How to Read JSON in Python
Grace Collins
Solutions Engineer · Leapcell
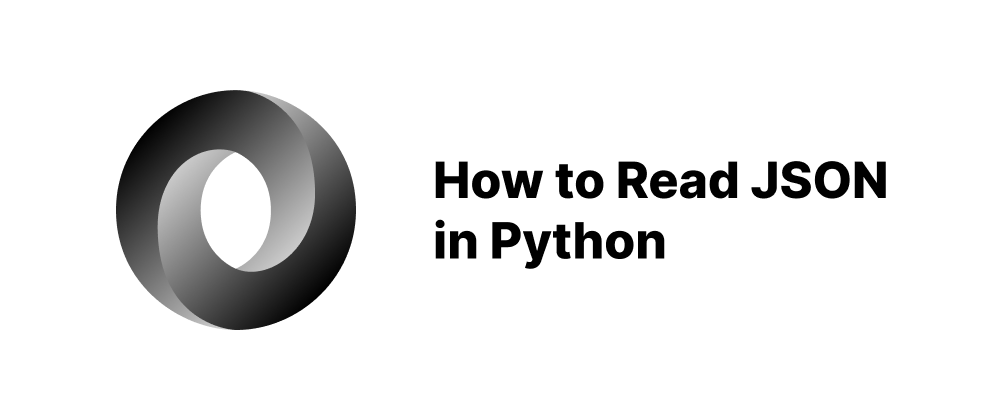
Key Takeaways
- Use
json.loads()
to parse JSON strings into Python objects. - Use
json.load()
to read JSON data from a file. - Always handle exceptions like
JSONDecodeError
andFileNotFoundError
.
JSON (JavaScript Object Notation) is a popular data format used for storing and exchanging data. Python provides built-in support for JSON through the json
module, making it easy to work with JSON data in your programs. In this article, we’ll explore how to read JSON from strings and files using Python.
What is JSON?
JSON is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It’s commonly used in web APIs and configuration files.
A typical JSON object looks like this:
{ "name": "Alice", "age": 30, "is_student": false, "courses": ["Math", "Science"] }
Reading JSON Strings
Python’s json.loads()
method can parse a JSON-formatted string and convert it into a Python dictionary:
import json json_string = '{"name": "Alice", "age": 30, "is_student": false, "courses": ["Math", "Science"]}' data = json.loads(json_string) print(data["name"]) # Output: Alice
Reading JSON from a File
To read a JSON file, use the json.load()
method. This method reads from a file object and parses the JSON data:
import json with open("data.json", "r") as file: data = json.load(file) print(data["courses"]) # Output: ['Math', 'Science']
Notes:
- Use the
with
statement to open files, as it ensures that the file is properly closed after reading. json.load()
should not be confused withjson.loads()
. The former reads from a file object, while the latter reads from a string.
Handling Errors
When working with JSON, you should always anticipate possible parsing errors:
import json try: with open("data.json", "r") as file: data = json.load(file) except json.JSONDecodeError as e: print("Failed to decode JSON:", e) except FileNotFoundError: print("The file was not found.")
Conclusion
Reading JSON in Python is straightforward thanks to the json
module. Whether you’re working with a string or a file, Python makes it easy to parse and work with JSON data structures. This is particularly useful when dealing with APIs, configuration files, or data exchange between systems.
FAQs
json.load()
reads from a file object; json.loads()
parses a JSON string.
Use try-except blocks to handle decoding and file-not-found errors.
Yes, nested JSON is parsed into nested Python dictionaries and lists.
We are Leapcell, your top choice for hosting backend projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ