Python Module Management: A Practical Guide
Daniel Hayes
Full-Stack Engineer · Leapcell
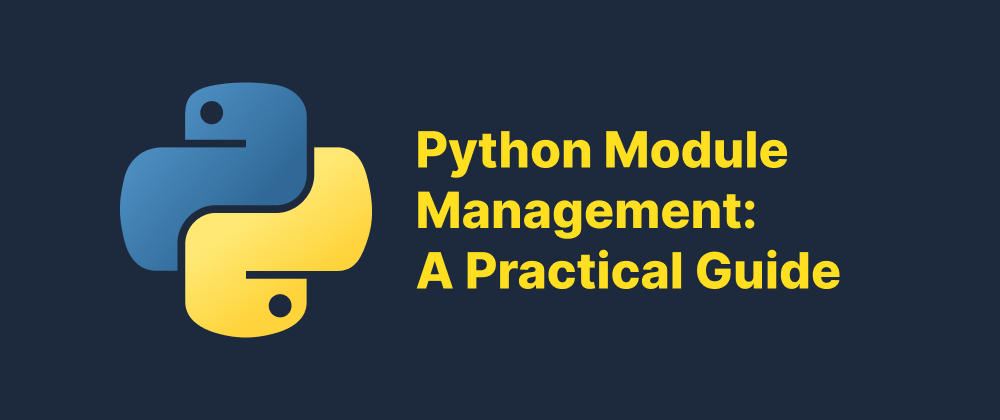
Key Takeaways
- Use virtual environments to isolate project dependencies.
- Manage packages efficiently with pip, Poetry, or Pipenv.
- Organize code into logical modules and packages for maintainability.
Managing modules effectively is an essential skill for any Python developer. As Python projects grow in complexity, proper module and dependency management ensures maintainability, collaboration, and deployment success. This article explores the common practices and tools used for managing Python modules and dependencies.
What Is a Python Module?
A Python module is simply a .py
file containing Python definitions and statements. Modules allow developers to organize their code logically, separating functionalities into reusable and manageable components. A collection of modules can be grouped into a package, which is a directory with an __init__.py
file.
For example:
# math_utils.py def add(x, y): return x + y
This file can be imported and used in other scripts:
import math_utils result = math_utils.add(3, 5)
Installing External Modules
To use third-party modules, Python developers typically rely on PyPI, the Python Package Index. The standard tool for installing packages from PyPI is pip
.
pip install requests
You can then use the module in your script:
import requests response = requests.get("https://example.com")
Virtual Environments
One of the best practices in Python development is using virtual environments to isolate project dependencies. This prevents conflicts between packages required by different projects.
To create and activate a virtual environment:
python -m venv venv source venv/bin/activate # On Windows: venv\Scripts\activate
Now any pip install
command installs packages only inside this environment.
Managing Dependencies
To keep track of installed packages, you can use pip freeze
:
pip freeze > requirements.txt
This file can be shared with others or used in deployment:
pip install -r requirements.txt
Alternatively, tools like Poetry and Pipenv provide higher-level dependency management by automatically resolving and locking package versions.
Example with Poetry:
poetry init poetry add requests poetry install
Poetry maintains a pyproject.toml
and poetry.lock
file for consistent builds across environments.
Module Structure Best Practices
Here are some tips for organizing Python modules:
- Use meaningful and consistent naming conventions.
- Keep related functions/classes in the same module.
- Avoid circular imports by rethinking module boundaries.
- Group multiple modules into packages for better structure.
A sample project layout:
my_project/
├── main.py
├── utils/
│ ├── __init__.py
│ └── file_ops.py
├── services/
│ ├── __init__.py
│ └── api_handler.py
Conclusion
Proper Python module management is key to writing clean, scalable, and maintainable code. By using virtual environments, dependency tools like pip or Poetry, and following modular design principles, you can keep your Python projects efficient and collaborative. As your projects grow, mastering these tools will save time and reduce technical debt.
FAQs
It isolates dependencies, preventing conflicts between different projects.
pip installs packages manually, while Poetry manages dependencies and lock files automatically.
Group related modules into packages and use clear, consistent naming conventions.
We are Leapcell, your top choice for hosting Python projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ