Understanding Maximum Integer Values in Go
James Reed
Infrastructure Engineer · Leapcell
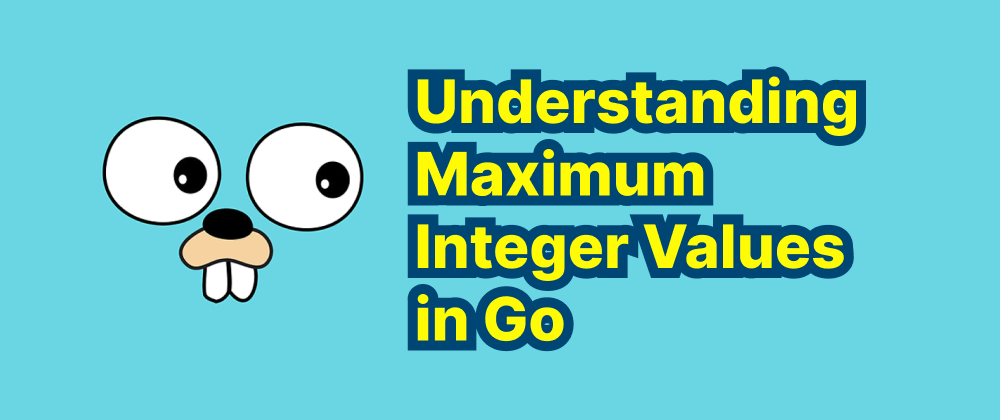
Key Takeaways
- The maximum value of
int
in Go depends on the system architecture (32-bit or 64-bit). - Integer overflow in Go does not panic but wraps around using modulo arithmetic.
- Choosing the right integer type is crucial for avoiding overflow issues.
In programming, understanding the limits of data types is crucial for writing robust and error-free code. In Go, the int
type is commonly used to represent integer values. However, the maximum value that an int
can hold isn't fixed and depends on the architecture of the system on which the Go program is running. This article explores the maximum integer values in Go and how to handle them effectively.
Integer Sizes in Go
Go defines several integer types with varying sizes:
int8
: 8-bit signed integerint16
: 16-bit signed integerint32
: 32-bit signed integerint64
: 64-bit signed integeruint8
: 8-bit unsigned integeruint16
: 16-bit unsigned integeruint32
: 32-bit unsigned integeruint64
: 64-bit unsigned integer
The int
and uint
types are platform-dependent:
- On a 32-bit system,
int
anduint
are 32 bits wide. - On a 64-bit system,
int
anduint
are 64 bits wide.
Maximum Values of Integer Types
Each integer type has a specific range of values it can represent:
int8
: -128 to 127int16
: -32,768 to 32,767int32
: -2,147,483,648 to 2,147,483,647int64
: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807uint8
: 0 to 255uint16
: 0 to 65,535uint32
: 0 to 4,294,967,295uint64
: 0 to 18,446,744,073,709,551,615
For the int
type:
- On a 32-bit system: -2,147,483,648 to 2,147,483,647
- On a 64-bit system: -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
Determining the Maximum Value of int
To programmatically determine the maximum value of the int
type in Go, you can use the math
package:
package main import ( "fmt" "math" ) func main() { fmt.Println("Max int value:", math.MaxInt) }
The math.MaxInt
constant provides the maximum value of the int
type for the current platform.
Handling Integer Overflow
Integer overflow occurs when an operation results in a value that exceeds the maximum value the integer type can hold. Go does not panic on integer overflow; instead, it wraps around using modulo arithmetic. For example:
package main import "fmt" func main() { var a int8 = 127 a++ fmt.Println(a) // Output: -128 }
In this example, incrementing the maximum int8
value (127
) results in -128
due to overflow.
Best Practices
- Choose Appropriate Integer Types: Use integer types that suit the range of values you expect. For example, use
int32
orint64
if you anticipate large numbers. - Check for Overflow: Implement checks to detect potential overflows, especially when performing arithmetic operations.
- Use Unsigned Types Cautiously: Unsigned integers (
uint
) can represent larger positive values but can lead to unexpected behavior if not handled carefully, especially when subtracting or comparing with signed integers.
Understanding the maximum values of integer types in Go is essential for writing reliable and efficient programs. By selecting appropriate types and implementing proper checks, you can prevent and handle integer overflows effectively.
FAQs
Use math.MaxInt
, which provides the platform-specific maximum int
value.
Go wraps the value around using modulo arithmetic instead of causing an error.
Not necessarily; choose an integer type based on expected value ranges and performance needs.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ