Understanding Buffered Channels in Go
Emily Parker
Product Engineer · Leapcell
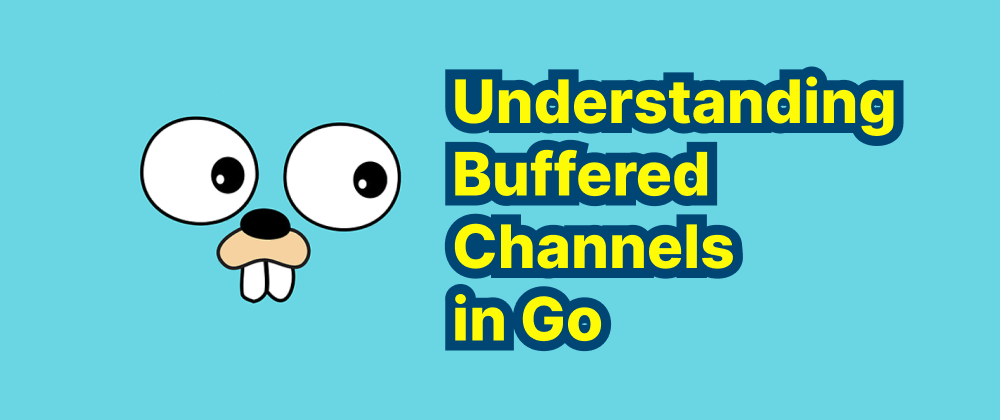
Key Takeaways
- Buffered channels in Go allow asynchronous communication by storing messages without immediate receipt.
- They improve performance by reducing blocking between sender and receiver goroutines.
- Proper buffer sizing is crucial to prevent deadlocks and optimize resource usage.
Go, commonly referred to as Golang, is a statically typed, compiled programming language designed for simplicity and efficiency. One of its standout features is the built-in support for concurrent programming through goroutines and channels. Channels facilitate communication between goroutines, and they come in two primary types: unbuffered and buffered. This article delves into buffered channels, exploring their characteristics, usage, and benefits.
What Are Buffered Channels?
In Go, a channel is a conduit through which goroutines can communicate by sending and receiving values of a specified type. By default, channels are unbuffered, meaning that a send operation will block until another goroutine is ready to receive the value, and vice versa. Buffered channels, on the other hand, have a capacity to store a predetermined number of values without requiring an immediate corresponding receive operation. This buffering allows for more flexible communication patterns between goroutines.
Creating Buffered Channels
Buffered channels are created using the make
function, where the second argument specifies the buffer's capacity:
ch := make(chan int, 3)
In this example, ch
is a buffered channel with a capacity to hold three int
values. This means that up to three values can be sent into the channel without blocking; the fourth send operation will block until a value is received from the channel, making space available.
Behavior of Buffered Channels
The primary distinction between buffered and unbuffered channels lies in their blocking behavior:
-
Sending to a Buffered Channel: A send operation will block only when the channel's buffer is full. If the buffer has available space, the value is placed into the buffer immediately, and the goroutine continues execution without waiting for a receiver.
-
Receiving from a Buffered Channel: A receive operation will block only when the channel's buffer is empty. If there are values in the buffer, the receive operation retrieves the next value, and the goroutine proceeds without waiting for a sender.
This behavior allows for decoupling the timing between the sender and receiver goroutines, enabling more asynchronous communication patterns.
Example of Buffered Channel Usage
Consider the following example demonstrating the use of a buffered channel:
package main import "fmt" func main() { // Create a buffered channel with a capacity of 2 messages := make(chan string, 2) // Send values into the channel messages <- "buffered" messages <- "channel" // Receive and print values from the channel fmt.Println(<-messages) fmt.Println(<-messages) }
Output:
buffered
channel
In this example, the messages
channel has a buffer capacity of 2. The two send operations do not block because the buffer has space to accommodate both values. The subsequent receive operations retrieve and print the values from the channel.
Advantages of Buffered Channels
Buffered channels offer several benefits:
-
Decoupling of Goroutines: They allow the sending and receiving goroutines to operate more independently. The sender can proceed without waiting for an immediate receiver, provided the buffer is not full, and the receiver can retrieve messages at its own pace as long as the buffer is not empty.
-
Improved Performance: By reducing the number of context switches between goroutines, buffered channels can enhance performance in scenarios where high-frequency communication is required. This is because goroutines are less likely to block and yield control, leading to more efficient execution.
-
Control Over Flow: Buffered channels provide a mechanism to control the flow of data between goroutines, acting as a queue that can help manage backpressure and prevent overwhelming a receiver with too many messages at once.
Considerations When Using Buffered Channels
While buffered channels offer flexibility, it's essential to use them judiciously:
-
Deadlocks: If a goroutine attempts to send a value to a full buffered channel and there is no receiving goroutine to consume it, the sending goroutine will block, potentially leading to a deadlock if not managed correctly. Similarly, receiving from an empty buffered channel will block until a value is available.
-
Buffer Size: Choosing an appropriate buffer size is crucial. A buffer that's too small may not provide the desired decoupling between goroutines, while a buffer that's too large could consume unnecessary memory resources.
-
Synchronization: While buffered channels can reduce the need for explicit synchronization mechanisms like mutexes, it's still important to ensure that the program's logic accounts for the asynchronous nature of goroutine communication to avoid race conditions and ensure data consistency.
Conclusion
Buffered channels are a powerful feature in Go that facilitate flexible and efficient communication between goroutines. By allowing a specified number of values to be stored without immediate receipt, they enable asynchronous interaction patterns and can lead to performance improvements in concurrent applications. However, developers must carefully consider buffer sizes and potential blocking behaviors to avoid pitfalls such as deadlocks. Understanding the nuances of buffered channels is essential for writing robust and efficient Go programs.
FAQs
Buffered channels can store multiple values without blocking, whereas unbuffered channels require a simultaneous sender and receiver.
Use a buffered channel when you need to decouple sender and receiver timing or improve concurrency performance.
If a sender writes to a full buffered channel without a receiver, or a receiver waits on an empty one, execution will block indefinitely.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ