Building an Efficient Web Scraper in Golang
Daniel Hayes
Full-Stack Engineer · Leapcell
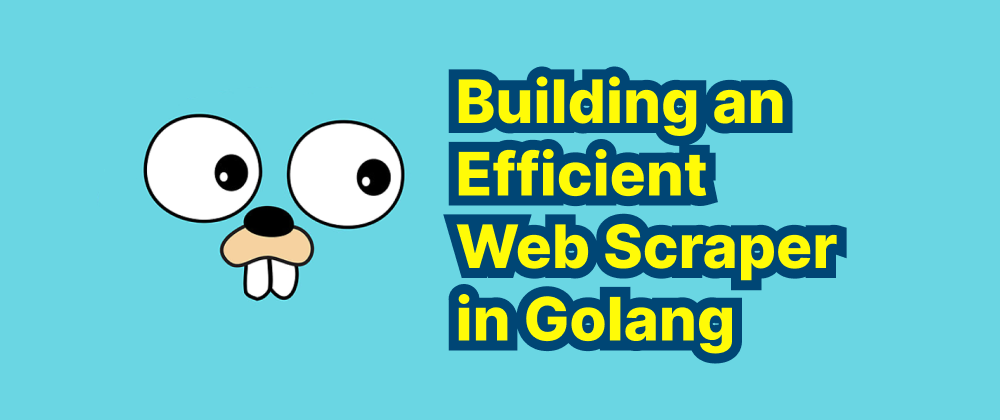
Key Takeaways
- Colly is a Powerful Tool: Colly simplifies web scraping in Go with its clean API and robust features.
- Concurrency Enhances Efficiency: Using asynchronous scraping and concurrency settings improves data extraction speed.
- Respect Website Policies: Adhering to
robots.txt
and implementing rate limiting prevents potential issues like IP bans.
Web scraping is the automated process of extracting information from websites. It is widely used for data mining, research, and monitoring purposes. Golang, known for its efficiency and concurrency capabilities, is an excellent choice for building web scrapers.
Setting Up the Go Environment
Before diving into coding, ensure that you have Go installed on your system. You can download it from the official Go website. After installation, verify it by running:
go version
This command should display the installed Go version.
Next, set up your Go workspace and initialize a new module:
mkdir go-web-scraper cd go-web-scraper go mod init web-scraper
This sequence creates a new directory, navigates into it, and initializes a Go module named web-scraper
.
Choosing a Web Scraping Library
Golang offers several libraries for web scraping. One of the most popular and efficient is Colly, which provides a clean API for scraping tasks. Install Colly using:
go get github.com/gocolly/colly
This command adds Colly to your project's dependencies.
Building the Web Scraper
Create a file named main.go
in your project directory and start by setting up the basic structure:
package main import ( "fmt" "github.com/gocolly/colly" ) func main() { // Initialize the collector c := colly.NewCollector() // Define the scraping logic c.OnHTML("element-selector", func(e *colly.HTMLElement) { // Extract data data := e.Text fmt.Println(data) }) // Start the scraping process c.Visit("https://example.com") }
In this template:
colly.NewCollector()
initializes a new collector.c.OnHTML
specifies the HTML elements to target using CSS selectors.e.Text
retrieves the text content of the selected element.c.Visit
begins the scraping process by visiting the specified URL.
Replace "element-selector"
with the actual CSS selector of the data you wish to extract, and "https://example.com"
with your target URL.
Handling Requests and Responses
Colly allows you to manage various events during the scraping process:
- OnRequest: Triggered before making an HTTP request.
- OnResponse: Triggered after receiving a response.
- OnError: Triggered upon encountering an error.
For example:
c.OnRequest(func(r *colly.Request) { fmt.Println("Visiting", r.URL) }) c.OnResponse(func(r *colly.Response) { fmt.Println("Received", r.StatusCode) }) c.OnError(func(r *colly.Response, err error) { fmt.Println("Error:", err) })
These handlers provide insights into the scraping workflow and assist in debugging.
Extracting Specific Data
To extract specific data, inspect the target website to identify the HTML structure. For instance, to scrape article titles from a blog:
c.OnHTML("h2.article-title", func(e *colly.HTMLElement) { title := e.Text fmt.Println("Article Title:", title) })
Here, h2.article-title
is the CSS selector for the article titles.
Managing Concurrency
Colly supports concurrent scraping, which speeds up the data extraction process:
c := colly.NewCollector( colly.Async(true), ) c.Limit(&colly.LimitRule{ DomainGlob: "*", Parallelism: 2, Delay: 5 * time.Second, })
This configuration sets the scraper to work asynchronously with a maximum of two concurrent requests and a 5-second delay between them.
Respecting robots.txt
and Rate Limiting
It's essential to respect the robots.txt
file of websites and implement rate limiting to avoid overloading servers:
c := colly.NewCollector( colly.Async(true), colly.UserAgent("YourUserAgent"), colly.AllowURLRevisit(), ) c.Limit(&colly.LimitRule{ DomainGlob: "*", Parallelism: 1, Delay: 2 * time.Second, })
This setup ensures compliance with the website's crawling policies and prevents potential IP bans.
Conclusion
Building a web scraper in Golang using Colly is both efficient and straightforward. By following best practices such as respecting robots.txt
and implementing rate limiting, you can create robust scrapers that responsibly extract data from websites.
FAQs
Colly offers an efficient, user-friendly API for scraping and supports advanced features like concurrency and custom request handling.
By enabling Colly's async mode and configuring parallelism and delay rules to control request rates.
Configure Colly to respect robots.txt
and use rate limiting to avoid overloading servers.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ