How to Use `time.Sleep` in Go
Daniel Hayes
Full-Stack Engineer · Leapcell
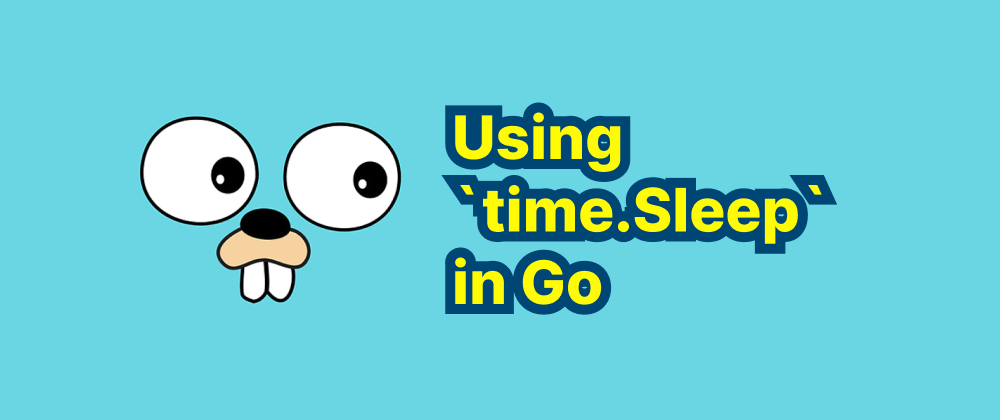
Key Takeaways
time.Sleep
pauses the current goroutine for a specified duration.- It uses predefined time constants like
time.Second
andtime.Millisecond
. - It only affects the calling goroutine, not the entire program.
In Go programming, managing the timing of code execution is essential for tasks like rate limiting, scheduling, and simulating delays. The time
package provides the Sleep
function to pause the execution of the current goroutine for a specified duration.
Importing the time
Package
To use time.Sleep
, you must first import the time
package:
import "time"
Syntax of time.Sleep
The Sleep
function has the following syntax:
func Sleep(d Duration)
The parameter d
represents the duration of the sleep interval. The Duration
type is a type alias for int64
and represents the elapsed time in nanoseconds.
Specifying Duration
Go offers predefined constants in the time
package to specify durations:
time.Nanosecond
(ns)time.Microsecond
(µs)time.Millisecond
(ms)time.Second
(s)time.Minute
(m)time.Hour
(h)
You can use these constants to set the desired sleep duration. For example, to sleep for 2 seconds:
time.Sleep(2 * time.Second)
Example: Pausing Execution
Here's a simple example demonstrating the use of time.Sleep
:
package main import ( "fmt" "time" ) func main() { fmt.Println("Start") time.Sleep(3 * time.Second) fmt.Println("End") }
In this program, "Start" is printed, the execution pauses for 3 seconds, and then "End" is printed.
Considerations
-
Goroutine Suspension:
time.Sleep
only pauses the current goroutine, not the entire program. Other goroutines continue to run unaffected. -
Accuracy: The actual sleep duration may be longer than specified due to factors like system scheduling and the accuracy of the system clock.
-
Alternatives: For more advanced timing control, consider using
time.Ticker
ortime.Timer
.
Conclusion
The time.Sleep
function is a straightforward way to introduce delays in Go programs. By specifying the appropriate duration, you can control the timing of code execution effectively.
FAQs
No, it only pauses the current goroutine.
You can use time.Nanosecond
, time.Millisecond
, time.Second
, etc.
No, for more precise control, time.Ticker
or time.Timer
is preferred.
We are Leapcell, your top choice for hosting Go projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ