Understanding Global Variables in Go
James Reed
Infrastructure Engineer Β· Leapcell
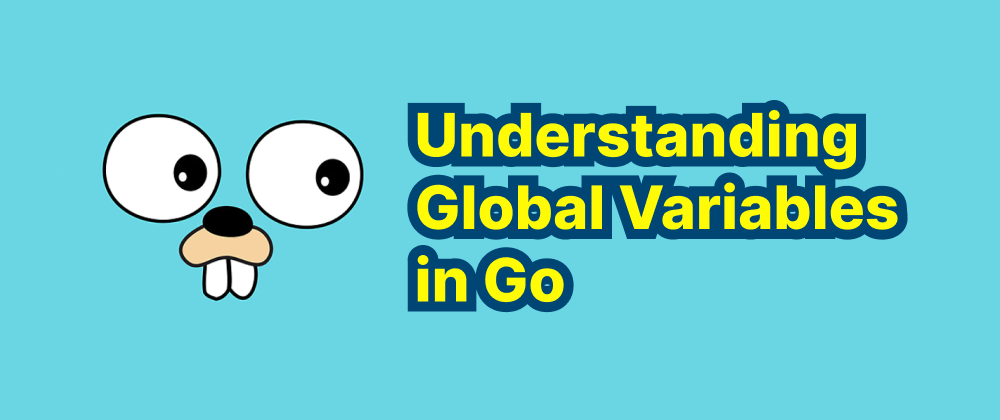
Key Takeaways
- Global variables provide easy data sharing across functions but reduce modularity.
- Overuse of global variables can lead to testing challenges and concurrency issues.
- Proper encapsulation and synchronization help mitigate risks of global variables.
In Go, global variables are those declared outside of any function, making them accessible throughout the entire package. While they can be useful, their usage requires careful consideration to maintain code clarity and prevent potential issues.
Declaring Global Variables
To declare a global variable in Go, place the var
keyword outside of any function:
package main import "fmt" var globalVar = "I am global" func main() { fmt.Println(globalVar) localFunction() } func localFunction() { fmt.Println(globalVar) }
In this example, globalVar
is accessible both in main
and localFunction
.
Pros and Cons of Global Variables
Pros
- Convenience: They allow for easy sharing of data across multiple functions without the need for parameter passing.
- State Management: Useful for maintaining state that is relevant across various parts of the application.
Cons
- Tight Coupling: Functions become dependent on global state, reducing modularity.
- Testing Challenges: Global variables can make unit testing difficult due to shared state.
- Concurrency Issues: Without proper synchronization, global variables can lead to race conditions in concurrent programs.
Best Practices
- Limit Usage: Use global variables sparingly. Prefer passing variables as function parameters to promote modularity.
- Encapsulation: If a global variable is necessary, consider encapsulating it within a struct or using getter and setter functions to control access.
- Synchronization: For concurrent applications, protect global variables with synchronization primitives like mutexes to prevent race conditions.
Conclusion
While global variables in Go offer a straightforward way to share data across functions, they come with trade-offs. Judicious use, along with adherence to best practices, ensures that your Go applications remain maintainable, testable, and free from concurrency issues.
FAQs
Overuse can lead to tight coupling, testing difficulties, and concurrency issues.
Use synchronization mechanisms like mutexes to ensure thread safety.
Passing variables as function parameters promotes modularity and maintainability.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage β no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead β just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ