Understanding Optional Arguments in Python
Daniel Hayes
Full-Stack Engineer · Leapcell
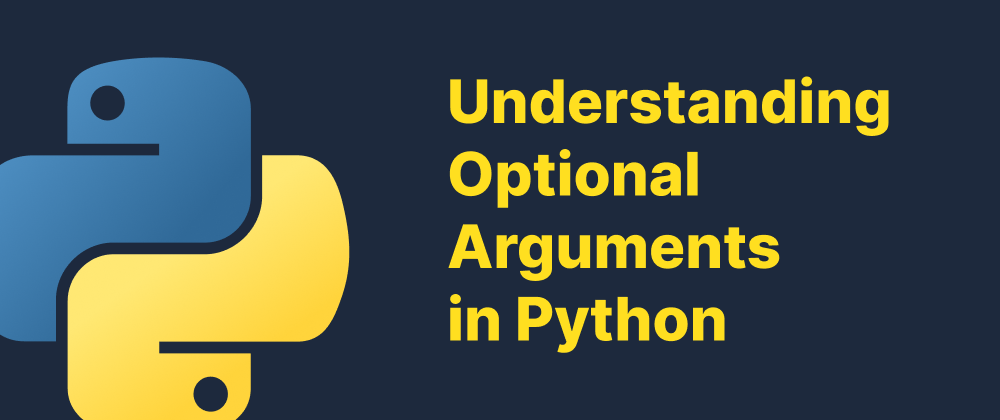
Key Takeaways
- Optional arguments provide flexibility through default parameter values.
*args
and**kwargs
allow variable-length optional arguments.- Avoid using mutable objects as default values to prevent bugs.
When writing functions in Python, flexibility is often a key concern. One powerful feature that helps achieve this is optional arguments. These allow function callers to provide fewer arguments than the number of parameters defined, making your code cleaner and more user-friendly.
In this article, we’ll explore what optional arguments are, how to use them effectively, and some common pitfalls to avoid.
What Are Optional Arguments?
Optional arguments are function parameters that are not required when the function is called. They are defined with default values in the function signature. If no value is passed for an optional argument, the default value is used.
Basic Syntax
def greet(name, greeting="Hello"): print(f"{greeting}, {name}!")
In the example above, greeting
is an optional argument. If the caller does not provide a value for it, "Hello"
will be used:
greet("Alice") # Output: Hello, Alice! greet("Bob", "Hi") # Output: Hi, Bob!
Types of Optional Arguments
1. Default Values
As shown above, you can set default values directly in the function signature.
def multiply(a, b=2): return a * b print(multiply(3)) # Output: 6 print(multiply(3, 4)) # Output: 12
2. *args
and **kwargs
For more dynamic optional arguments, Python supports variable-length arguments:
*args
: accepts any number of positional arguments as a tuple.**kwargs
: accepts any number of keyword arguments as a dictionary.
def report(*args, **kwargs): print("Positional:", args) print("Keyword:", kwargs) report(1, 2, 3, name="Alice", age=30) # Output: # Positional: (1, 2, 3) # Keyword: {'name': 'Alice', 'age': 30}
These are useful when you want to allow flexible numbers of inputs.
Keyword-Only Arguments
You can force certain optional arguments to be passed as keywords by placing them after a *
in the function signature:
def connect(host, port=80, *, timeout=30): print(f"Connecting to {host}:{port} with timeout {timeout}") connect("example.com", port=8080, timeout=10)
In this example, timeout
must be passed as a keyword argument.
Common Pitfalls
1. Mutable Default Values
Using mutable types (like lists or dictionaries) as default values can lead to unexpected behavior:
def add_item(item, items=[]): items.append(item) return items print(add_item("apple")) # ['apple'] print(add_item("banana")) # ['apple', 'banana'] — not what you might expect!
Solution: Use None
as the default and initialize inside the function.
def add_item(item, items=None): if items is None: items = [] items.append(item) return items
When to Use Optional Arguments
Optional arguments are useful when:
- You want to provide a sensible default for most cases.
- You want to allow customization without requiring verbose calls.
- You’re designing libraries or APIs with flexible interfaces.
Conclusion
Optional arguments are a cornerstone of Python's flexibility. By mastering default values, *args
, **kwargs
, and keyword-only arguments, you can design more intuitive and robust functions. Just be cautious with mutable default values, and always consider the readability of your function signatures.
FAQs
Parameters with default values that callers can omit when calling the function.
Because they retain state across function calls, leading to unintended behavior.
By placing them after a *
in the function definition.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ