Understanding and Using the `append` Function in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
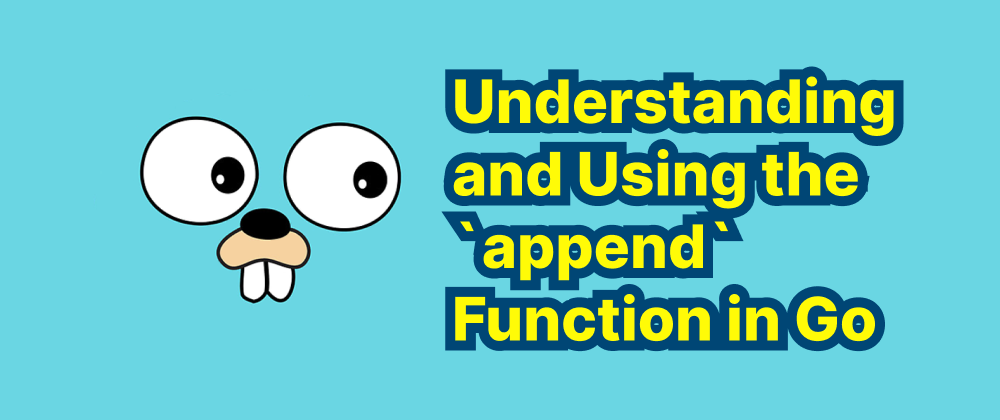
Key Takeaways
- The
append
function dynamically resizes slices and returns a new slice. - If capacity is insufficient,
append
allocates a new underlying array. - Always reassign the result of
append
to retain changes.
In Go, slices are a versatile and powerful data structure that provide a more flexible alternative to arrays. One of the most common operations performed on slices is appending elements. This article delves into the mechanics of the append
function, its usage, and important considerations to keep in mind.
Basics of Slices
Before exploring the append
function, it's essential to understand what slices are. A slice in Go is a descriptor for a contiguous segment of an underlying array and provides dynamic sizing. Unlike arrays, slices are not fixed in length, allowing for more flexible and powerful data structures.
Here's how you can declare and initialize a slice:
var numbers []int // Declaring a slice of integers
At this point, numbers
is a nil slice with a length and capacity of 0. You can initialize it with values as follows:
numbers = []int{1, 2, 3, 4, 5} // Initializing the slice with values
Using the append
Function
The append
function is built into Go and is used to add elements to the end of a slice. Its signature is:
func append(slice []T, elements ...T) []T
slice
is the original slice to which elements will be added.elements
are the new elements to append to the slice.- The function returns a new slice that includes the original elements followed by the appended ones.
Appending a Single Element
To append a single element to a slice:
numbers := []int{1, 2, 3} numbers = append(numbers, 4) fmt.Println(numbers) // Output: [1 2 3 4]
Appending Multiple Elements
You can also append multiple elements at once:
numbers := []int{1, 2, 3} numbers = append(numbers, 4, 5, 6) fmt.Println(numbers) // Output: [1 2 3 4 5 6]
Alternatively, you can append another slice using the ...
operator:
numbers := []int{1, 2, 3} moreNumbers := []int{4, 5, 6} numbers = append(numbers, moreNumbers...) fmt.Println(numbers) // Output: [1 2 3 4 5 6]
Important Considerations
-
Capacity and Allocation: If the slice has sufficient capacity, the
append
function adds the new elements without allocating a new underlying array. If the capacity is insufficient,append
allocates a new array, copies the existing elements, and then adds the new ones. This behavior is crucial to understand, especially when working with large slices or performance-critical applications. -
Reassigning the Slice: The
append
function returns the updated slice. It's essential to reassign the result back to the original slice variable or another variable. Failing to do so means the original slice remains unchanged.numbers := []int{1, 2, 3} append(numbers, 4) fmt.Println(numbers) // Output: [1 2 3]
In the above example, since the result of
append
is not assigned back,numbers
remains unchanged. -
Appending to Nil Slices: You can append elements to a nil slice. Go handles this gracefully by creating a new underlying array.
var numbers []int numbers = append(numbers, 1, 2, 3) fmt.Println(numbers) // Output: [1 2 3]
-
Appending Empty Slices: Appending an empty slice (or no elements) to another slice doesn't change the original slice.
numbers := []int{1, 2, 3} emptySlice := []int{} numbers = append(numbers, emptySlice...) fmt.Println(numbers) // Output: [1 2 3]
Conclusion
The append
function is a fundamental tool in Go for working with slices, offering flexibility and dynamic resizing capabilities. Understanding its behavior, especially regarding capacity and memory allocation, is vital for writing efficient and effective Go code. By mastering slices and the append
function, you can harness the full potential of Go's powerful data structures.
FAQs
A new slice with an allocated array is created automatically.
No, it returns a new slice that must be reassigned.
Yes, either by listing elements directly or using the ...
operator for another slice.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ