Understanding the `select` Statement in Go
Min-jun Kim
Dev Intern · Leapcell
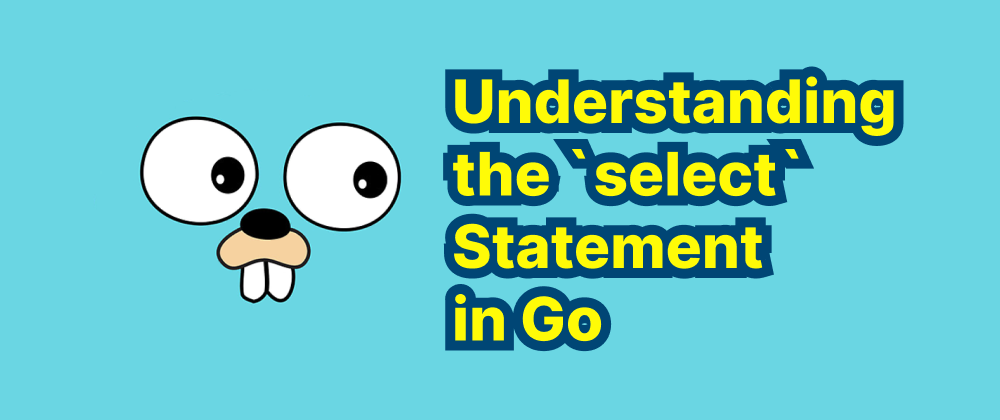
Key Takeaways
- The
select
statement enables goroutines to wait on multiple channel operations concurrently. - It randomly selects a ready case to execute, ensuring no specific channel is favored.
select
can be used to implement timeouts, improving responsiveness in concurrent programs.
The select
statement in Go is a powerful control structure that allows a goroutine to wait on multiple communication operations, enabling efficient handling of concurrent tasks. It is specifically designed to work with channels, facilitating synchronization and communication between goroutines.
Syntax and Basic Usage
The syntax of the select
statement is similar to that of a switch
statement, but it operates on channel operations:
select { case <-ch1: // Code to execute when a message is received from ch1 case ch2 <- value: // Code to execute when a value is sent to ch2 default: // Code to execute when none of the above cases are ready }
In this structure, each case
represents a communication operation: receiving from or sending to a channel. The select
statement blocks until at least one of its cases can proceed. If multiple cases are ready, one is chosen at random. The default
case, if present, executes immediately when no other case is ready, preventing the select
from blocking.
Practical Example
Consider a scenario where two goroutines perform tasks concurrently, and we want to handle their results as they become available:
package main import ( "fmt" "time" ) func task1(ch chan string) { time.Sleep(2 * time.Second) ch <- "Result from task 1" } func task2(ch chan string) { time.Sleep(1 * time.Second) ch <- "Result from task 2" } func main() { ch1 := make(chan string) ch2 := make(chan string) go task1(ch1) go task2(ch2) for i := 0; i < 2; i++ { select { case res := <-ch1: fmt.Println(res) case res := <-ch2: fmt.Println(res) } } }
In this example, two tasks are executed concurrently, each sending a result through its respective channel after a delay. The select
statement in the main
function waits for messages from either channel and prints them as they arrive. Since task2
has a shorter sleep duration, its result is received and printed first.
Randomness in Case Selection
When multiple cases in a select
statement are ready, Go selects one at random to proceed. This behavior ensures that no specific case is favored over others, preventing potential starvation of any particular channel operation.
Implementing Timeouts
The select
statement can be used to implement timeouts for channel operations. By utilizing Go's time.After
function, we can specify a duration to wait before proceeding with an alternative action:
select { case res := <-ch: fmt.Println("Received:", res) case <-time.After(3 * time.Second): fmt.Println("Timeout: no message received") }
In this snippet, the select
waits for a message from ch
. If no message is received within 3 seconds, the timeout case executes, indicating that the operation has timed out.
Key Differences Between select
and switch
While select
and switch
statements in Go share syntactical similarities, they differ in several important aspects:
-
Scope of Use:
select
is exclusively used for channel operations (send/receive), whereasswitch
is more general-purpose and can evaluate various types of expressions. -
Execution Order: In a
switch
statement, cases are evaluated sequentially from top to bottom. In contrast,select
does not have a predetermined order; if multiple cases are ready, one is chosen at random. -
Blocking Behavior: A
switch
statement does not block and proceeds to the matching case or thedefault
case. Aselect
statement, however, blocks until at least one of its cases can proceed, unless adefault
case is provided.
Conclusion
The select
statement is a fundamental construct in Go for managing multiple channel operations within goroutines. It provides a mechanism to handle concurrent tasks efficiently, enabling developers to write responsive and robust programs. Understanding its syntax, behavior, and differences from similar control structures is essential for effective concurrent programming in Go.
FAQs
No, select
is specifically designed for channel communication and cannot be used with other types of operations.
Go randomly selects one of the ready cases to execute, preventing bias toward any specific channel.
You can use a default
case or incorporate time.After
to introduce a timeout.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ