Working with YAML in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
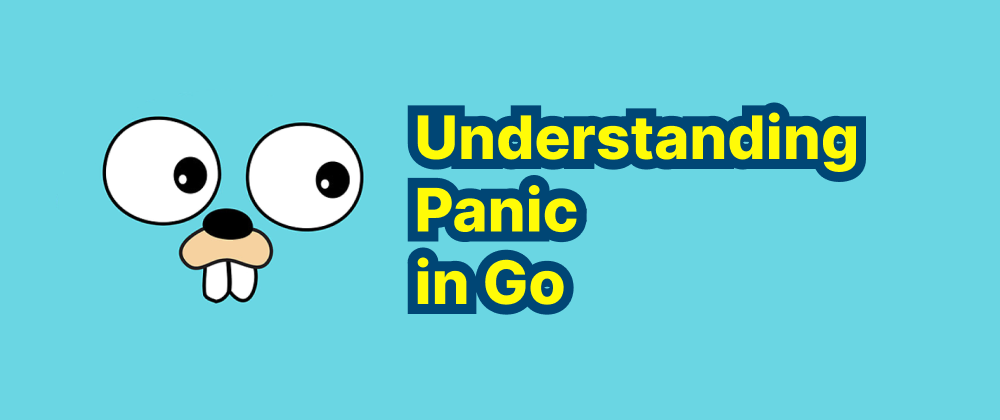
Key Takeaways
- Go provides multiple libraries, such as
go-yaml/yaml
andgoccy/go-yaml
, for parsing and generating YAML. - YAML can be unmarshaled into Go structs and marshaled back into YAML format.
- Choosing the right YAML library depends on feature support and performance needs.
YAML (YAML Ain't Markup Language) is a human-readable data serialization standard commonly used for configuration files and data exchange between languages with different data structures. In the Go programming language, there are several libraries available to parse and generate YAML content. This article explores the primary libraries and demonstrates how to work with YAML in Go.
YAML Libraries in Go
go-yaml/yaml
The go-yaml/yaml
package is one of the most widely used libraries for handling YAML in Go. Developed as a pure Go port of the libyaml C library, it allows Go programs to encode and decode YAML values comfortably. The package supports most of YAML 1.2 and preserves some behavior from YAML 1.1 for backward compatibility. [Source: https://github.com/go-yaml/yaml]
To install the package, run:
go get gopkg.in/yaml.v3
goccy/go-yaml
An alternative to go-yaml/yaml
is the goccy/go-yaml
library. Developed from scratch to replace go-yaml/yaml
, this library addresses some of the limitations of the former, such as maintenance concerns and parsing capabilities. It offers features like better parsing, support for YAML anchors and aliases, and customizable marshal/unmarshal behavior. [Source: https://github.com/goccy/go-yaml]
To install this package, run:
go get github.com/goccy/go-yaml
Parsing YAML in Go
To parse a YAML file in Go, you can define a struct that mirrors the structure of your YAML content and use the Unmarshal
function to decode the YAML data into the struct.
Here's an example:
package main import ( "fmt" "log" "gopkg.in/yaml.v3" ) var data = ` a: Easy! b: c: 2 d: [3, 4] ` type T struct { A string B struct { RenamedC int `yaml:"c"` D []int `yaml:",flow"` } } func main() { t := T{} err := yaml.Unmarshal([]byte(data), &t) if err != nil { log.Fatalf("error: %v", err) } fmt.Printf("--- t:\n%v\n\n", t) }
In this example, the YAML content is unmarshaled into the struct T
, and the values are printed accordingly. [Source: https://pkg.go.dev/gopkg.in/yaml.v3]
Generating YAML in Go
To generate YAML from Go data structures, you can use the Marshal
function provided by the YAML libraries. Here's how you can do it:
package main import ( "fmt" "log" "gopkg.in/yaml.v3" ) type T struct { A string B struct { RenamedC int `yaml:"c"` D []int `yaml:",flow"` } } func main() { t := T{ A: "Easy!", B: struct { RenamedC int `yaml:"c"` D []int `yaml:",flow"` }{ RenamedC: 2, D: []int{3, 4}, }, } d, err := yaml.Marshal(&t) if err != nil { log.Fatalf("error: %v", err) } fmt.Printf("--- t dump:\n%s\n\n", string(d)) }
This code defines a struct T
, populates it with data, and marshals it into YAML format. The resulting YAML content is then printed. [Source: https://pkg.go.dev/gopkg.in/yaml.v3]
Conclusion
Working with YAML in Go is straightforward, thanks to robust libraries like go-yaml/yaml
and goccy/go-yaml
. Depending on your project's requirements, you can choose the library that best fits your needs. By understanding how to parse and generate YAML, you can effectively manage configuration files and facilitate data exchange in your Go applications.
FAQs
go-yaml/yaml
is widely used, but goccy/go-yaml
offers alternative features and performance improvements.
Use the Unmarshal
function from a YAML library to decode YAML into Go struct fields.
Yes, using the Marshal
function, Go structs can be serialized into YAML.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ