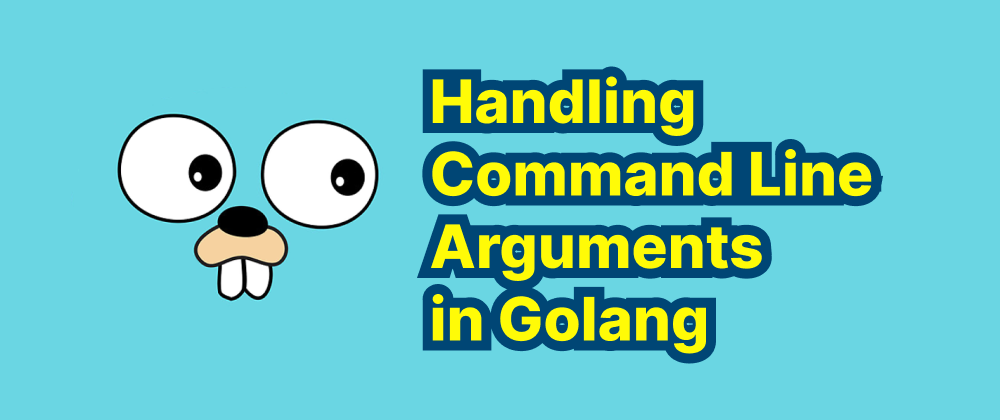
Key Takeaways
- Use
os.Args
for basic command line argument retrieval. - The
flag
package enables structured parsing of named flags. pflag
supports GNU-style flags for advanced CLI applications.
Command line arguments are an essential part of any CLI (Command Line Interface) application, allowing users to pass parameters when executing a program. Golang provides built-in support for handling these arguments through the os
and flag
packages. This article explores how to work with command line arguments in Golang, from basic usage to advanced flag parsing.
Accessing Command Line Arguments with os.Args
The simplest way to retrieve command line arguments in Golang is by using os.Args
. This slice contains all arguments passed to the program, with os.Args[0]
representing the executable name.
Example: Reading Raw Arguments
package main import ( "fmt" "os" ) func main() { args := os.Args fmt.Println("Arguments:", args) }
Example Execution:
$ go run main.go hello world Arguments: [/tmp/go-build1234/b001/exe/main hello world]
Here, os.Args[0]
is the program name, and the actual arguments start from index 1
.
Accessing Specific Arguments
if len(os.Args) > 1 { fmt.Println("First argument:", os.Args[1]) }
Parsing Flags with the flag
Package
While os.Args
is useful for simple argument retrieval, it lacks structured parsing capabilities. The flag
package provides a more robust way to handle command line options.
Example: Parsing Flags
package main import ( "flag" "fmt" ) func main() { name := flag.String("name", "Gopher", "Your name") age := flag.Int("age", 25, "Your age") verbose := flag.Bool("verbose", false, "Enable verbose output") flag.Parse() fmt.Println("Name:", *name) fmt.Println("Age:", *age) fmt.Println("Verbose Mode:", *verbose) }
Example Execution:
$ go run main.go -name Alice -age 30 -verbose Name: Alice Age: 30 Verbose Mode: true
Handling Positional Arguments
The flag
package also allows handling additional arguments after parsing flags using flag.Args()
.
Example: Parsing Positional Arguments
flag.Parse() positionalArgs := flag.Args() fmt.Println("Positional arguments:", positionalArgs)
Example Execution:
$ go run main.go -name Bob extra1 extra2 Positional arguments: [extra1 extra2]
Using pflag
for GNU-Style Flags
The standard flag
package does not support GNU-style flags (e.g., --long-flag
). For better compatibility, the pflag
package from github.com/spf13/pflag
can be used.
Example: Using pflag
package main import ( "fmt" "github.com/spf13/pflag" ) func main() { name := pflag.String("name", "Gopher", "Your name") pflag.Parse() fmt.Println("Name:", *name) }
Example Execution:
$ go run main.go --name Charlie Name: Charlie
Conclusion
Golang provides multiple ways to handle command line arguments:
os.Args
– Directly accesses raw arguments.flag
package – Provides structured parsing for named flags.flag.Args()
– Retrieves positional arguments.pflag
package – Offers GNU-style flag support.
For small scripts, os.Args
might be sufficient, while for complex CLI applications, flag
or pflag
should be preferred. By leveraging these tools, developers can create flexible and user-friendly command line applications in Golang.
FAQs
Use os.Args
to access raw command line arguments as a slice.
Use the flag
package to define and parse named flags.
Use the pflag
package from github.com/spf13/pflag
.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ