Exploring Graphics Libraries in Go
Grace Collins
Solutions Engineer · Leapcell
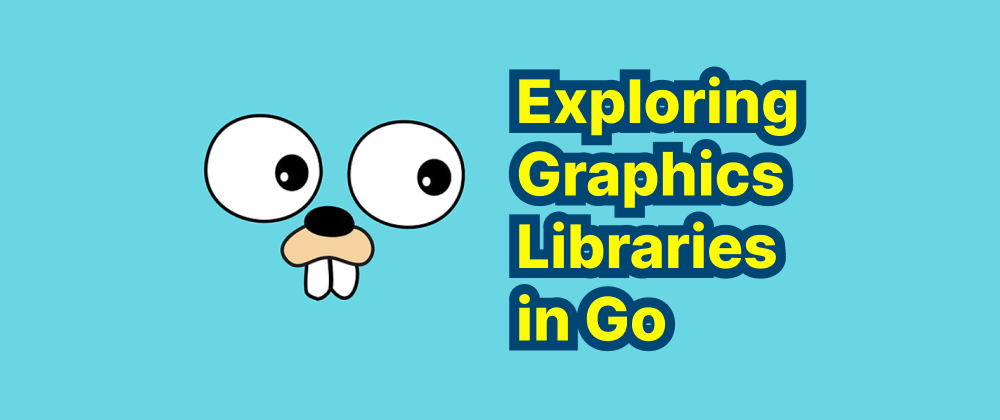
Key Takeaways
- Go's standard
image
package supports basic image manipulations like encoding and decoding. - Libraries like
gg
,gonum/plot
, andgo-echarts
offer powerful graphics and data visualization capabilities. Fyne
enables cross-platform GUI development with custom graphics support.
Go, often referred to as Golang, is renowned for its simplicity and efficiency in systems programming. However, its capabilities extend into the realm of graphics and visualization, thanks to a variety of libraries developed by the community. This article delves into some of the prominent graphics libraries available in Go, highlighting their features and use cases.
1. Standard Library: image
Go's standard library includes the image
package, which provides fundamental tools for image manipulation. It supports basic operations such as image creation, decoding, and encoding for formats like PNG, JPEG, and GIF. While it offers essential functionalities, for more advanced graphics rendering, developers often turn to external libraries.
2. gg
: 2D Rendering Made Simple
The gg
library is designed for 2D rendering in pure Go. It offers a straightforward API for drawing shapes, text, and images. Here's a simple example of creating a circle using gg
:
package main import "github.com/fogleman/gg" func main() { dc := gg.NewContext(1000, 1000) dc.DrawCircle(500, 500, 400) dc.SetRGB(0, 0, 0) dc.Fill() dc.SavePNG("circle.png") }
This code initializes a 1000x1000 canvas, draws a black circle at the center, and saves it as a PNG file.
3. gonum/plot
: Data Visualization
For those interested in data visualization, gonum/plot
is a powerful library that facilitates the creation of various plots and charts. It supports line plots, scatter plots, bar charts, and more. Here's an example of generating a simple line plot:
package main import ( "gonum.org/v1/plot" "gonum.org/v1/plot/plotter" "gonum.org/v1/plot/vg" "math/rand" ) func main() { p, err := plot.New() if err != nil { panic(err) } p.Title.Text = "Random Points" p.X.Label.Text = "X" p.Y.Label.Text = "Y" pts := make(plotter.XYs, 15) for i := range pts { pts[i].X = float64(i) pts[i].Y = rand.Float64() } line, err := plotter.NewLine(pts) if err != nil { panic(err) } p.Add(line) if err := p.Save(4*vg.Inch, 4*vg.Inch, "line.png"); err != nil { panic(err) } }
This script creates a line plot of random points and saves it as a PNG file.
4. go-chart
: Simple Charting
go-chart
is a basic charting library that allows for the creation of simple charts with minimal effort. It supports time series and continuous line charts, among others. An example of generating a line chart:
package main import ( "github.com/wcharczuk/go-chart/v2" "os" ) func main() { graph := chart.Chart{ Series: []chart.Series{ chart.ContinuousSeries{ XValues: []float64{1, 2, 3, 4, 5}, YValues: []float64{1, 2, 3, 4, 5}, }, }, } f, _ := os.Create("simple_chart.png") defer f.Close() graph.Render(chart.PNG, f) }
This code produces a simple line chart with a direct correlation between X and Y values.
5. go-echarts
: Interactive Visualizations
Inspired by Apache ECharts, go-echarts
enables the creation of interactive and highly customizable charts. It leverages the power of ECharts to provide a rich set of visualization options. Here's how you can create a basic line chart:
package main import ( "github.com/go-echarts/go-echarts/v2/charts" "github.com/go-echarts/go-echarts/v2/opts" "os" ) func main() { line := charts.NewLine() line.SetGlobalOptions(charts.WithTitleOpts(opts.Title{Title: "Basic Line Example"})) line.SetXAxis([]string{"Jan", "Feb", "Mar", "Apr", "May"}). AddSeries("Category A", []opts.LineData{ {Value: 10}, {Value: 20}, {Value: 30}, {Value: 40}, {Value: 50}, }) f, _ := os.Create("line.html") defer f.Close() line.Render(f) }
This script generates an interactive line chart and saves it as an HTML file.
6. Fyne
: Cross-Platform GUI
While not exclusively a graphics library, Fyne
is a cross-platform GUI toolkit for Go that supports the creation of graphical applications. It provides a range of widgets and supports drawing operations, making it suitable for applications that require custom graphics alongside standard GUI components.
Conclusion
Go's ecosystem offers a variety of libraries tailored for graphics and visualization needs. Whether you're aiming to perform basic image manipulations, render 2D graphics, visualize data, or develop full-fledged graphical applications, there's likely a Go library that fits your requirements. Exploring these libraries can significantly enhance the graphical capabilities of your Go projects.
FAQs
The gg
library offers an easy API for 2D drawing in pure Go.
go-echarts
provides interactive and customizable charting options.
Yes, the Fyne
library supports cross-platform GUI application development.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ