Understanding Golang JSON Tags
Daniel Hayes
Full-Stack Engineer · Leapcell
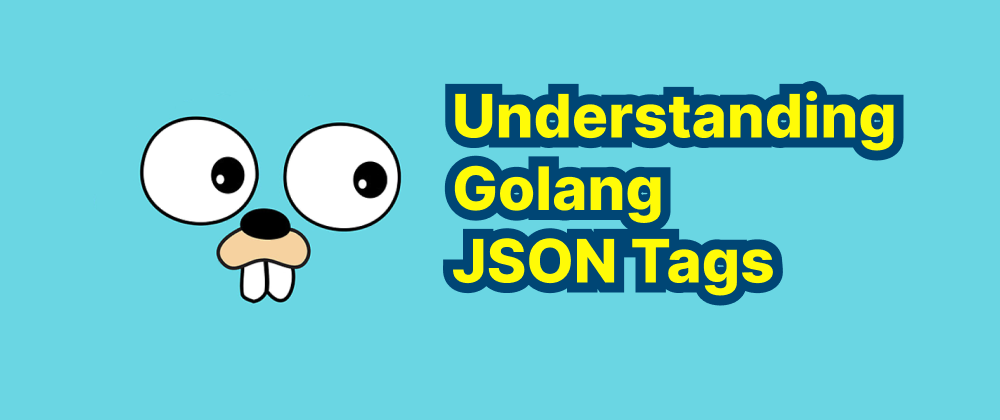
Key Takeaways
- Golang JSON tags control how struct fields are serialized and deserialized.
- Options like
omitempty
and-
modify field inclusion in JSON output. - The
string
option forces numeric and boolean fields to be serialized as strings.
In Go, struct tags play a crucial role in controlling the encoding and decoding of JSON data. By leveraging json
tags, developers can customize how struct fields are mapped to JSON keys, influencing both serialization (marshaling) and deserialization (unmarshaling) processes.
Basic Usage of JSON Tags
Consider the following struct definition:
type Person struct { Name string `json:"name"` Age int `json:"age"` Email string `json:"email,omitempty"` }
Here, the struct tags specify the JSON key names corresponding to each field:
Name
maps to"name"
.Age
maps to"age"
.Email
maps to"email"
but includes theomitempty
option.
The omitempty
option ensures that if the Email
field is empty (i.e., its zero value), it will be omitted from the JSON output.
Ignoring Fields During Serialization
To exclude a struct field from JSON serialization, use the -
tag:
type Person struct { Name string `json:"name"` Age int `json:"age"` Secret string `json:"-"` }
In this example, the Secret
field will be ignored during both marshaling and unmarshaling.
Handling Empty Values with omitempty
The omitempty
tag option prevents fields with zero values from appearing in the JSON output:
type Person struct { Name string `json:"name"` Age int `json:"age,omitempty"` Email *string `json:"email,omitempty"` }
If Age
is 0
or Email
is nil
, these fields will be omitted from the serialized JSON.
Using the string
Tag Option
The string
option tells the encoder to serialize numeric or boolean fields as JSON strings:
type Person struct { ID int `json:"id,string"` Name string `json:"name"` Active bool `json:"active,string"` }
Here, ID
and Active
will be represented as strings in the JSON output.
Multiple Tags and Reflection
Go supports multiple tags for different purposes:
type Person struct { Name string `json:"name" xml:"name"` Age int `json:"age" xml:"age"` }
Using reflection, you can retrieve these tags at runtime:
t := reflect.TypeOf(Person{}) field, _ := t.FieldByName("Name") jsonTag := field.Tag.Get("json") xmlTag := field.Tag.Get("xml")
Conclusion
Understanding and utilizing JSON tags in Go structs allows for precise control over JSON serialization and deserialization, facilitating seamless data interchange between Go applications and other systems.
For more detailed examples and advanced usage, refer to the official Go documentation and community resources.
FAQs
The field name will be used as the default JSON key in camel case.
Yes, you can use multiple struct tags for different encoding formats.
Use json:"-"
to prevent a field from being included in the JSON output.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ