Understanding Panic in Go: Causes, Recovery, and Best Practices
Daniel Hayes
Full-Stack Engineer · Leapcell
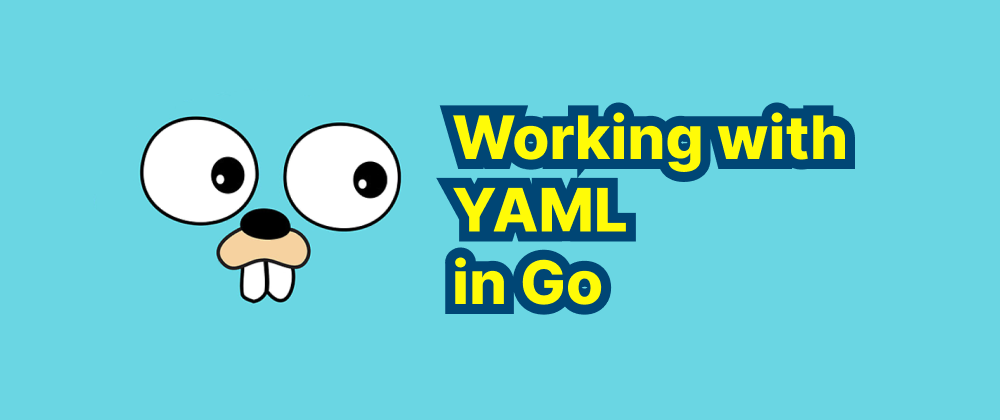
Key Takeaways
- Panic signifies a critical runtime error that disrupts normal program execution.
- Recover allows controlled handling of panics to prevent abrupt termination.
- Panics should be used sparingly, with explicit error handling preferred for expected issues.
In Go programming, a panic signifies a critical runtime error that disrupts the normal execution flow. Distinct from conventional error handling, which anticipates and manages expected issues, a panic addresses unforeseen errors that typically lead to the termination of the program. This article delves into the concept of panic in Go, its appropriate usage, and strategies for recovery.
What Is a Panic?
A panic in Go is an event that halts the normal execution of a program. It can be initiated explicitly by the programmer using the panic
function or occur implicitly due to runtime errors, such as out-of-bounds array accesses or nil pointer dereferences. When a panic occurs, the program begins unwinding the stack, executing any deferred functions, and ultimately terminates if the panic is not recovered.
When to Use Panic
Panics are generally reserved for situations where the program encounters an unrecoverable state. Common scenarios include:
- Invalid Operations: Performing operations that are inherently erroneous, such as dividing by zero or accessing invalid memory.
- Inconsistent States: Detecting a state in the program that should be impossible according to the program's logic, indicating a bug.
- Critical Failures: Encountering conditions where continuing execution could lead to data corruption or other severe issues.
It's important to note that Go encourages explicit error handling using the error
type for expected errors, reserving panics for truly exceptional circumstances.
Example: Triggering a Panic
Consider a function that divides two integers:
package main import "fmt" func divide(a, b int) int { if b == 0 { panic("division by zero") } return a / b } func main() { fmt.Println(divide(10, 0)) fmt.Println("This line will not be executed") }
In this example, calling divide(10, 0)
triggers a panic due to an attempt to divide by zero. Consequently, the program terminates, and the subsequent line is not executed.
Recovering from a Panic
Go provides a mechanism to regain control of a panicking goroutine using the recover
function. When invoked within a deferred function, recover
captures the panic and allows the program to continue executing.
Here's an example demonstrating recovery from a panic:
package main import "fmt" func divide(a, b int) int { if b == 0 { panic("division by zero") } return a / b } func main() { defer func() { if r := recover(); r != nil { fmt.Println("Recovered from panic:", r) } }() fmt.Println(divide(10, 0)) fmt.Println("This line will be executed") }
In this case, the deferred function captures the panic, prints a recovery message, and allows the program to proceed, resulting in the execution of the final print statement.
Best Practices
- Use Panics Sparingly: Reserve panics for truly exceptional conditions that are unrecoverable and indicate a serious flaw in the program.
- Prefer Explicit Error Handling: For anticipated errors, utilize Go's built-in error handling mechanisms, returning error values and checking them explicitly.
- Ensure Clean-Up with Deferred Functions: Leverage deferred functions to release resources or perform necessary clean-up tasks, even in the event of a panic.
By adhering to these practices, developers can write robust Go programs that handle errors gracefully and maintain stability.
FAQs
Use panic only for unrecoverable errors, such as logical inconsistencies or fatal failures.
When used inside a deferred function, recover
captures a panic and prevents program termination.
No, Go does not recover automatically. A developer must use recover
explicitly to regain control.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ