How to Delete from a Map in Golang
Daniel Hayes
Full-Stack Engineer · Leapcell
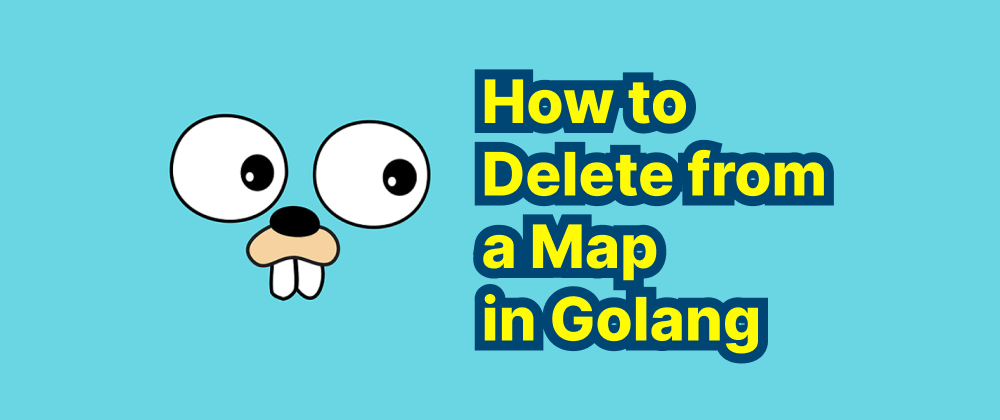
Key Takeaways
- Use the built-in
delete
function to remove keys from a map. - Deleting a non-existent key is safe and does not cause an error.
- Reinitializing the map is the most efficient way to clear all elements.
Maps are one of the most commonly used data structures in Golang, allowing developers to store key-value pairs efficiently. However, managing map data often requires deleting elements when they are no longer needed. In this article, we will explore how to delete an element from a map in Golang, discuss edge cases, and examine best practices.
Using the delete
Function
Golang provides a built-in function, delete
, to remove an entry from a map. The syntax is simple:
delete(mapName, key)
Here’s an example demonstrating its usage:
package main import "fmt" func main() { // Define a map with string keys and integer values userAges := map[string]int{ "Alice": 25, "Bob": 30, "Charlie": 35, } fmt.Println("Before deletion:", userAges) // Delete the entry with key "Bob" delete(userAges, "Bob") fmt.Println("After deletion:", userAges) }
Explanation
- The
delete
function takes two arguments:- The map from which the element should be removed.
- The key of the element to delete.
- If the key exists, the key-value pair is removed.
- If the key does not exist, the function does nothing (no error is thrown).
Handling Non-Existent Keys
One of the advantages of using delete
is that it does not panic when attempting to remove a non-existent key. This allows safe deletions without explicit checks.
delete(userAges, "David") // No error, even though "David" is not in the map
Checking Before Deleting
Although delete
is safe to use without checking, sometimes you may want to verify whether a key exists before removing it. This can be done using the comma-ok idiom:
if _, exists := userAges["Charlie"]; exists { delete(userAges, "Charlie") fmt.Println("Charlie was deleted.") } else { fmt.Println("Charlie does not exist in the map.") }
Deleting All Elements from a Map
If you need to remove all elements from a map, you have two approaches:
1. Reinitialize the Map
The simplest and most efficient way to clear a map is to reinitialize it:
userAges = make(map[string]int) // Creates a new empty map
2. Iterate and Delete Keys
If you want to explicitly delete each key (useful when modifying a shared reference), you can loop through the map:
for key := range userAges { delete(userAges, key) }
Performance Considerations
- The
delete
function has O(1) time complexity on average, making it efficient for removing elements. - Since maps are implemented as hash tables, deleting an element does not cause memory to shrink. If memory usage is a concern, consider reinitializing the map when all elements are deleted.
Conclusion
Deleting elements from a map in Golang is straightforward with the built-in delete
function. It is safe to use without explicit checks and has minimal performance overhead. Whether deleting a single key, checking for existence before deletion, or clearing an entire map, understanding how delete
works allows for effective map management in Go programs.
FAQs
Nothing happens; Golang's delete
function handles it gracefully without errors.
Use the comma-ok idiom: if _, exists := map[key]; exists { delete(map, key) }
.
Reinitialize the map using make(map[KeyType]ValueType)
for efficiency.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ