Aspect-Oriented Programming (AOP) in Go
James Reed
Infrastructure Engineer · Leapcell
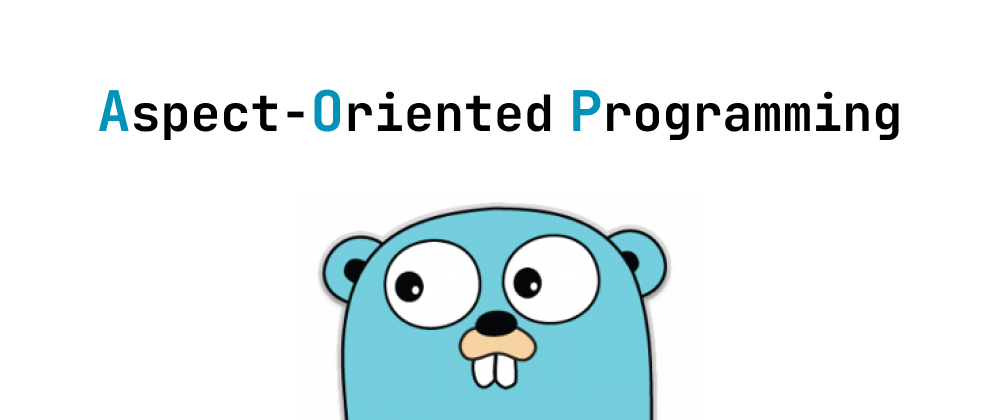
In Java development, AOP (Aspect-Oriented Programming) is a very popular technique. It decouples cross-cutting concerns—such as logging, permission checks, and performance monitoring—from core business logic, making code structure clearer and responsibilities more explicit. Next, we will use the middleware and function-wrapping mechanisms of the Gin framework to demonstrate how to implement this concept and analyze its similarities and differences compared to pure AOP.
Advantages of AOP
In Java applications, the main advantages of AOP include:
- Decoupling business logic from common functionalities: Business code focuses on core functionality, while aspects such as logging, error handling, and performance monitoring are automatically injected by the aspect mechanism.
- Improved code reuse and consistency: Developers only need to define aspect code once, and the framework ensures it takes effect across multiple join points, avoiding repetitive implementation.
- Ease of maintenance and extensibility: When modifying logging strategies or performance monitoring logic, there is no need to adjust business logic in multiple places—only the corresponding aspect code needs updating.
These advantages make systems more modular and easier to maintain, and adopting this idea in Go projects also has practical value.
How Go Implements AOP
Although Go is inherently simple and straightforward without a built-in AOP framework, cross-cutting concerns still exist in some scenarios. If we write logging, monitoring, and error-handling code directly in every business function, it leads to redundancy and high coupling, reducing code maintainability.
With the help of Go's higher-order functions, closures, and the decorator pattern, we can “weave in” logging or monitoring functionality dynamically without modifying the core business logic. For web services, the middleware mechanism in the Gin framework is a perfect embodiment of this idea: during the request processing flow, pre-processing and post-processing are handled via chained function calls.
Implementing AOP-like Effects in Gin
Below are several examples demonstrating how to implement logging—an aspect-like feature—within Gin’s controller layer (i.e., where handler functions reside).
Writing Logs Directly in Handlers
In this approach, each handler must manually insert logging code:
package main import ( "log" "net/http" "github.com/gin-gonic/gin" ) func main() { r := gin.Default() // Route handler with embedded logging logic r.GET("/noaspect", func(c *gin.Context) { // Log request start log.Println("[Log Center] Request started") // Execute business logic c.String(http.StatusOK, "Executing business logic - No aspect implemented") // Log request end log.Println("[Log Center] Request ended") }) r.Run(":8080") }
Disadvantages:
- Every handler must repeat the logging code, leading to redundancy.
- Modifying the logging strategy requires changes in each individual handler, resulting in high maintenance cost.
Using Middleware to Implement “Aspects”
The Gin framework provides a middleware mechanism. We can separate the logging logic into middleware, allowing it to be automatically “woven into” the request flow with pre- and post-processing around the business logic.
package main import ( "log" "net/http" "github.com/gin-gonic/gin" ) // Logger middleware: logs the start and end of each request func Logger() gin.HandlerFunc { return func(c *gin.Context) { // Log before handling the request log.Printf("[Log Center] Request started: %s %s", c.Request.Method, c.Request.URL.Path) // Proceed to the next handler c.Next() // Log after handling the request, such as the status code log.Printf("[Log Center] Request ended: Status code %d", c.Writer.Status()) } } func main() { r := gin.Default() // Register Logger middleware globally r.Use(Logger()) // Define business route r.GET("/ping", func(c *gin.Context) { c.String(http.StatusOK, "pong") }) r.Run(":8080") }
This method handles all requests uniformly, ensuring centralized management of cross-cutting concerns like logging.
Function Wrapping (for Specific Handlers)
If you only want to enhance logging for specific handler methods, you can also use function wrapping, as shown below:
package main import ( "log" "net/http" "github.com/gin-gonic/gin" ) func main() { r := gin.Default() // Regular route without function wrapping r.GET("/noaspect", func(c *gin.Context) { c.String(http.StatusOK, "Executing business logic - No aspect implemented") }) // Route using LogAspect wrapper r.GET("/aspect", LogAspect(BusinessController)) r.Run(":8080") } // BusinessController is the actual business handler, focusing only on core logic func BusinessController(c *gin.Context) { c.String(http.StatusOK, "Executing business logic") } // LogAspect wraps business handlers with logging logic before and after execution func LogAspect(handler gin.HandlerFunc) gin.HandlerFunc { return func(c *gin.Context) { // Pre-processing: log request start log.Println("[Log Center] Operation started") // Call business logic handler(c) // Post-processing: log request end log.Println("[Log Center] Operation ended") } }
Advantages:
- Decoupling: Business handlers don’t need to worry about logging, allowing a clear separation between core logic and cross-cutting concerns.
- Reusability: The same
LogAspect
wrapper can be applied to multiple handlers for centralized management. - Ease of maintenance: Modifying the logging logic only requires updating the wrapper or middleware, without touching individual business handlers.
Differences and Similarities Between Middleware and AOP
Essentially, Gin middleware is a type of decorator pattern. In each request handling flow (Handler Chain), the middleware:
- Executes pre-processing before the request enters the business handler (e.g., logging, permission checks, setting context variables).
- Then invokes the next handler or the final business logic.
- After the request is processed, it performs post-processing, such as logging the response or collecting metrics.
This flow perfectly matches the “before-execute-after” pattern, making it ideal for unified logging or request monitoring.
Although Gin middleware and function wrappers implement logic similar to AOP, there are still some key differences:
-
Implementation Mechanism:
- AOP usually relies on framework support, using techniques like dynamic proxies or bytecode weaving to inject additional logic without modifying business code.
- Middleware and function wrappers use closures and the decorator pattern, manually constructing the call chain at the code level, offering more transparency.
-
Scope of Application:
- AOP can operate at a fine-grained method level and can even inject logic at arbitrary points inside methods.
- Middleware typically applies to the entire lifecycle of an HTTP request, while function wrapping mainly enhances the beginning and end of a handler function.
-
Coupling:
- AOP automatically weaves in logic, reducing repetitive code, but sometimes makes debugging or tracing less intuitive.
- Middleware and wrappers have explicit call chains, which are easier to understand, but require developers to manually construct wrapping logic.
Regardless of the approach, the core idea is to adopt the design of cross-cutting concerns, separating generic behavior from business logic, thereby improving code modularity and maintainability.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ